javascript URLから「#(シャープ)」以降の値を取得する
- 作成日 2022.12.27
- javascript
- javascript
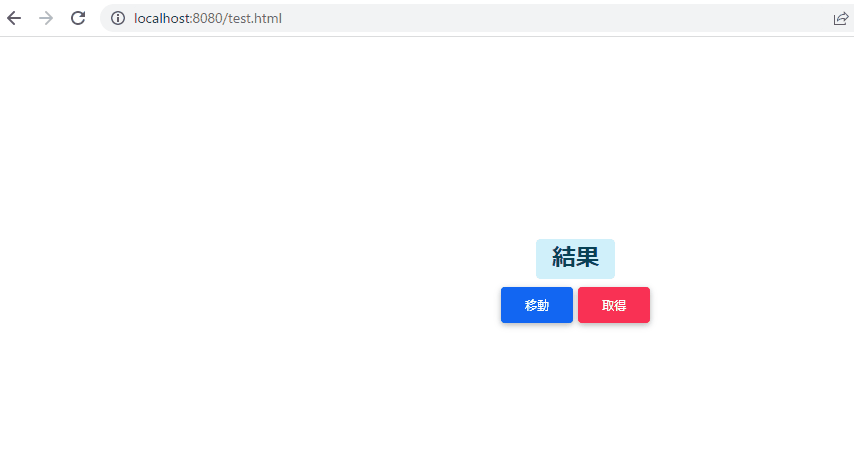
javascriptで、任意のURLからURLフラグメントと呼ばれる「#(シャープ)」は以降の値を取得するサンプルコードを記述してます。「lastIndexOf」と「slice」を使用して取得します。
環境
- OS windows11 pro 64bit
- ブラウザ chrome 108.0.5359.125
「#(シャープ)」以降の値を取得
「#(シャープ)」以降の値を取得するには、
1. 「lastIndexOf」で指定した文字列の最後インデックスを取得
2. 「slice」で「#」を除いて取得
の手順で可能です。
const url = 'https://mebee.info/#foo';
const last = url.lastIndexOf('#'); // 指定した文字列の最後インデックスを取得
console.log(last); // 19
console.log(url.slice(last)); // #foo
const hash = url.slice(last + 1); // 「#」を除いて取得
console.log(hash); // 'foo'
「#(シャープ)」が存在しない場合は、「-1」が返ってくるので「URL」が全て取得されます。
const url = 'https://mebee.info/foo';
const last = url.lastIndexOf('#'); // 指定した文字列の最後インデックスを取得
console.log(last); // -1
const hash = url.slice(last + 1); // 「#」を除いて取得
console.log(hash); // https://mebee.info/foo
実行させたくない場合は、以下のように条件を指定します。
const url = 'https://mebee.info/foo';
const last = url.lastIndexOf('#'); // 指定した文字列の最後インデックスを取得
console.log(last); // -1
if(last !== -1){
const hash = url.slice(last + 1); // 実行されない
console.log(hash); // 実行されない
}
location.hash
自信のURLであれば「location.hash」を使用して取得することも可能です。
// 自信のURLが「http://localhost:8080/sample.html#foo」だった場合
console.log(location.hash); // #foo
const hash = location.hash.slice(1); // 「#」を除いて取得
console.log(hash); // 'foo'
サンプルコード
以下は、
「移動」ボタンをクリックすると、アンカーを「#」で指定して移動して、「取得」ボタンでアンカー以降を取得して表示する
サンプルコードとなります。
※cssには「bootstrap material」を使用してます。関数はアロー関数で記述してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<!-- MDB -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/mdb-ui-kit/4.2.0/mdb.min.css" rel="stylesheet" />
</head>
<body>
<div class="container text-center w-75 mx-auto" style="margin-top:200px">
<h2><span class="badge badge-info">結果</span></h2>
<a href="#btn" class="btn btn-primary">移動</a>
<button id="btn" type="button" onclick="hoge()" class="btn btn-raised btn-danger">
取得
</button>
</div>
<script>
const hoge = () => {
const last = location.href.lastIndexOf('#'); // 自信のURLから指定した文字列の最後インデックスを取得
console.log(last);
if (last !== -1) document.getElementsByClassName("badge")[0].textContent = location.href.slice(last + 1);
}
</script>
</body>
</html>
表示されていることが確認できます。
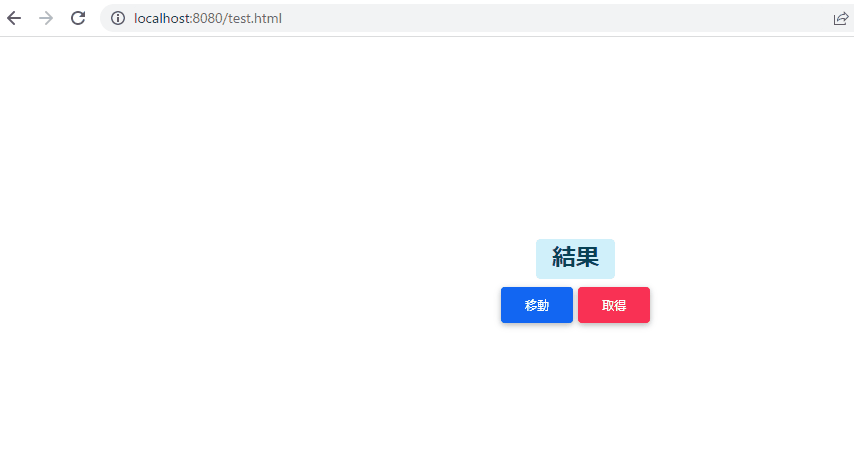
-
前の記事
mongoDB mongoシェル上でファイルのMD5値を取得する 2022.12.26
-
次の記事
kotlin 文字列から指定した文字列の位置を取得する 2022.12.27
コメントを書く