javascript 配列の中央値を求める
- 作成日 2022.05.01
- 更新日 2022.11.27
- javascript
- javascript
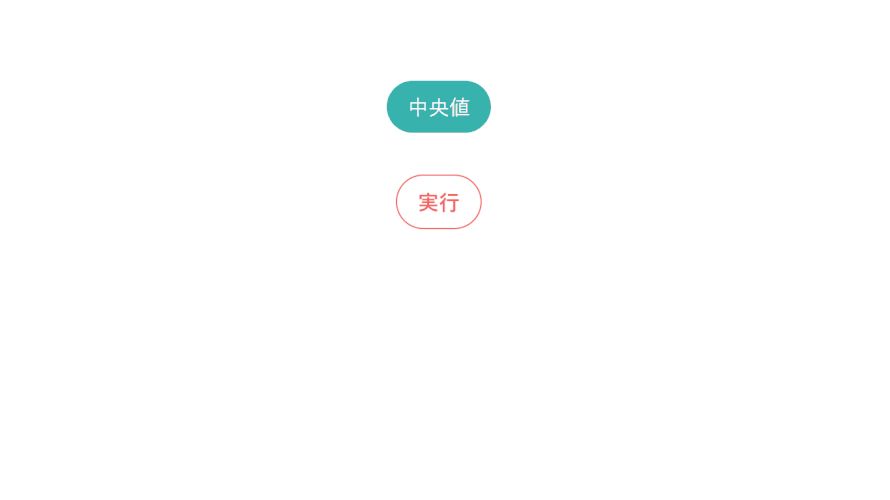
javascriptで、配列の中央値を求めるサンプルコードを記述してます。中央値とは、データを降順に並べて真ん中真ん中にくる値のことです。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 107.0.5304.122
配列の中央値
配列の中央値は、データを小さい順に変換して、真ん中に来る値を取得することで求めることが可能です。配列が偶数の場合は、真ん中2つの平均値となります。
const arr = [1, 1, 2, 4, 5, 8, 9, 10, 11];
// 真ん中の位置を取得
const m = (arr.length / 2) | 0;
// ソート
const arr2 = arr.sort(
function(x, y) {
return x - y;
});
// 結果
if (arr2.length % 2) {
console.log ( arr2[m] ) // 5
}else{
console.log ( arr2[m - 1] + arr2[m] ) / 2
}
サンプルコード
以下は、
「実行」ボタンをクリックすると、ランダムに生成した6個の配列の中央値を計算して表示する
サンプルコードとなります。
※cssには「tailwind」を使用してます。関数はアロー関数を使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8" />
<title>mebeeサンプル</title>
<link
href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css"
rel="stylesheet"
/>
</head>
<script>
const hoge = () => {
const arr = Array(6)
.fill()
.map((x) => ~~(Math.random() * 10));
// 生成した配列を表示
disp(arr, "rand");
// 中央値を表示
result.innerHTML = calc(arr);
};
// 中央値を求める関数
const calc = (n) => {
const m = (n.length / 2) | 0;
const arr = n.sort((x, y) => { return x - y });
console.log( m + " " + arr[m] )
if (arr.length % 2) {
return arr[m]
} else {
return (arr[m - 1] + arr[m]) / 2
}
};
//フロントに表示する関数
const disp = (arr, id) => {
let text = [];
// 配列を利用してforEach文を作成
arr.forEach((x, i) =>
text.push('<li class="list-group-item">' + arr[i] + "</li>")
);
//innerHTMLを使用して表示
document.getElementById(id).innerHTML = text.join("");
};
window.onload = () => {
// クリックイベントを登録
btn.onclick = () => {
hoge();
}; // document.getElementById('btn');を省略
};
</script>
<body>
<div class="container mx-auto my-56 w-56 px-4">
<div class="flex justify-center">
<p
id="result"
class="bg-teal-500 text-white py-2 px-4 rounded-full mb-3 mt-4"
>
中央値
</p>
</div>
<div class="flex justify-center">
<ul id="rand"></ul>
</div>
<div class="flex justify-center">
<button
id="btn"
type="button"
class="mt-5 bg-transparent border border-red-500 hover:border-red-300 text-red-500 hover:text-red-300 font-bold py-2 px-4 rounded-full"
>
実行
</button>
</div>
</div>
</body>
</html>
配列の中央値が計算されていることが確認できます。
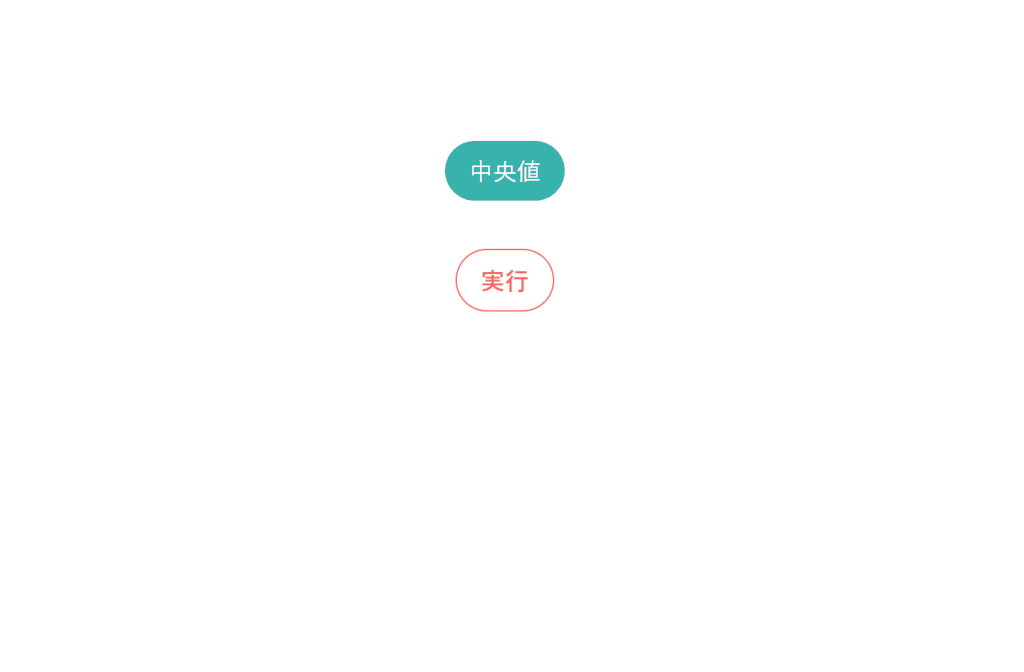
-
前の記事
MySQL DBにあるテーブルごとのレコード数を取得する 2022.04.30
-
次の記事
jquery 指定した要素以前の同じ階層の兄弟要素を範囲を指定して取得する 2022.05.01
コメントを書く