React.js ライブラリ「react-select-search」を使って軽量の選択式のコンポーネントを利用する
- 作成日 2020.07.14
- 更新日 2020.07.17
- React
- react-select-search, React.js, インストール, ライブラリ
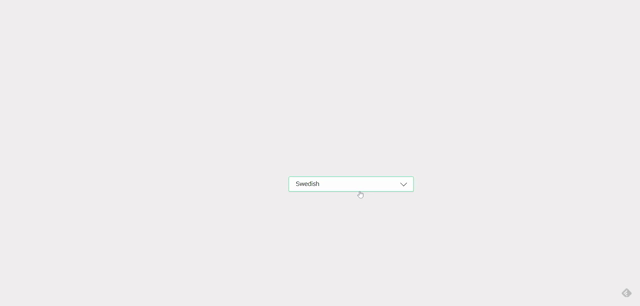
ライブラリ「react-select-search」をインストールすると、軽量の選択式のコンポーネントを利用することが可能です。ここでは、react.jsで react-select-searchを利用するための手順と簡単な使い方を記述してます。
環境
- OS CentOS Linux release 8.0.1905 (Core)
- node V12.13.1
- npm 6.14.2
- React 16.13.0
react.js環境構築
下記のコマンドで構築してます。ここでは、react-appという名前でプロジェクトを作成してます。
create-react-app react-app
react-select-searchインストール
作成したプロジェクトに移動して、インストールします。
## 作成したプロジェクトに移動
cd react-app
## インストール
npm install react-select-search
react-select-search使い方
srcディレクトリにsample.jsと名前で下記のコードを記述します。
import React from 'react';
import SelectSearch from 'react-select-search';
import './style.css';
const Sample = () => {
const options = [
{name: 'Swedish', value: 'sv'},
{name: 'English', value: 'en'},
{
type: 'group',
name: 'Group name',
items: [
{name: 'Spanish', value: 'es'},
]
},
];
return (
<div>
<SelectSearch options={options} value="sv" name="language" placeholder="Choose your language" />
</div>
);
}
export default Sample;
srcディレクトリ配下のstyle.cssを下記のように編集します。
html,
body,
#root {
width: 100%;
height: 100%;
margin: 0;
padding: 0;
background-color: white;
}
body {
font-family: -apple-system, BlinkMacSystemFont, avenir next, avenir, helvetica neue, helvetica, ubuntu,
roboto, noto, segoe ui, arial, sans-serif;
background: transparent;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
cursor: default;
}
#root {
display: flex;
align-items: center;
justify-content: center;
overflow: hidden;
background: #f0f0f0;
}
.card {
width: 45ch;
height: 45ch;
background: grey;
border-radius: 5px;
background-image: url(https://cdn.pixabay.com/photo/2020/03/03/15/47/landscape-4899037_1280.jpg);
background-size: cover;
background-position: center center;
box-shadow: 0px 10px 30px -5px rgba(0, 0, 0, 0.3);
transition: box-shadow 0.5s;
will-change: transform;
border: 15px solid white;
}
.card:hover {
box-shadow: 0px 30px 100px -10px rgba(0, 0, 0, 0.4);
}
.select-search {
width: 300px;
position: relative;
font-family: 'Nunito Sans', sans-serif;
box-sizing: border-box;
}
.select-search *,
.select-search *::after,
.select-search *::before {
box-sizing: inherit;
}
/**
* Value wrapper
*/
.select-search__value {
position: relative;
z-index: 1;
}
.select-search__value::after {
content: '';
display: inline-block;
position: absolute;
top: calc(50% - 9px);
right: 19px;
width: 11px;
height: 11px;
}
/**
* Input
*/
.select-search__input {
display: block;
height: 36px;
width: 100%;
padding: 0 16px;
background: #fff;
border: 1px solid transparent;
box-shadow: 0 .0625rem .125rem rgba(0, 0, 0, 0.15);
border-radius: 3px;
outline: none;
font-family: 'Noto Sans', sans-serif;
font-size: 14px;
text-align: left;
text-overflow: ellipsis;
line-height: 36px;
-webkit-appearance: none;
}
.select-search__input::-webkit-search-decoration,
.select-search__input::-webkit-search-cancel-button,
.select-search__input::-webkit-search-results-button,
.select-search__input::-webkit-search-results-decoration {
-webkit-appearance:none;
}
.select-search__input:not([readonly]):focus {
cursor: initial;
}
/**
* Options wrapper
*/
.select-search__select {
background: #fff;
box-shadow: 0 .0625rem .125rem rgba(0, 0, 0, 0.15);
}
/**
* Options
*/
.select-search__options {
list-style: none;
}
/**
* Option row
*/
.select-search__row:not(:first-child) {
border-top: 1px solid #eee;
}
/**
* Option
*/
.select-search__option {
display: block;
height: 36px;
width: 100%;
padding: 0 16px;
background: #fff;
border: none;
outline: none;
font-family: 'Noto Sans', sans-serif;
font-size: 14px;
text-align: left;
cursor: pointer;
}
.select-search--multiple .select-search__option {
height: 48px;
}
.select-search__option.is-selected {
background: #2FCC8B;
color: #fff;
}
.select-search__option.is-highlighted,
.select-search__option:not(.is-selected):hover {
background: rgba(47, 204, 139, 0.1);
}
.select-search__option.is-highlighted.is-selected,
.select-search__option.is-selected:hover {
background: #2eb378;
color: #fff;
}
/**
* Group
*/
.select-search__group-header {
font-size: 10px;
text-transform: uppercase;
background: #eee;
padding: 8px 16px;
}
/**
* States
*/
.select-search.is-disabled {
opacity: 0.5;
}
.select-search.is-loading .select-search__value::after {
background-image: url("data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' width='50' height='50' viewBox='0 0 50 50'%3E%3Cpath fill='%232F2D37' d='M25,5A20.14,20.14,0,0,1,45,22.88a2.51,2.51,0,0,0,2.49,2.26h0A2.52,2.52,0,0,0,50,22.33a25.14,25.14,0,0,0-50,0,2.52,2.52,0,0,0,2.5,2.81h0A2.51,2.51,0,0,0,5,22.88,20.14,20.14,0,0,1,25,5Z'%3E%3CanimateTransform attributeName='transform' type='rotate' from='0 25 25' to='360 25 25' dur='0.6s' repeatCount='indefinite'/%3E%3C/path%3E%3C/svg%3E");
background-size: 11px;
}
.select-search:not(.is-disabled) .select-search__input {
cursor: pointer;
}
/**
* Modifiers
*/
.select-search--multiple {
border-radius: 3px;
overflow: hidden;
}
.select-search:not(.is-loading):not(.select-search--multiple) .select-search__value::after {
transform: rotate(45deg);
border-right: 1px solid #000;
border-bottom: 1px solid #000;
pointer-events: none;
}
.select-search--multiple .select-search__input {
cursor: initial;
}
.select-search--multiple .select-search__input {
border-radius: 3px 3px 0 0;
}
.select-search--multiple:not(.select-search--search) .select-search__input {
cursor: default;
}
.select-search:not(.select-search--multiple) .select-search__input:hover {
border-color: #2FCC8B;
}
.select-search:not(.select-search--multiple) .select-search__select {
position: absolute;
z-index: 2;
top: 44px;
right: 0;
left: 0;
border-radius: 3px;
overflow: auto;
max-height: 360px;
}
.select-search--multiple .select-search__select {
position: relative;
overflow: auto;
max-height: 260px;
border-top: 1px solid #eee;
border-radius: 0 0 3px 3px;
}
次に、srcディレクトリ配下にあるApp.jsを下記のように編集します。
import React from 'react';
import Sample from './sample';
import './App.css';
function App() {
const style = {
width: "50%",
margin: "0 auto",
marginTop: 150,
};
return (
<div className="App">
<div style={style}>
<Sample />
</div>
</div>
);
}
export default App;
実行します。
npm start
ブラウザから http://プライベートIP:3000にアクセスすると、 選択式のコンポーネントが生成されてます。
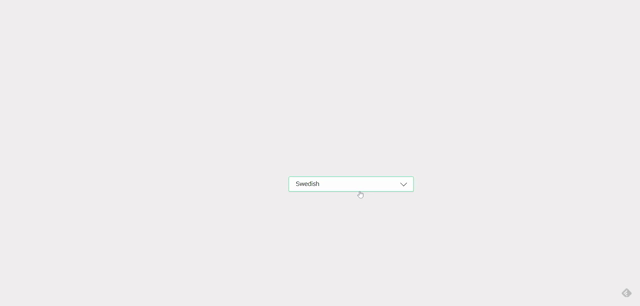
-
前の記事
apache chromeでERR_UNSAFE_PORTが発生した場合の対処法 2020.07.14
-
次の記事
docker-composeを使ってアクセス解析ツール「matomo(piwik)」を構築する手順 2020.07.14
コメントを書く