javascript リンククリックでボタンを非活性から活性化させる
- 作成日 2021.04.03
- 更新日 2022.08.12
- javascript
- javascript
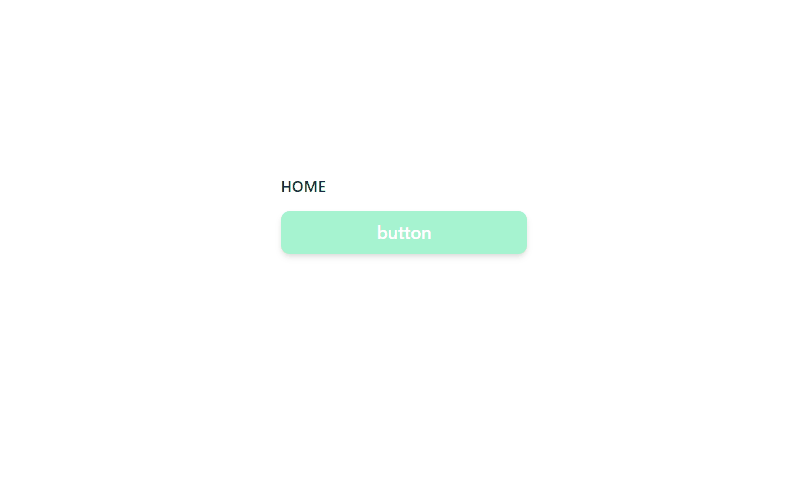
javascriptで、リンククリックでボタンを非活性から活性化させるサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 104.0.5112.81
ボタンを非活性
「button」を非活性化させるには「disabled」を使用します。
<input id="btn" type="button" value="ボタン" disabled>
リンククリックで活性化させるには、リンククリック時のイベントで、この「disabled」を取り除きます。
<a href="javascript:hoge();">mebee</a>
<input id="btn" type="button" value="ボタン" disabled>
<script>
'use strict';
function hoge(){
document.getElementById('btn').removeAttribute("disabled");
}
</script>
実行結果をみると、リンククリックでボタンが活性化されていることが確認できます。
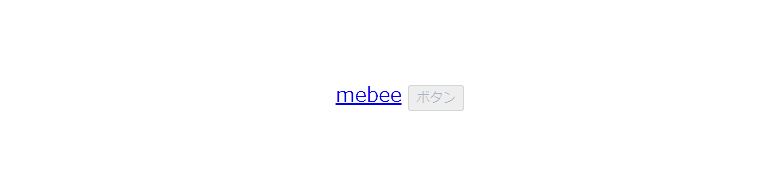
存在しない要素を指定
存在しない要素を指定すると、エラーが発生します。
<a href="javascript:hoge();">mebee</a>
<input id="btn" type="button" value="ボタン" disabled>
<script>
'use strict';
function hoge(){
document.getElementById('noelm').removeAttribute("disabled");
// Uncaught TypeError: Cannot read properties of null (reading 'removeAttribute')
}
</script>
エラーを回避するには、存在チェックをしてから使用します。
<a href="javascript:hoge();">mebee</a>
<input id="btn" type="button" value="ボタン" disabled>
<script>
'use strict';
function hoge(){
if(document.getElementById('noelm') !== null){
document.getElementById('noelm').removeAttribute("disabled");
}
}
</script>
クラス名を指定して非活性
「removeAttribute」は「HTMLCollection」なので「getElementsByClassName」で使用するとエラーとなります。
<a href="javascript:hoge();">mebee</a>
<input class="btn" type="button" value="ボタン" disabled>
<input class="btn" type="button" value="ボタン" disabled>
<input class="btn" type="button" value="ボタン" disabled>
<script>
'use strict';
function hoge(){
document.getElementsByClassName('btn').removeAttribute("disabled");
// Uncaught TypeError: document.getElementsByClassName(...).removeAttribute is not a function
}
</script>
「HTMLCollection」なので、ループ処理を使用して非活性させます。
<script>
'use strict';
function hoge() {
const elm = document.getElementsByClassName('btn');
if (0 < elm.length) {
[...elm].forEach(v =>
v.removeAttribute("disabled")
)
}
}
</script>
実行結果
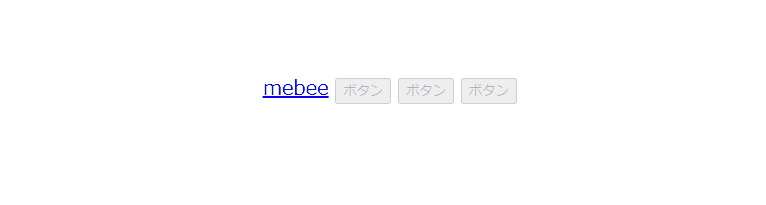
コードの簡潔化
また、以下のコードを、
document.getElementById('mebee').onclick = function(){
document.getElementById('btn').removeAttribute("disabled");
}
document.getElementByIdの省略とアロー関数を使用して、簡潔に記述することもできます。
mebee.onclick = () => {
btn.removeAttribute("disabled");
}
サンプルコード
以下は、
リンク「home」をクリックすると、「button」が活性化するだけの
サンプルコードとなります。
※cssには「tailwind」を使用して、アロー関数で関数は定義してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css" rel="stylesheet">
</head>
<script>
const hoge = () => {
btn.removeAttribute("disabled");
// cssも変更
btn.className = "py-2 px-4 bg-green-800 text-white font-semibold rounded-lg shadow-md";
}
window.onload = () => {
mebee.onclick = () => { hoge() };
}
</script>
<body>
<div class="container mx-auto my-56 w-64 px-4">
<div id="sample" class="flex flex-col justify-center">
<a id="mebee" href="https://mebee.info" class="mb-3 capitalize font-medium text-sm hover:text-teal-600 transition ease-in-out duration-500" target="_blank">
HOME
</a>
<button id="btn"
class="py-2 px-4 bg-green-200 text-white font-semibold rounded-lg shadow-md" disabled>
button
</button>
</div>
</div>
</body>
</html>
ボタンが活性化されたことが確認できます。
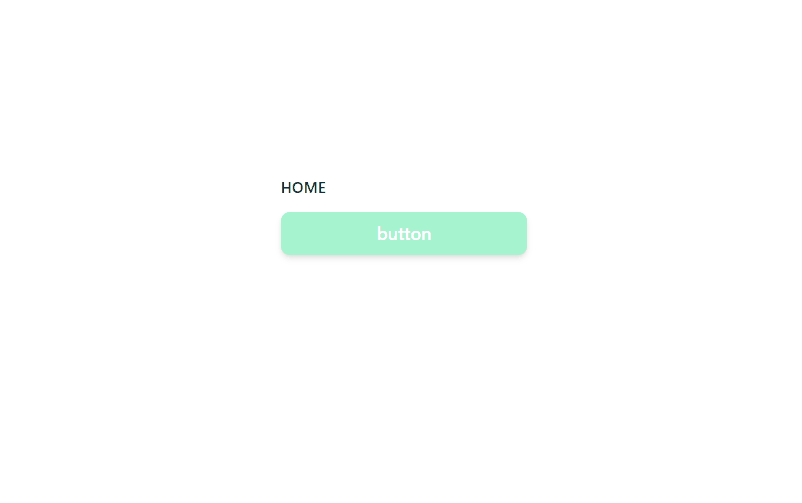
-
前の記事
rails6 バリデーション重複チェックを大文字・小文字を区別せずにチェックする 2021.04.03
-
次の記事
Pop!_OSに最新バージョンの「docker」と「docker compose」をインストールする 2021.04.03
コメントを書く