React.js ライブラリ「react-table-scrollbar」を使ってテーブル内でスクロールを実装する
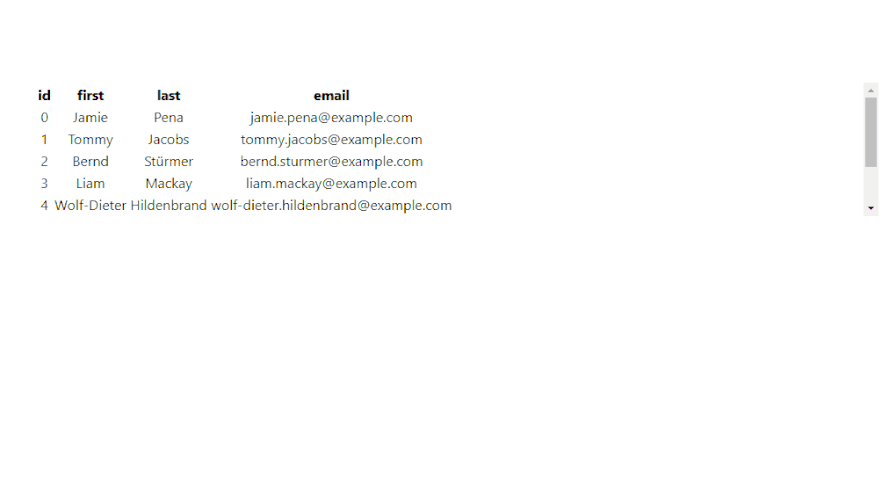
ライブラリ「react-table-scrollbar」をインストールすると、テーブル内でスクロールを実装することが可能です。ここでは、react.jsでreact-table-scrollbarを利用するための手順と簡単な使い方を記述してます。
環境
- OS CentOS Linux release 8.0.1905 (Core)
- node V12.16.3
- npm 6.14.7
- React 16.13.0
react.js環境構築
下記のコマンドで構築してます。ここでは、react-appという名前でプロジェクトを作成してます。
create-react-app react-app
react-table-scrollbarインストール
作成したプロジェクトに移動して、インストールします。
## 作成したプロジェクトに移動
cd react-app
## インストール
npm install react-table-scrollbar
react-table-scrollbar使い方
srcディレクトリにsample.jsと名前で下記のコードを記述します。
import React from 'react'
import TableScrollbar from 'react-table-scrollbar';
const Sample = () => {
const randomusers = [
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Jamie",
"last": "Pena"
},
"location": {
"street": {
"number": 3403,
"name": "Photinia Ave"
},
"city": "Albury",
"state": "Western Australia",
"country": "Australia",
"postcode": 6035,
"coordinates": {
"latitude": "-84.0501",
"longitude": "-160.4075"
},
"timezone": {
"offset": "-5:00",
"description": "Eastern Time (US & Canada), Bogota, Lima"
}
},
"email": "jamie.pena@example.com",
"phone": "08-6765-4381"
},
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Tommy",
"last": "Jacobs"
},
"location": {
"street": {
"number": 7820,
"name": "Mill Lane"
},
"city": "Tipperary",
"state": "Fingal",
"country": "Ireland",
"postcode": 55813,
"coordinates": {
"latitude": "22.0553",
"longitude": "-144.0220"
},
"timezone": {
"offset": "-5:00",
"description": "Eastern Time (US & Canada), Bogota, Lima"
}
},
"email": "tommy.jacobs@example.com",
"phone": "051-790-8740"
},
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Bernd",
"last": "Stürmer"
},
"location": {
"street": {
"number": 5306,
"name": "Waldweg"
},
"city": "Michelstadt",
"state": "Schleswig-Holstein",
"country": "Germany",
"postcode": 30150,
"coordinates": {
"latitude": "-37.7769",
"longitude": "-96.4769"
},
"timezone": {
"offset": "+5:45",
"description": "Kathmandu"
}
},
"email": "bernd.sturmer@example.com",
"phone": "0281-6378065"
},
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Liam",
"last": "Mackay"
},
"location": {
"street": {
"number": 4101,
"name": "Vimy St"
},
"city": "Lasalle",
"state": "Manitoba",
"country": "Canada",
"postcode": "K1F 1K6",
"coordinates": {
"latitude": "-65.8912",
"longitude": "-151.9498"
},
"timezone": {
"offset": "+10:00",
"description": "Eastern Australia, Guam, Vladivostok"
}
},
"email": "liam.mackay@example.com",
"phone": "720-689-4408"
},
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Wolf-Dieter",
"last": "Hildenbrand"
},
"location": {
"street": {
"number": 4388,
"name": "Im Winkel"
},
"city": "Zierenberg",
"state": "Bayern",
"country": "Germany",
"postcode": 47197,
"coordinates": {
"latitude": "-39.1975",
"longitude": "-73.4941"
},
"timezone": {
"offset": "+11:00",
"description": "Magadan, Solomon Islands, New Caledonia"
}
},
"email": "wolf-dieter.hildenbrand@example.com",
"phone": "0578-1952888"
},
{
"gender": "female",
"name": {
"title": "Ms",
"first": "Kristina",
"last": "Williamson"
},
"location": {
"street": {
"number": 9766,
"name": "Edwards Rd"
},
"city": "Darwin",
"state": "Victoria",
"country": "Australia",
"postcode": 4926,
"coordinates": {
"latitude": "-79.5492",
"longitude": "59.7930"
},
"timezone": {
"offset": "-11:00",
"description": "Midway Island, Samoa"
}
},
"email": "kristina.williamson@example.com",
"phone": "06-9642-4718"
},
{
"gender": "female",
"name": {
"title": "Mrs",
"first": "Anna",
"last": "Curtis"
},
"location": {
"street": {
"number": 2637,
"name": "Fairview St"
},
"city": "Bowral",
"state": "South Australia",
"country": "Australia",
"postcode": 5873,
"coordinates": {
"latitude": "-83.9958",
"longitude": "88.4588"
},
"timezone": {
"offset": "-4:00",
"description": "Atlantic Time (Canada), Caracas, La Paz"
}
},
"email": "anna.curtis@example.com",
"phone": "06-7490-3951"
},
{
"gender": "female",
"name": {
"title": "Mrs",
"first": "Luzia",
"last": "Castro"
},
"location": {
"street": {
"number": 2400,
"name": "Travessa dos Açorianos"
},
"city": "São José do Rio Preto",
"state": "Piauí",
"country": "Brazil",
"postcode": 71931,
"coordinates": {
"latitude": "-72.6418",
"longitude": "43.8589"
},
"timezone": {
"offset": "-4:00",
"description": "Atlantic Time (Canada), Caracas, La Paz"
}
},
"email": "luzia.castro@example.com",
"phone": "(14) 7199-7452"
},
];
return (
<div>
<TableScrollbar rows={5}>
<table>
<thead>
<tr>
<th>id</th>
<th>first</th>
<th>last</th>
<th>email</th>
</tr>
</thead>
<tbody>
{randomusers.map((user, id) =>
<tr key={id}>
<td>{id}</td>
<td>{user.name.first}</td>
<td>{user.name.last}</td>
<td>{user.email}</td>
</tr>
)}
</tbody>
</table>
</TableScrollbar>
</div>
);
}
export default Sample;
次に、srcディレクトリ配下にあるApp.jsを下記のように編集します。
import React from 'react';
import Sample from './sample';
import './App.css';
function App() {
const style = {
width: "50%",
margin: "0 auto",
marginTop: 150,
};
return (
<div className="App">
<div style={style}>
<Sample />
</div>
</div>
);
}
export default App;
実行します。
npm start
ブラウザから http://プライベートIP:3000にアクセスすると、 テーブルがスクロールされて表示されていることが確認できます。
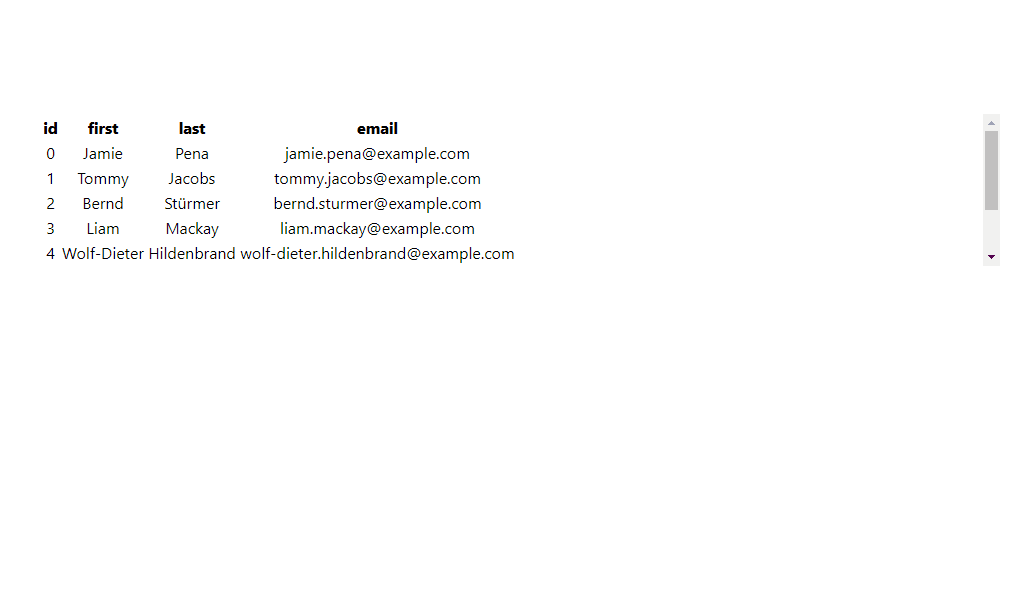
-
前の記事
Python 絶対パスを取得する 2020.11.23
-
次の記事
go言語 べき乗を計算する 2020.11.23
コメントを書く