javascript プライベートプロパティやメソッドを使用する
- 作成日 2022.12.31
- javascript
- javascript
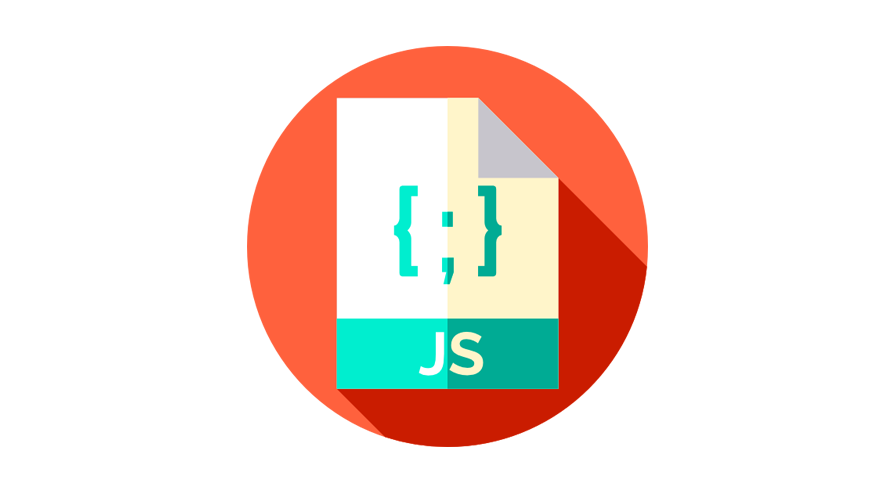
javascriptで、プライベートプロパティやメソッドを使用するサンプルコードを記述してます。接頭辞に「#」を使用して宣言することでプライベートとして使用できます。「in」を使用してアクセスすると「false」が返ります。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 108.0.5359.99
プライベートプロパティやメソッドを使用
プライベートプロパティやメソッドを使用するには
1. 前に「#(シャープ)」を追加
で可能です。
class Hoge {
#num;
constructor(num) {
this.#num = num;
}
#calc() {
return this.#num * 2;
}
calc() {
console.log(this.#calc());
}
}
const hoge = new Hoge(10);
hoge.calc(); // 20
プライベートに設定したプロパティやメソッドを使用するとエラーになります。
class Hoge {
#num;
constructor(num) {
this.#num = num;
}
#calc() {
return this.#num * 2;
}
calc() {
console.log(this.#calc());
}
}
const hoge = new Hoge(10);
console.log(hoge.#num);
// Uncaught SyntaxError: Private field '#num' must be declared in an enclosing class
console.log(hoge.#calc());
// Uncaught SyntaxError: Private field '#calc' must be declared in an enclosing class
inを使用
「in」からもアクセスできません。
class Hoge {
#num;
constructor(num) {
this.#num = num;
this._num = num;
}
#calc() {
return this.#num * 2;
}
calc() {
console.log(this.#calc());
}
}
const hoge = new Hoge(10);
console.log("#num" in hoge); // false
console.log("_num" in hoge); // true
static
staticで宣言してもアクセスするとエラーになります。
class Hoge {
static #num = 1;
static str = 'aaa';
constructor(num) {
Hoge.#num = num;
}
#calc() {
return Hoge.#num * 2;
}
calc() {
console.log(this.#calc());
}
}
const hoge = new Hoge(10);
console.log(Hoge.str);
// aaa
console.log(Hoge.#num);
// Uncaught SyntaxError: Private field '#num' must be declared in an enclosing class
-
前の記事
PostgreSQL 正規表現に一致した文字列を置換する 2022.12.30
-
次の記事
GAS スプレッドシートの指定したセルにURLで参照した画像を挿入する 2022.12.31
コメントを書く