javascript 文字列内から指定した文字列の1つ前の1文字を抽出する
- 作成日 2022.08.17
- 更新日 2022.10.14
- javascript
- javascript
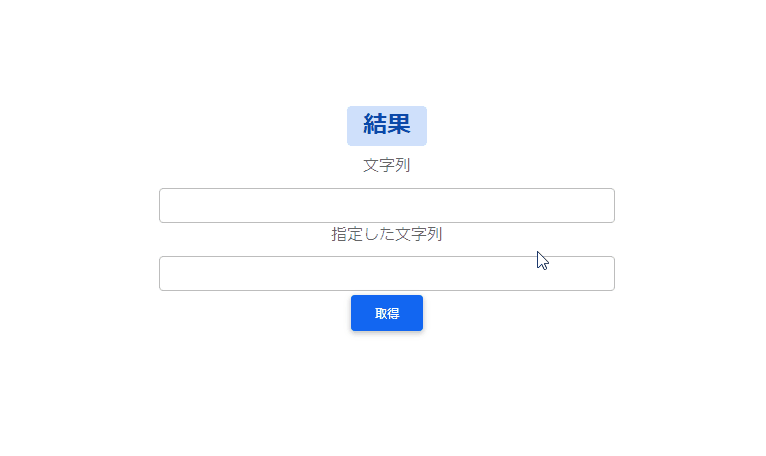
javascriptで、文字列内から指定した文字列の1つ前の1文字を抽出するサンプルコードを記述してます。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 106.0.5249.103
文字列内から指定した文字列の1つ前の1文字を抽出
文字列内から指定した文字列の1つ前の1文字を抽出するには「indexOf」で文字列の位置を取得した後に「substring」で1文字だけ抽出します。
const str = "abcde"
let position = str.indexOf('bc');
console.log( str.substring(position - 1, position) );
// a
前に文字が存在しない場合は、空となります。
const str = "abcde"
let position = str.indexOf('bc');
console.log( str.substring(position - 1, position) );
// a
後ろの1文字
逆に後ろの1文字を取得する場合は、以下となります。
const str = "abcde"
let selectStr = "bcd"
console.log( str.indexOf(selectStr) ) // 1
let position = str.indexOf(selectStr);
console.log( str.substring( position + 1 ) ); // cde
console.log( str.substring( position + 1 )[selectStr.length - 1 ] ); // e
サンプルコード
以下は、
「取得」ボタンをクリックすると、フォームに入力された文字列に対して、別のフォームに指定した文字列の前にある1文字を取得して表示する
サンプルコードとなります。
※cssには「bootstrap material」を使用してます。関数はアロー関数で記述してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<!-- MDB -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/mdb-ui-kit/4.2.0/mdb.min.css" rel="stylesheet" />
</head>
<body>
<div class="container text-center w-25" style="margin-top:150px">
<h2><span class="badge badge-primary">結果</span></h2>
<label class="form-label" for="txt1">文字列</label>
<input id="txt1" type="text" class="form-control" />
<label class="form-label" for="txt2">指定した文字列</label>
<input id="txt2" type="text" class="form-control" />
<button id="btn" type="button" class="btn mt-1 btn-primary">
取得
</button>
</div>
<script>
// クリックイベントを登録
btn.onclick = () => { hoge() };
const getBefore = (str) => {
let position = str.indexOf(txt2.value);
return str.substring(position - 1, position);
}
const hoge = () => {
document.getElementsByClassName('badge')[0].textContent = getBefore(txt1.value);
}
</script>
</body>
</html>
取得されていることが確認できます。
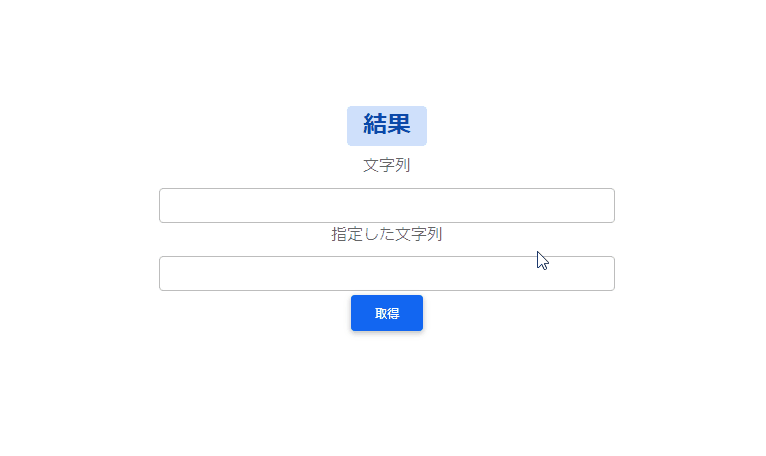
-
前の記事
Linux grep実行時にバイナリファイルを無視する 2022.08.17
-
次の記事
SQL Server レコード数をカウントする 2022.08.17
コメントを書く