javascript 今月末の日付を取得する
- 作成日 2021.05.18
- 更新日 2022.08.27
- javascript
- javascript
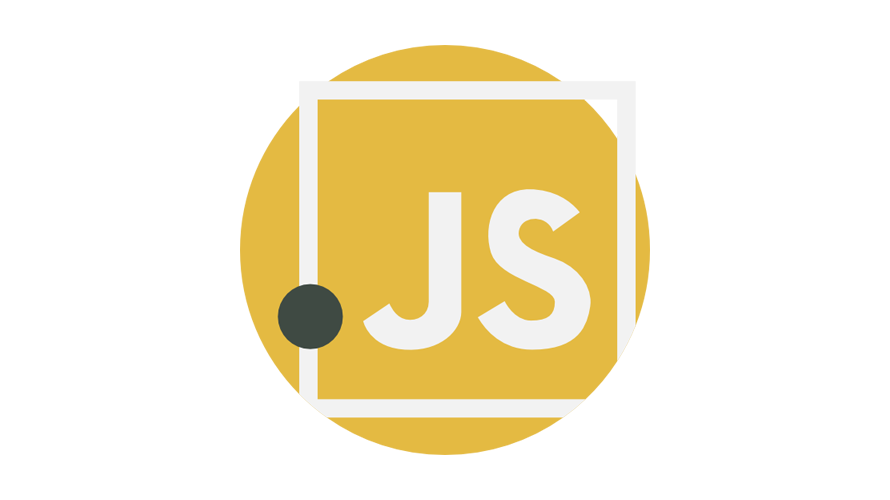
javascriptで今月末の日付を取得するサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 104.0.5112.101
今月末の日付を取得
「now.getFullYear(), now.getMonth() + 1, 0」で、昨日の日付を取得することが可能です。これを利用して取得します。
<input id="btn" type="button" value="ボタン"/>
<script>
'use strict';
document.getElementById('btn').onclick = function(){
const now = new Date();
console.log( new Date() );
console.log( new Date(now.getFullYear(), now.getMonth() + 1, 0) );
}
</script>
実行結果
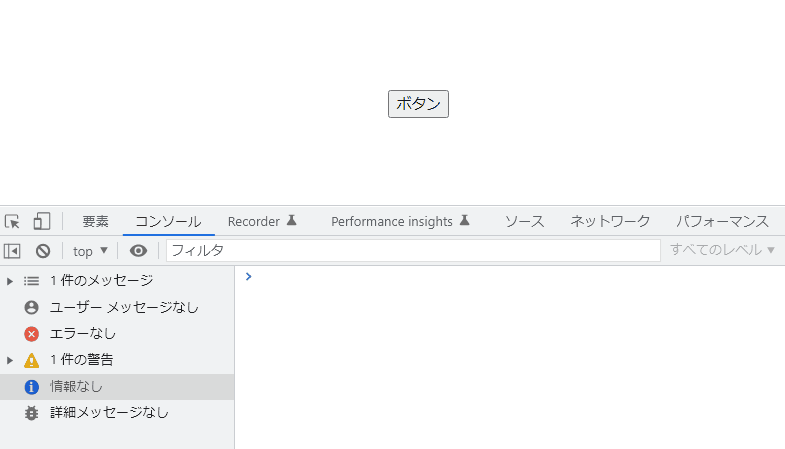
フォーマットを変更する場合は、以下のようにテンプレートリテラルを使用します。
'use strict';
document.getElementById('btn').onclick = function () {
const now = new Date()
let day = new Date(now.getFullYear(), now.getMonth() + 1, 0);
day = `${day.getFullYear()}-${(day.getMonth() + 1).toString().padStart(2, '0')}-${day.getDate().toString().padStart(2, '0')}`.replace(/\n|\r/g, '');
console.log(day);
}
実行結果
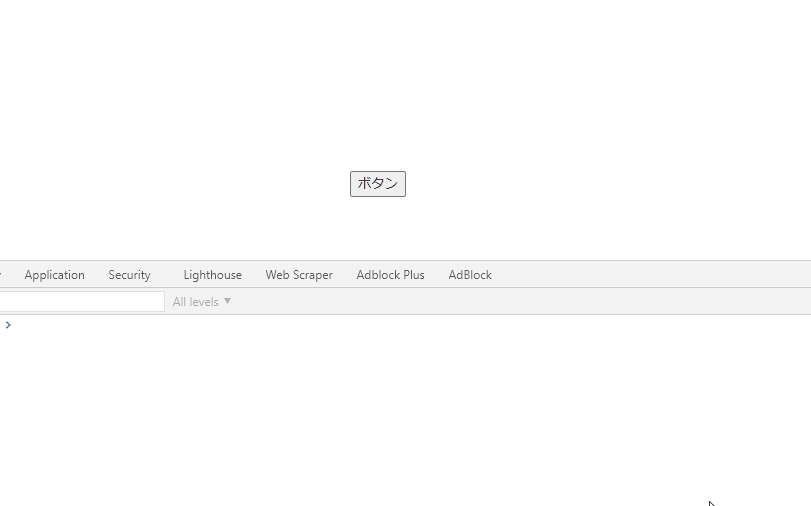
翌月を取得
翌月の場合は「+2」します。
'use strict';
document.getElementById('btn').onclick = function () {
const now = new Date()
let day = new Date(now.getFullYear(), now.getMonth() + 2, 0);
day = `${day.getFullYear()}-${(day.getMonth() + 1).toString().padStart(2, '0')}-${day.getDate().toString().padStart(2, '0')}`.replace(/\n|\r/g, '');
console.log(day);
}
実行結果
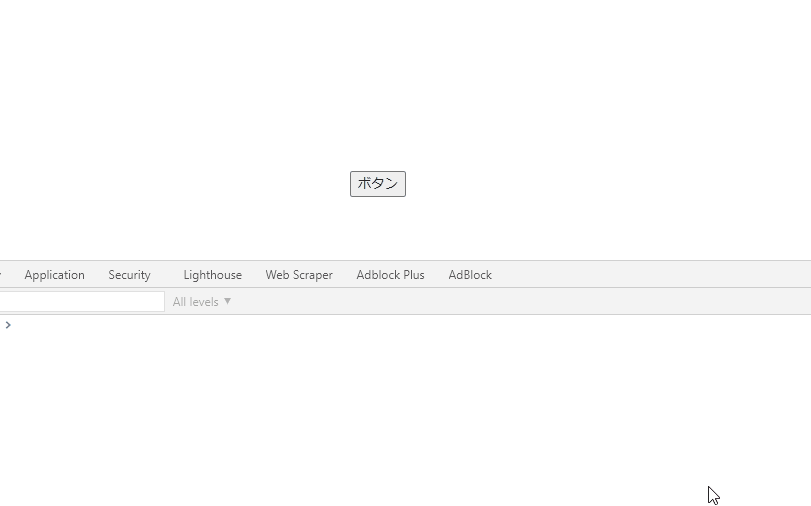
先月を取得
先月の場合は、そのまま「0」だけを指定します。
'use strict';
document.getElementById('btn').onclick = function () {
const now = new Date()
let day = new Date(now.getFullYear(), now.getMonth(), 0);
day = `${day.getFullYear()}-${(day.getMonth() + 1).toString().padStart(2, '0')}-${day.getDate().toString().padStart(2, '0')}`.replace(/\n|\r/g, '');
console.log(day);
}
実行結果
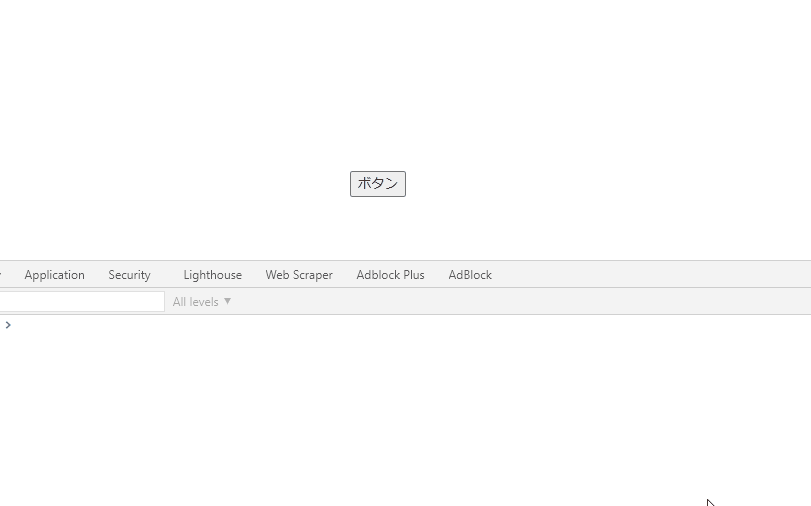
コードの簡潔化
また、以下のコードを、
document.getElementById('btn').onclick = function () {
const now = new Date()
let day = new Date(now.getFullYear(), now.getMonth(), 0);
day = `${day.getFullYear()}-${(day.getMonth() + 1).toString().padStart(2, '0')}-${day.getDate().toString().padStart(2, '0')}`.replace(/\n|\r/g, '');
console.log(day);
}
アロー関数とdocument.getElementByIdを省略して、簡潔に記述することもできます。
btn.onclick = () => {
const now = new Date()
let day = new Date(now.getFullYear(), now.getMonth(), 0);
day = `${day.getFullYear()}-${(day.getMonth() + 1).toString().padStart(2, '0')}-${day.getDate().toString().padStart(2, '0')}`.replace(/\n|\r/g, '');
console.log(day);
}
-
前の記事
gitlab メール送信機能を設定する 2021.05.17
-
次の記事
AlmaLinux SELinuxを無効にする 2021.05.18
コメントを書く