javascript 最大公倍数を求める
- 作成日 2021.05.14
- 更新日 2022.08.24
- javascript
- javascript
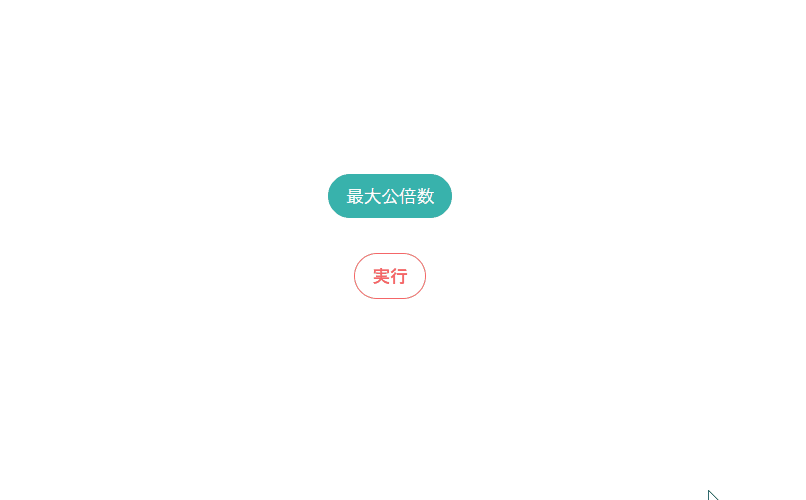
javascriptで、最大公倍数を求めるサンプルコードを記述してます。値が複数個ある場合でも、求めることは可能です。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 104.0.5112.10
最大公倍数
以下のコードで、最大公倍数を求めることが可能です。
const lcm = (n, m) => {
const gcd = (x, y) => y ? gcd(y, x % y) : x
return n * m / gcd(n, m)
}
console.log(lcm(15, 25))
// 75
値が複数個ある場合は、argumentsを使うことで計算することが可能です
※アロー関数は、argumentsは使用できません。
function lcm() {
const g = (n, m) => m ? g(m, n % m) : n
const l = (n, m) => n * m / g(n, m)
let ans = arguments[0]
for (let i = 1; i < arguments.length; i++) {
ans = l(ans, arguments[i])
}
return ans
}
console.log(lcm(15,25,50))
// 150
サンプルコード
以下は、
「実行」ボタンをクリックすると、ランダムな2桁の配列を生成して最大公倍数を求める
サンプルコードとなります。
※cssには「tailwind」を使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
</head>
<script>
function hoge() {
// ランダムな2桁の配列を生成
const arr = Array(2).fill().map(x => ~~(Math.random() * 100));
// 生成した配列を表示
disp(arr, "rand");
// 最大公倍数を計算する関数
const gcd = (x, y) => y ? gcd(y, x % y) : x
const f = (x, y) => x * y / gcd(x, y);
// 最大公倍数を表示
result.innerHTML = f(arr[0],arr[1]);
}
//フロントに表示する関数
function disp(arr, id) {
let text = [];
// 配列を利用してfor文を作成
[...arr].forEach((x, i) => text.push('<li class="list-group-item">' + arr[i] + '</li>'))
//innerHTMLを使用して表示
document.getElementById(id).innerHTML = text.join('');
}
window.onload = () => {
// クリックイベントを登録
btn.onclick = () => { hoge(); }; // document.getElementById('btn');を省略
}
</script>
<body>
<div class="container mx-auto my-56 w-56 px-4">
<div class="flex justify-center">
<p id="result" class="bg-teal-500 text-white py-2 px-4 rounded-full mb-3 mt-4">最大公倍数</p>
</div>
<div class="flex justify-center">
<ul id="rand"></ul>
</div>
<div class="flex justify-center">
<button id="btn" type="button"
class="mt-5 bg-transparent border border-red-500 hover:border-red-300 text-red-500 hover:text-red-300 font-bold py-2 px-4 rounded-full">
実行
</button>
</div>
</div>
</body>
</html>
最大公倍数が計算されていることが確認できます。
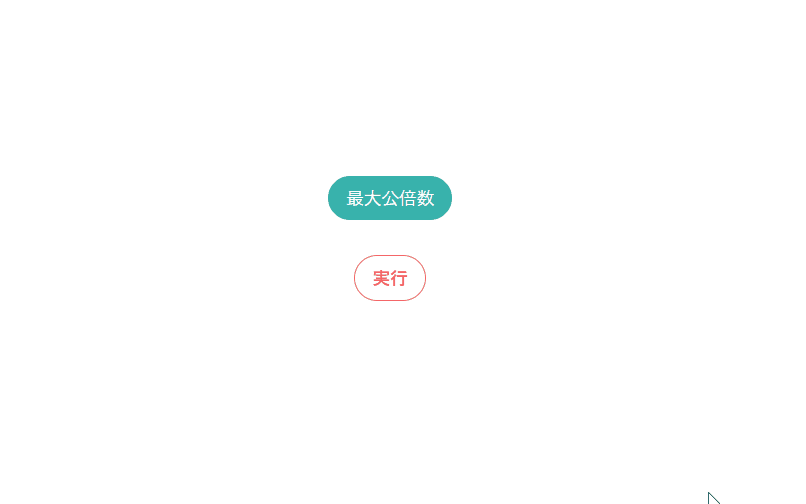
-
前の記事
Ubuntu21.04 最新版のsqlite3をインストールする 2021.05.14
-
次の記事
SQL Server 各テーブルのレコード数を取得する 2021.05.14
コメントを書く