javascript エラー「Uncaught DOMException: Failed to execute ‘setItem’ on ‘Storage’: Setting the value of ‘xxx’ exceeded the quota.」の解決方法
- 作成日 2022.08.09
- javascript
- javascript
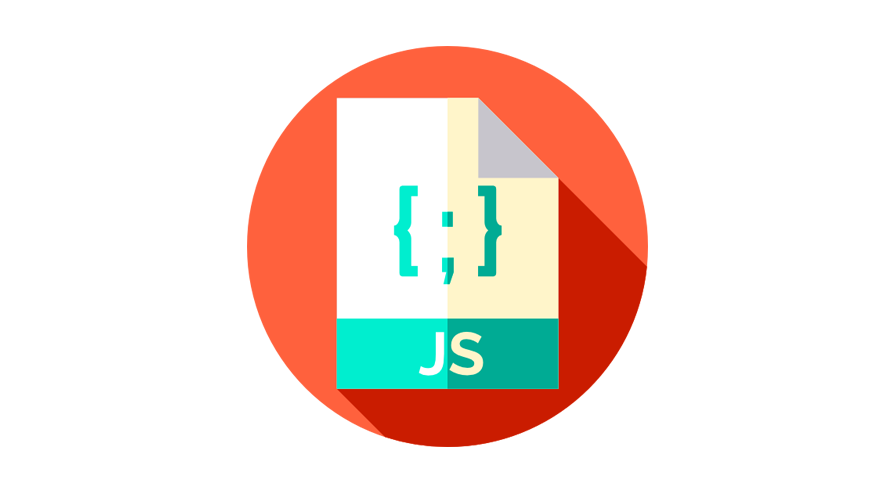
javascriptで、エラー「Uncaught DOMException: Failed to execute ‘setItem’ on ‘Storage’: Setting the value of ‘xxx’ exceeded the quota.」が発生した場合の原因と解決方法を記述してます。
環境
- OS windows11 pro 64bit
- ブラウザ chrome 103.0.5060.134
エラー内容
以下の、「sessionStorage」にデータを100万件挿入しようとしたコードを実行時に発生。
// ウェブストレージに対応している場合
if (window.localStorage) {
// 保存するオブジェクト
const obj = {
str1: 'Hello',
str2: 'World',
str3: '!!',
};
// オブジェクトをjsonに変換
const txt = JSON.stringify(obj);
let str = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ";
for (let i = 0; i < 1_000_000; i++) {
let randstr = '';
for (let i = 0; i < 10; i++) {
randstr += str[~~(Math.random() * str.length)];
}
// jsonデータをsessionStorageに保存
sessionStorage.setItem(randstr, txt);
}
console.log('finish')
}
エラーメッセージ
Uncaught DOMException: Failed to execute 'setItem' on 'Storage': Setting the value of 'GbEtdkLJRB' exceeded the quota.
画像

firefox102の場合は、以下のエラーが発生します。
Uncaught DOMException: The quota has been exceeded.
画像

原因
「sessionStorage」に保存できるデータの容量を超えてしまったため
解決方法
「try-catch」を使用して、制限を超えた場合はエラーを「catch」して処理を中断する
// ウェブストレージに対応している場合
if (window.localStorage) {
// 保存するオブジェクト
const obj = {
str1: 'Hello',
str2: 'World',
str3: '!!',
};
// オブジェクトをjsonに変換
const txt = JSON.stringify(obj);
let str = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ";
try {
for (let i = 0; i < 1000; i++) {
let randstr = '';
for (let i = 0; i < 100_000; i++) {
randstr += str[~~(Math.random() * str.length)];
}
// jsonデータをsessionStorageに保存
sessionStorage.setItem(randstr, txt);
}
}
catch (e) {
console.log("データがいっぱいになりました。");
}
console.log('処理が完了しました')
}
実行結果

-
前の記事
Ruby 文字列がASCII文字だけであるかを判定する 2022.08.09
-
次の記事
コマンドプロンプト NICに割り合っているMACアドレスを取得する 2022.08.09
コメントを書く