javascript テキストフォームにフォーカス時に最後の値の位置に移動する
- 作成日 2022.11.18
- javascript
- javascript
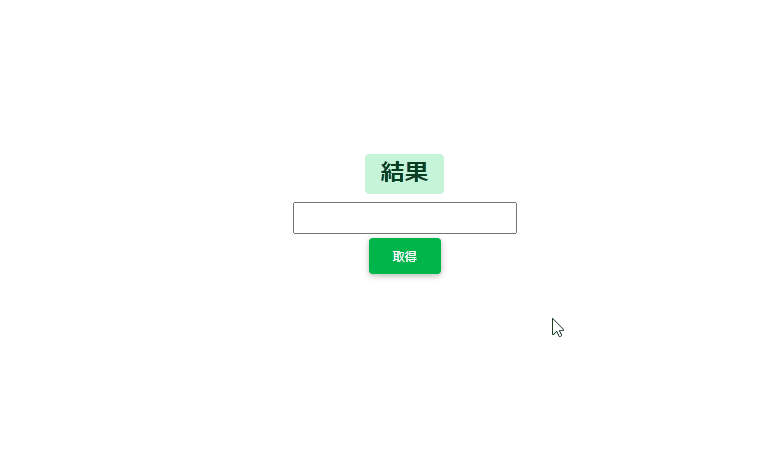
javascriptで、フォームにフォーカス時に最後の値の位置に移動するサンプルコードを記述してます。「length」を使用して長さから最終位置を取得して、それを選択範囲として「setSelectionRange」で使用することで可能です。
環境
- OS windows11 pro 64bit
- ブラウザ chrome 107.0.5304.88
最後の値の位置に移動
最後の値の位置に移動するには
1. 「フォーム」内の値の最後の位置を「length」で取得
2. 「setSelectionRange(開始,終了)」で選択範囲を「1.」で取得した位置に指定
で可能です。
<input type="text" value="mebee" id="txt">
<button onclick="hoge();" id="btn">実行</button>
<script>
function hoge(){
// テキストの要素を取得
let obj = document.getElementById("txt");
// 最終位置を取得
const end = obj.value.length;
// フォーカス
obj.focus();
// 最後の文字の位置を選択状態に変更
obj.setSelectionRange(end, end);
}
</script>
実行結果
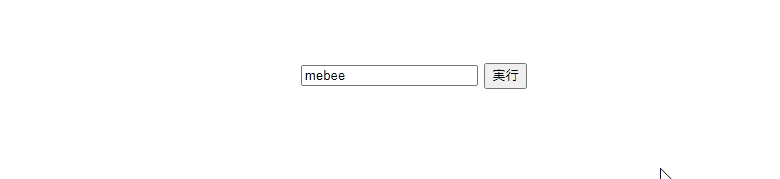
存在チェック
存在しない要素を指定するとエラーとなります。
<input type="text" value="mebee" id="txt">
<button onclick="hoge();" id="btn">実行</button>
<script>
function hoge(){
// テキストの要素を取得
let obj = document.getElementById("noelm");
// 最終位置を取得
const end = obj.value.length;
// Uncaught TypeError: Cannot read properties of null (reading 'value')
// フォーカス
obj.focus();
// 最後の文字の位置を選択状態に変更
obj.setSelectionRange(end, end);
}
</script>
存在チェックをしておくとエラーは回避できます。
<script>
function hoge() {
// テキストの要素を取得
let obj = document.getElementById("noelm");
if (obj !== null) {
// 最終位置を取得
const end = obj.value.length;
// Uncaught TypeError: Cannot read properties of null (reading 'value')
// フォーカス
obj.focus();
// 最後の文字の位置を選択状態に変更
obj.setSelectionRange(end, end);
}
}
</script>
サンプルコード
以下は、
「 実行 」ボタンをクリックすると、フォームに入力された文字列の最後に位置にフォーカスするサンプルコードとなります。
※cssには「bootstrap material」を使用してます。関数はアロー関数で記述してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<!-- MDB -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/mdb-ui-kit/4.2.0/mdb.min.css" rel="stylesheet" />
</head>
<body>
<div class="container text-center w-50" style="margin-top:200px">
<h2><span class="badge badge-success">結果</span></h2>
<form>
<div class="form-group">
<input type="text" id="setData">
</div>
</form>
<button type="button" onclick="handleClick()" class="btn btn-success mt-1">
取得
</button>
</div>
<script>
const handleClick = () => {
// 最終位置を取得
const end = setData.value.length;
// フォーカス
setData.focus();
// 最後の文字の位置を選択状態に変更
setData.setSelectionRange(end, end);
}
</script>
</body>
</html>
移動していることが確認できます。
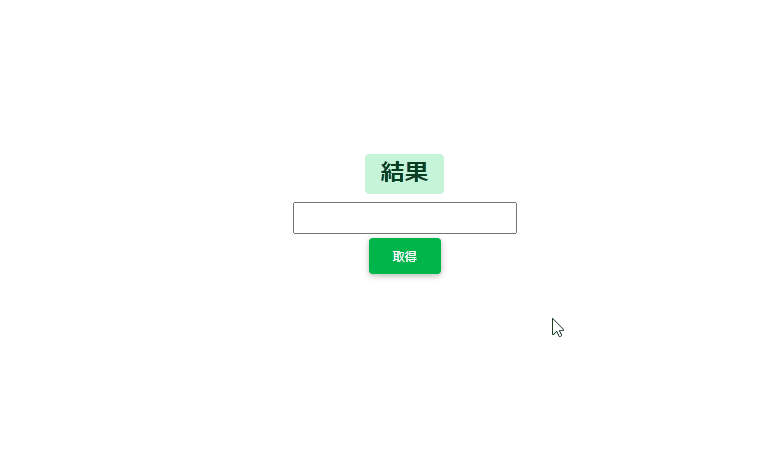
-
前の記事
SourceTree 未追跡のファイルを削除するショートカットキー 2022.11.17
-
次の記事
javascript オブジェクトの最初の値を取得する 2022.11.18
コメントを書く