javascript エラー「Uncaught ReferenceError: Cannot access ‘xxx’ before initialization」の解決方法
- 作成日 2022.04.17
- 更新日 2022.11.12
- javascript
- javascript
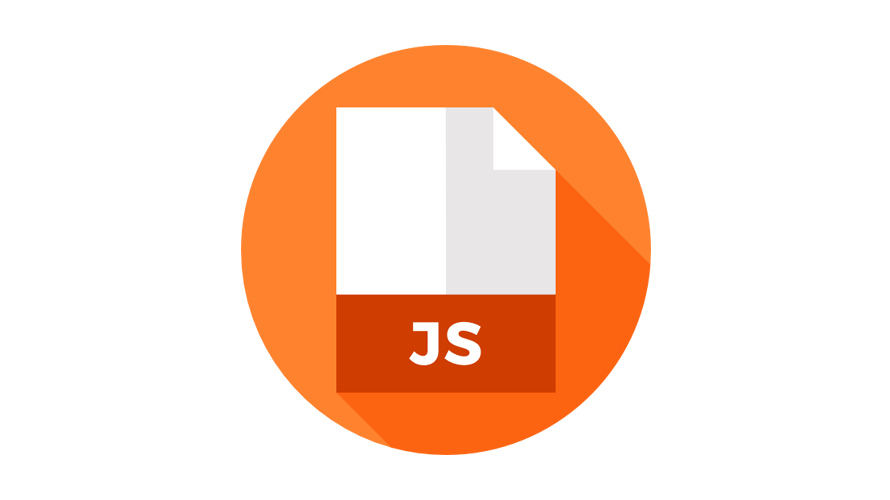
javascriptで、エラー「Uncaught ReferenceError: Cannot access ‘xxx’ before initialization」が発生した場合の原因と解決方法を記述してます。複数のパターンで発生します。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 107.0.5304.107
エラー内容
以下のコードを実行時に発生。
※その他のパターンは後述してます。
let x = x + 1;
エラーメッセージ
Uncaught ReferenceError: Cannot access 'x' before initialization
画像

firefox(バージョン106)では、以下のエラーとなります。
Uncaught ReferenceError: can't access lexical declaration 'x' before initialization
画像

原因
変数「x」を定義する前に利用しているため
解決方法
定義してから利用する
let x;
x = x + 1;
その他のパターン
三項演算子の括弧
三項演算子の括弧が原因で、同じパターンのエラーが発生します。
const n = "mebee"
( n.length > 5 ) ? console.log("5より大きい") : console.log("5以下")
// Uncaught ReferenceError: Cannot access 'n' before initialization
firefox106では、以下のエラーとなります。
Uncaught ReferenceError: can't access lexical declaration 'n' before initialization
これは「;」がないため、以下のように同じステートメントと認識されるためです。
const n = "mebee"( n.length > 5 ) ? console.log("5より大きい") : console.log("5以下")
解決方法は「;」を使用するか、三項演算子の「()」を外します。
const n = "mebee";
( n.length > 5 ) ? console.log("5より大きい") : console.log("5以下")
or
const n = "mebee"
n.length > 5 ? console.log("5より大きい") : console.log("5以下")
無名関数
無名関数を使用して定義する前に、実行しようとするとパターンのエラーが発生します。
foo(); // Uncaught ReferenceError: Cannot access 'foo' before initialization
const foo = function() {
console.log("sample");
}
firefox106では、以下エラーになります。
Uncaught ReferenceError: can't access lexical declaration 'foo' before initialization
無名関数の場合は、先に定義する必要があるため、解決方法は先に定義するか、関数宣言を使用します。
const foo = function() {
console.log("sample");
}
foo(); // sample
or
foo(); // sample
function foo(){
console.log("sample");
}
ちなみに、以下のように関数内で関数を呼び出しても同じです。
function fn1() {
fn2();
};
fn1(); // Uncaught ReferenceError: Cannot access 'fn2' before initialization
const fn2 = function () {
console.log( "sample" );
}
アロー関数
アロー関数でも使用して定義する前に、実行しようとするとパターンのエラーが発生します。
foo(); // Uncaught ReferenceError: Cannot access 'foo' before initialization
const foo = () => {
console.log("sample");
}
解決方法は、無名関数の場合と同じで先に記述するか関数宣言を使用します。
const foo = () => {
console.log("sample");
}
foo(); // sample
or
foo(); // sample
function foo(){
console.log("sample");
}
-
前の記事
Linux topコマンドの結果をファイルに書き出す 2022.04.16
-
次の記事
Linux treeコマンド実行時に隠しファイルも表示する 2022.04.17
コメントを書く