react.js axiosを使用してapiを取得する
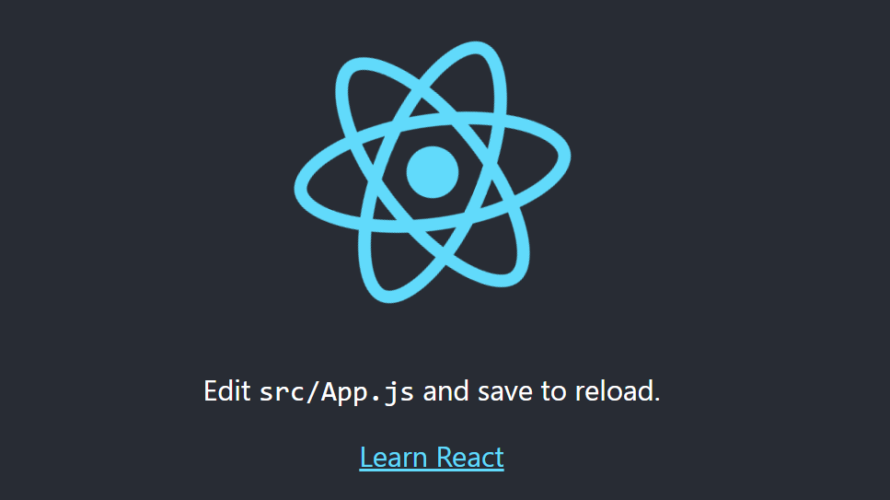
ユーザーが利用しているIPやログイン情報などを、イントラ内にあるソフトが提供してるAPIから取得する際にreact.jsを利用して、apiを取得した際の基本的なソースです。デザインはmaterial-uiを使用してます。
環境
- OS CentOS Linux release 8.0.1905 (Core)
- node V12.13.1
- npm 6.14.2
- React 16.13.0
react.js環境構築
下記のコマンドで構築してます。ここでは、react-appという名前でプロジェクトを作成してます。
create-react-app react-app
axios・material-ui・ material-table インストール
作成したプロジェクトに移動して、今回利用したものをインストールしてます。
## 作成したプロジェクトに移動
cd react-app
## インストール
npm install axios @material-ui/core material-table
axios利用
src配下にGetpcinfo.jsという名前で、下記のコードで記述してます。
取得するjsonは下記のようなものです。
{
"content": [
{
"ipAddresses": [
"192.168.xxx.xxx"
],
"macAddresses": [
"xx-xx-xx-xx-xx-xx"
],
"description": "",
"computerName": "mebee-pc",
"logonUserName": "testuser",
"memory": 3210981398,
"osname": "Windows XP"
}
]
}
API取得の際、認証にBearerが必要なのでheaderに設定します。
import React, { Component } from 'react';
import axios from "axios";
import { Button } from '@material-ui/core';
import MaterialTable from 'material-table';
class Getpcinfo extends Component {
constructor(props) {
super(props);
this.state = {
info: [],
isLoading: true
};
this.getData = this.getData.bind(this);
}
getData() {
axios
.get('http://test.com/api/v1/computers?pageSize=100',{
headers: {
Authorization: "Bearer 8213f5cd-5fds2-4891-83d0-48d172ffab77"
}
})
.then(results => {
const data = results.data.content;
this.setState({
info: data,
isLoading: false
});
});
}
render() {
const columns = [
{ title: 'IP', field: 'ipAddresses' },
{ title: 'user', field: 'logonUserName' },
{ title: 'hostname', field: 'computerName'},
{ title: 'memory', field: 'memory'},
{ title: 'osName', field: 'osName'},
]
return (
<div>
<MaterialTable
title="PC INFO"
columns={columns}
data={this.state.info}
isLoading={this.state.isLoading}
options={{
pageSize: 10,
pageSizeOptions: [10, 20,50, 100, 200, 300, 400 ,500],
toolbar: true,
paging: true
}}
/>
<Button variant="contained" color="primary" onClick={this.getData}>info get</Button>
</div>
)
}
}
export default Getpcinfo
src配下のApp.jsを下記のように編集してます。
import React from 'react';
import Getpcinfo from './Getpcinfo';
import './App.css';
function App() {
const style = {
width: "50%",
margin: "0 auto",
marginTop: 150,
};
return (
<div className="App">
<div style={style}>
<Getpcinfo />
</div>
</div>
);
}
export default App;
アイコンを利用するのでpublic配下のindex.htmlにcssを追加します。
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons" />
起動
起動します。
npm start
ブラウザから http://プライベートIP:3000 にアクセスすると「INFO GET」ボタンをクリックするまでは Loading されますが、ボタンを押すと情報が取得されるようになってます。
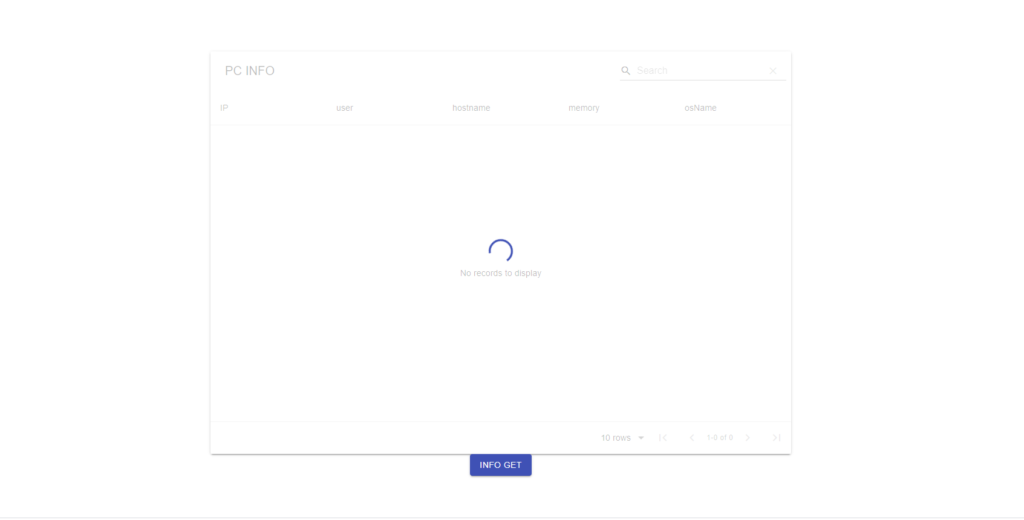
ボタンをクリックすると情報が取得されます。
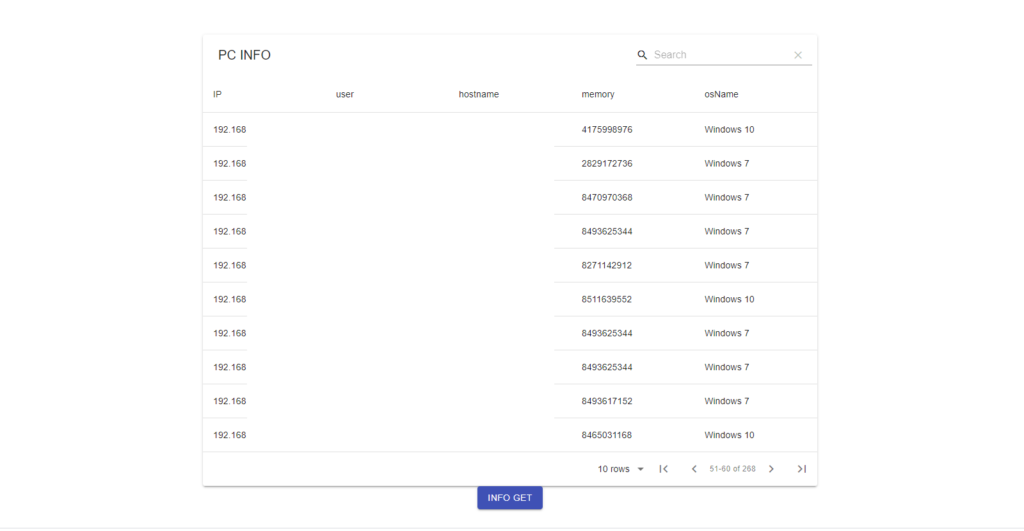
-
前の記事
Windows10 OrientDBをインストールする 2020.05.10
-
次の記事
Nuxt.js ライブラリ「trading-vue-js」をインストールしてJSONツリービューアを実装する 2020.05.10
コメントを書く