javascript 文字列内で一番使用されている文字の文字数を抽出する
- 作成日 2021.06.18
- 更新日 2022.09.07
- javascript
- javascript
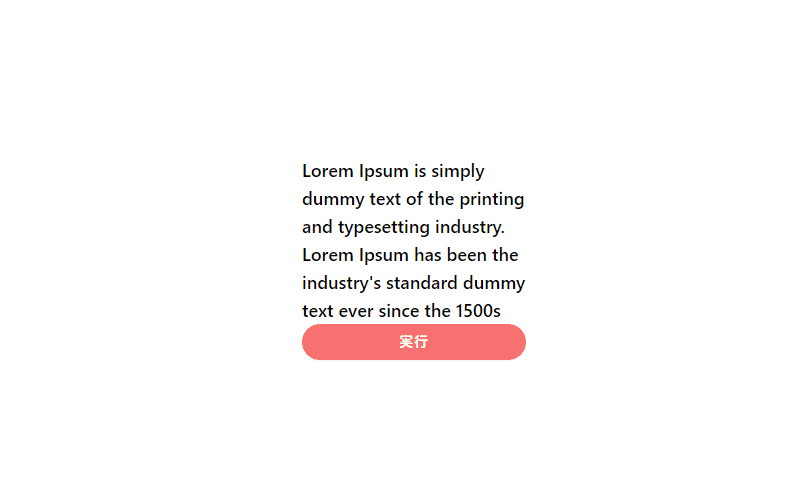
javascriptで、文字列内で一番使用されている文字の文字数を抽出するサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 104.0.5112.101
文字列内で一番使用されている文字の文字数を抽出する
文字列内で一番使用されている文字の文字数を抽出するには、「max・split・map」を使用することで可能です。
※ここでは、文字列は「 (半角スペース)」で区切られているものとします。
「Lorem Ipsum is simply dummy」という文章の中から、一番使用されている文字(単語)の文字数を取得してみます。
'use strict';
function f(str) {
return Math.max(...str.split(' ').map(w => w.length));
}
console.log( f('Lorem Ipsum is simply dummy') )
実行結果を確認すると、一番多い文字「dummy」の文字数が表示されていることが確認できます。
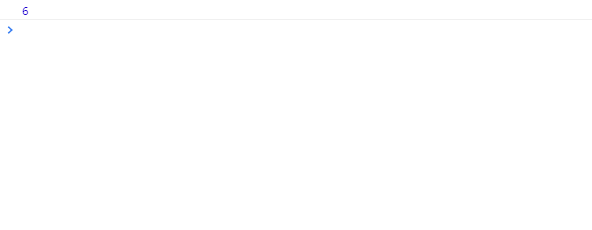
日本語の場合でも、同様に使用できます。
'use strict';
function f(str) {
return Math.max(...str.split(' ').map(w => w.length));
}
console.log( f('こんにちわ 世界 !!') ) // 5
コード簡略化
また、以下のコードを、
function f(str) {
return Math.max(...str.split(' ').map(w => w.length));
}
アロー関数を使用して、簡潔に記述することもできます。
btn.onclick = () =>{
const f = (str) =>{return Math.max(...str.split(' ').map(w => w.length))}
}
サンプルコード
以下は、
「実行」ボタンをクリックして、テキストの中にある文字列の使用されている文字(単語)の文字数を表示するサンプルコードとなります。
※cssには「tailwind」を使用して、アロー関数で関数は定義してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css" rel="stylesheet">
</head>
<script>
const hoge = () => {
foo.innerHTML = Math.max(...foo.innerHTML.split(' ').map(w => w.length))
}
window.onload = () => {
btn.onclick = () => { hoge() };
}
</script>
<body>
<div class="container mx-auto my-56 w-64 px-4">
<div id="sample" class="flex flex-col justify-center">
<h2 id="foo" class="font-semibold text-lg mr-auto">
Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s
</h2>
<button id="btn" class="mb-2 md:mb-0 bg-red-400 px-5 py-2 text-sm shadow-sm font-medium tracking-wider text-white rounded-full hover:shadow-lg hover:bg-red-500">
実行
</button>
</div>
</div>
</body>
</html>
実行結果を確認すると、最大文字数が表示されていることが確認できます。
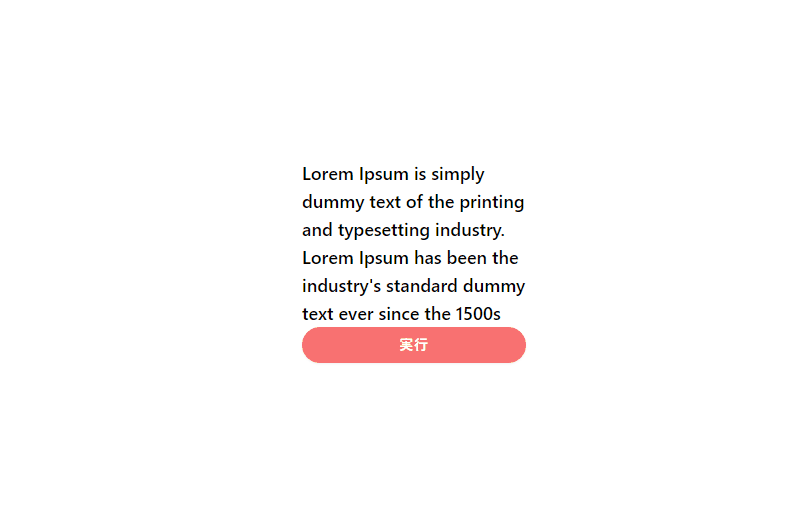
-
前の記事
Ruby ハッシュのキーを複数指定して値を取得する 2021.06.18
-
次の記事
python numpyの配列の値を削除する 2021.06.18
コメントを書く