javascript ハイフンありなしを分けて郵便番号チェックを行う
- 作成日 2022.07.16
- javascript
- javascript
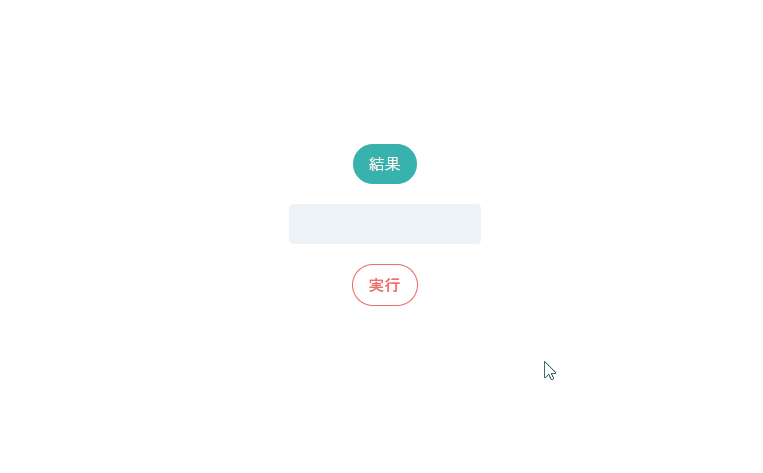
javascriptで、ハイフンありなしを分けて郵便番号チェックを行うサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 103.0.5060.114
正規表現
正規表現を用いると、郵便番号である妥当性を判定することが可能です。
ハイフンなしの場合
const num1 = "1001234";
const num2 = "10012345";
const num3 = "100-1234";
const num4 = "10-1234";
const regexp = /^\d{7}$/;
console.log(
regexp.test(num1) // true
);
console.log(
regexp.test(num2) // false
);
console.log(
regexp.test(num3) // false
);
console.log(
regexp.test(num4) // false
);
ハイフンありの場合
const num1 = "1001234";
const num2 = "10012345";
const num3 = "100-1234";
const num4 = "10-1234";
const regexp = /^\d{3}-\d{4}$/;
console.log(
regexp.test(num1) // false
);
console.log(
regexp.test(num2) // false
);
console.log(
regexp.test(num3) // true
);
console.log(
regexp.test(num4) // false
);
ハイフンありとなし両方
const num1 = "1001234";
const num2 = "10012345";
const num3 = "100-1234";
const num4 = "10-1234";
const regexp = /^\d{3}-?\d{4}$/;
console.log(
regexp.test(num1) // true
);
console.log(
regexp.test(num2) // false
);
console.log(
regexp.test(num3) // true
);
console.log(
regexp.test(num4) // false
);
サンプルコード
以下は、
「実行」ボタンをクリックすると、フォームから取得した郵便番号の妥当性を検証した結果を表示する
サンプルコードとなります。
※cssには「tailwind」を使用してます。関数はアロー化してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
</head>
<script>
const hoge = () => {
const regexp = /^\d{3}-?\d{4}$/;
// 結果を表示
result.innerHTML = regexp.test(num.value);
}
window.onload = () => {
// クリックイベントを登録
btn.onclick = () => { hoge() }; // document.getElementById('btn');を省略
}
</script>
<body>
<div class="container mx-auto my-56 w-56 px-4">
<div class="flex justify-center">
<p id="result" class="bg-teal-500 text-white py-2 px-4 rounded-full mt-4">結果</p>
</div>
<input
class="mt-5 bg-gray-200 appearance-none border-2 border-gray-200 rounded w-full py-2 px-4 text-gray-700 leading-tight focus:outline-none focus:bg-white focus:border-gray-500"
id="num" type="text">
<div class="flex justify-center">
<button id="btn" type="button"
class="mt-5 bg-transparent border border-red-500 hover:border-red-300 text-red-500 hover:text-red-300 font-bold py-2 px-4 rounded-full">
実行
</button>
</div>
</div>
</body>
</html>
妥当性が検証されていることが確認できます。
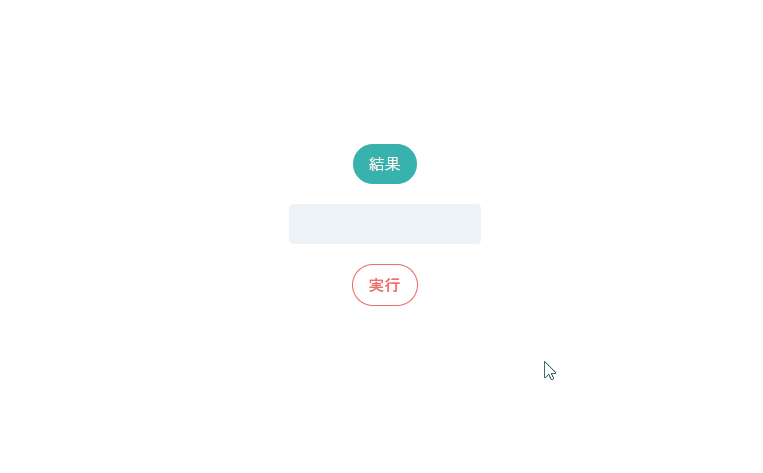
-
前の記事
VSCODE rubyで自動的にendを挿入する 2022.07.16
-
次の記事
javascript ランダムな配列の生成処理で「Array.apply」と「Array().fill」のパフォーマンスを計測する 2022.07.16
コメントを書く