javascript removeでhtml要素を削除する
- 作成日 2021.04.01
- 更新日 2022.08.12
- javascript
- javascript
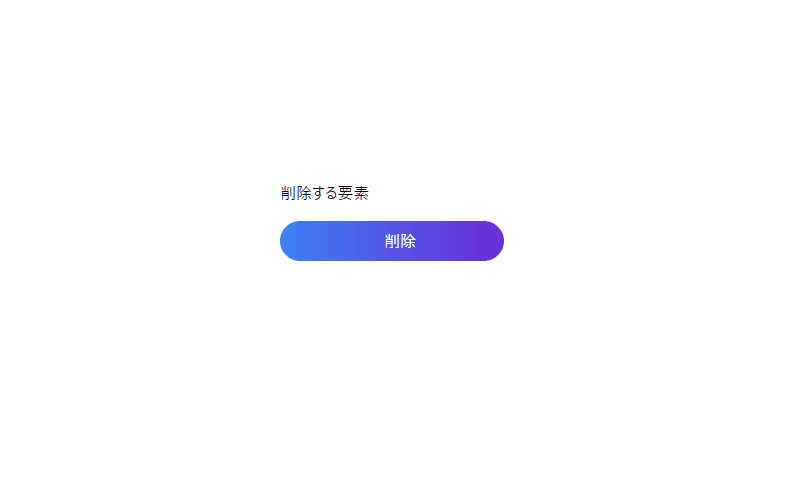
javascriptで、removeを使用して、html要素を削除するサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 104.0.5112.81
remove使い方
「remove」を使用すると、html要素を削除することが可能です。
ノード.remove
remove使い方
<div id="main">
<div id="one">one</div>
<div id="two">two</div>
</div>
<script>
'use strict';
const elm = document.getElementById("one");
elm.remove();
</script>
実行結果を見ると、要素が削除されているが確認できます。
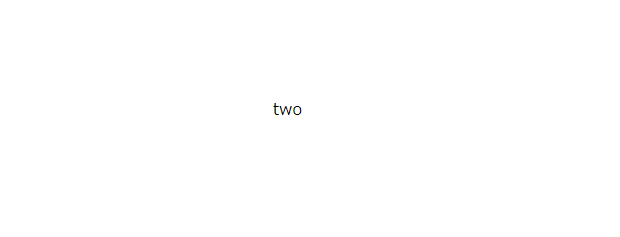

複数の要素を削除
「getElementsByClassName」でクラス名を指定して「remove」を使用すると「HTMLCollection」のためエラーが発生します。
<div id="main">
<div id="one" class="hoge">one</div>
<div id="two" class="foo">two</div>
<div id="two" class="foo">three</div>
<div id="two" class="foo">four</div>
</div>
<script>
'use strict';
const elm = document.getElementsByClassName('foo');
elm.remove();
// Uncaught TypeError: elm.remove is not a function
</script>
「HTMLCollection」なので、ループ処理を使用すれば削除可能です。
<div id="main">
<div id="one" class="hoge">one</div>
<div id="two" class="foo">two</div>
<div id="two" class="foo">three</div>
<div id="two" class="foo">four</div>
</div>
<script>
'use strict';
const elm = document.getElementsByClassName('foo');
if (0 < elm.length) {
while (elm.length) {
elm.item(0).remove()
}
}
</script>
実行結果
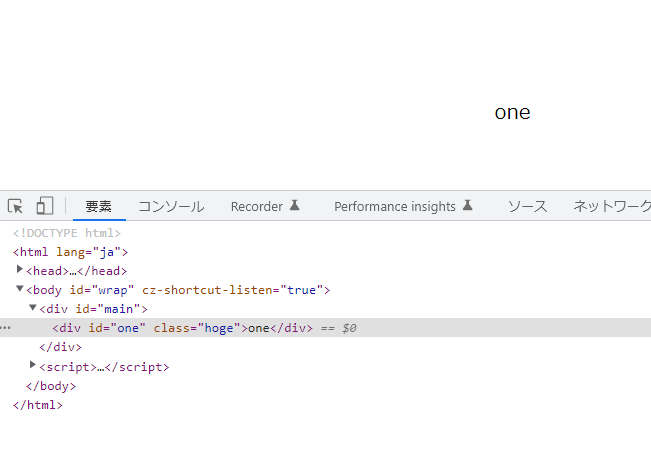
存在しないノードを指定
存在しないノードを指定すると、エラーが発生します。
<div id="main">
<div id="one">one</div>
<div id="two">two</div>
</div>
<script>
'use strict';
const elm = document.getElementById("noelm");
// Uncaught TypeError: Cannot read properties of null (reading 'remove')
elm.remove();
</script>
「エラー」を回避するなら、存在確認を行います。
<div id="main">
<div id="one">one</div>
<div id="two">two</div>
</div>
<script>
'use strict';
const elm = document.getElementById("noelm");
if(elm !== null) elm.remove();
</script>
コードの簡潔化
また、以下のコードを、
const elm = document.getElementById("one");
elm.remove();
document.getElementByIdの省略を使用して、簡潔に記述することもできます。
one.remove();
サンプルコード
以下は、
「削除」ボタンをクリックして、html要素を削除する
サンプルコードとなります。
※cssには「tailwind」を使用して、アロー関数で関数は定義してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css" rel="stylesheet">
</head>
<script>
window.onload = () => {
btn.onclick = () => {
chg.remove();
};
}
</script>
<body>
<div class="container mx-auto my-56 w-64 px-4">
<p id="chg">削除する要素</p>
<div id="sample" class="flex flex-col justify-center">
<button id="btn"
class="bg-gradient-to-r from-blue-500 to-purple-700 hover:from-pink-500 hover:to-yellow-500 text-white py-2 px-4 rounded-full mb-3 mt-4">
削除
</button>
</div>
</div>
</body>
</html>
html要素が削除されていることが確認できます。
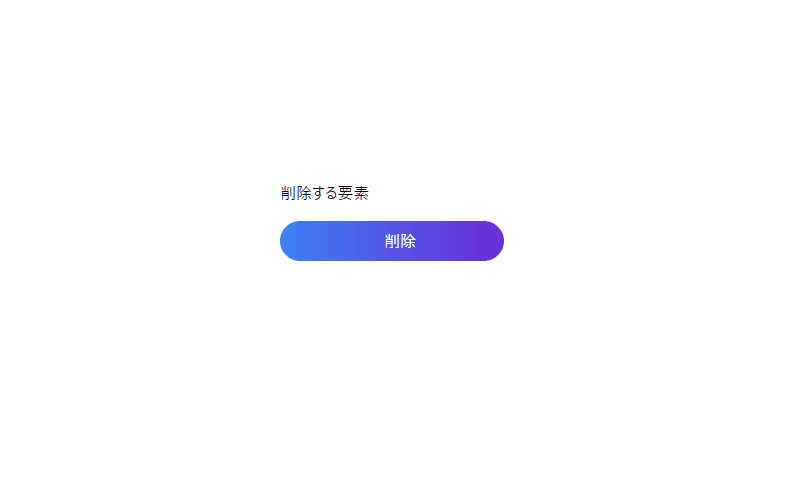
-
前の記事
python openpyxlを使ってEXCELの枠線を作成する 2021.03.31
-
次の記事
dockerでrails6とpostgresql環境を構築する 2021.04.01
コメントを書く