javascript エラー「Uncaught TypeError: Cannot write private member #xxx to an object whose class did not declare it」の解決方法
- 作成日 2023.01.18
- javascript
- javascript
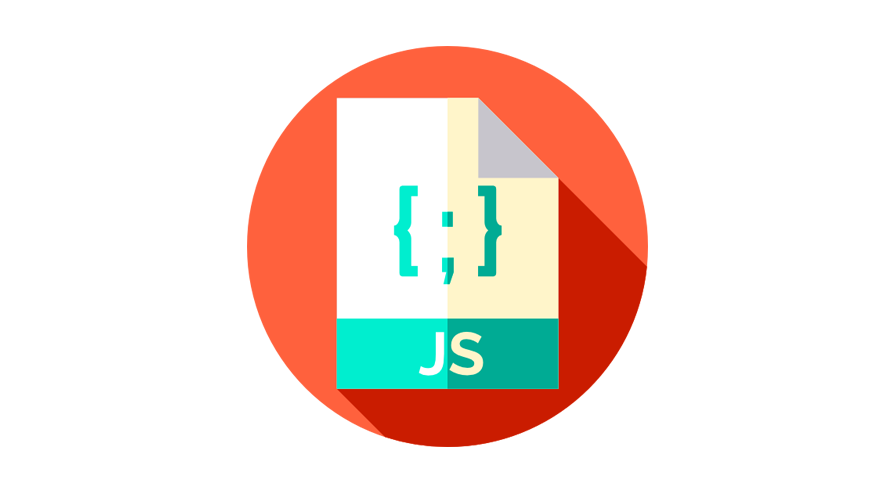
javascriptで、エラー「Uncaught TypeError: Cannot write private member #xxx to an object whose class did not declare it」が発生した場合の原因と解決方法を記述してます。「chrome」や「firefox」や「safari」の各ブラウザのエラーメッセージの画像もキャプチャしてます。
環境
- OS windows11 pro 64bit
- ブラウザ chrome 109.0.5414.75
エラー内容
以下の、「クラス」内で「static」で設定したプライベートフィールドに「this」でアクセスしようとしたコードで発生。
class Myclass {
static #n;
constructor(n) {
this.#n = n; // Uncaught TypeError: Cannot write private member #n to an object whose class did not declare it
}
#calc() {
return this.#n * 2;
}
calc() {
console.log(this.#calc());
}
}
const obj = new Myclass(10);
エラーメッセージ
Uncaught TypeError: Cannot write private member #n to an object whose class did not declare it
画像

firefox107の場合では、以下のエラーが発生します。
Uncaught TypeError: can't set private field: object is not the right class
画像

safari15.5では、以下のエラーとなります。
TypeError: Cannot access invalid private field (evaluating 'this.#n = n')
画像

解決方法
「this」をクラス名に置き換えます。
class Myclass {
static #n;
constructor(n) {
Myclass.#n = n;
}
#calc() {
return Myclass.#n * 2;
}
calc() {
console.log(this.#calc());
}
}
const obj = new Myclass(10);
obj.calc(); // 20
その他のエラー
サブクラスから利用
サブクラスから「this」で指定したプライベートプロパティにアクセスしようとしても同じようなエラーが発生します。
class Myclass {
static #n = 1;
calc() {
console.log(this.#n); // Uncaught TypeError: Cannot read private member #n from an object whose class did not declare it
}
}
class childMyclass extends Myclass {
};
const obj = new childMyclass();
obj.calc();
こちらも解決方法は同じで「this」をクラス名に置き換えます。
class Myclass {
static #n = 1;
calc() {
console.log(this.#n); // Uncaught TypeError: Cannot read private member #n from an object whose class did not declare it
}
}
class childMyclass extends Myclass {
};
const obj = new childMyclass();
obj.calc();
-
前の記事
python mongoDBに接続してデータを追加する 2023.01.18
-
次の記事
Dart webdriverでexecuteを使用してjsを実行する 2023.01.18
コメントを書く