javascript canvasタグに正方形のグリッドを作成する
- 作成日 2020.10.06
- 更新日 2022.07.05
- javascript
- javascript
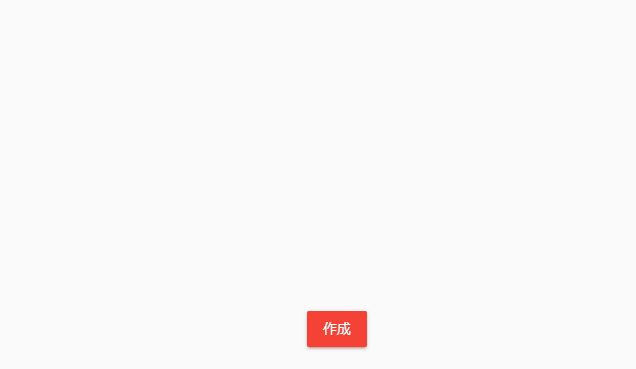
javascriptで、canvasタグを使って、正方形のグリッドを作成するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 103.0.5060.66
canvasタグ使い方
for文で、小さい正方形を、色を変更しながら作成します。
<canvas id="cvs"></canvas>
<script>
// 2D図形を扱う
let ctx = document.getElementById('cvs').getContext('2d');
// for文を利用
for (let i = 0; i < 5; i++) {
for (let j = 0; j < 5; j++) {
// 赤と青を変化させる
ctx.fillStyle = `rgb(${Math.floor(255 - 51 * i)}, 0, ${Math.floor(255 - 51 * j)})`;
// fillRect(開始x座標, 開始y座標, 描画幅, 描画高さ)
ctx.fillRect(j * 55, i * 55, 45, 45);
}
}
</script>
実行結果
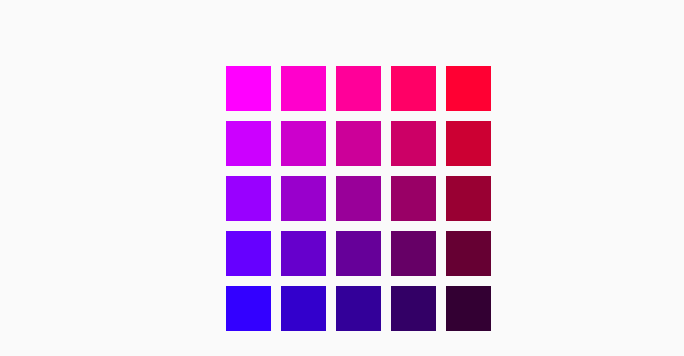
サンプルコード
以下は、
「作成」ボタンをクリックすると、canvasタグにランダムな配色の25個の正方形を作成する
サンプルコードとなります。
※cssには「bootstrap material」を使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700|Material+Icons">
<link rel="stylesheet"
href="https://unpkg.com/bootstrap-material-design@4.1.1/dist/css/bootstrap-material-design.min.css" id="css">
</head>
<style>
.main {
margin: 0 auto;
margin-top: 200px;
display: flex;
flex-direction: column;
align-items: center;
font-size: 30px;
}
</style>
<script>
function hoge() {
// 2D図形を扱う
let ctx = document.getElementById('cvs').getContext('2d');
for (let i = 0; i < 5; i++) {
for (let j = 0; j < 5; j++) {
// 赤と緑と青を変化させる
ctx.fillStyle = `rgb(${Math.floor(255 - Math.random() * 255)}, ${Math.floor(255 - Math.random() * 255)}, ${Math.floor(255 - Math.random() * 255)})`;
// fillRect(開始x座標, 開始y座標, 描画幅, 描画高さ)
ctx.fillRect(j * 55, i * 55, 45, 45);
}
}
}
window.onload = function () {
// ボタンを取得
let elmbtn = document.getElementById('btn');
// クリックイベントを登録
elmbtn.onclick = function () {
hoge();
};
}
</script>
<body>
<div class="main">
<canvas id="cvs" height="300"></canvas>
<button id="btn" type="button" class="btn btn-raised btn-danger">
作成
</button>
</div>
</body>
</html>
ランダムな配色の25個の正方形が作成されていることが確認できます。
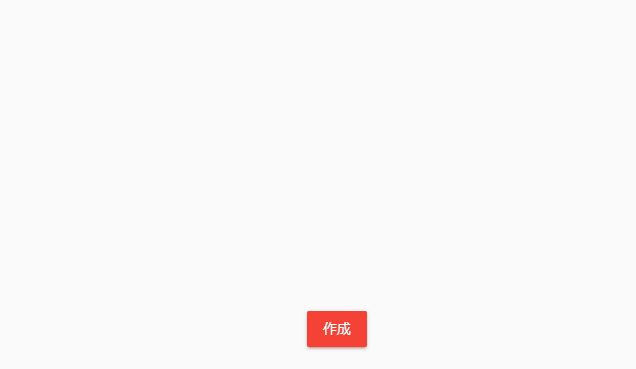
-
前の記事
classを利用しないクラスレスのCSSフレームワーク「tacit」を使用する手順 2020.10.06
-
次の記事
C# ラムダ式の簡単な使い方 2020.10.06
コメントを書く