ASP.NET core 6 DBからモデルを生成する
- 作成日 2022.01.23
- 更新日 2022.02.27
- ASP.NET Core
- ASP.NET Core
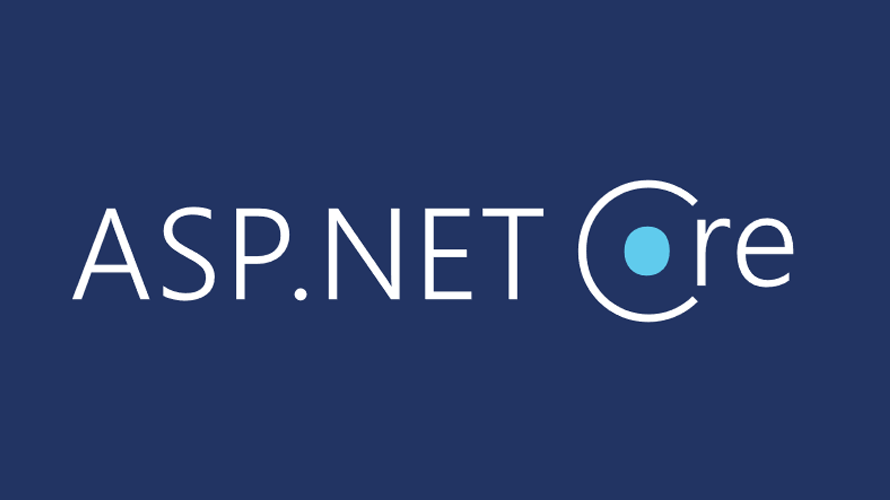
ASP.NET core 6で、DBからモデルを生成する手順を記述してます。.NETのバージョンは6を使用してます。
環境
- OS windows10 pro
- IDE Visual Studio 2022
- .NET 6
- Sql Server 2019
プロジェクト作成
ここでは、「Blazor Server アプリ」を選択してプロジェクトを作成してます。
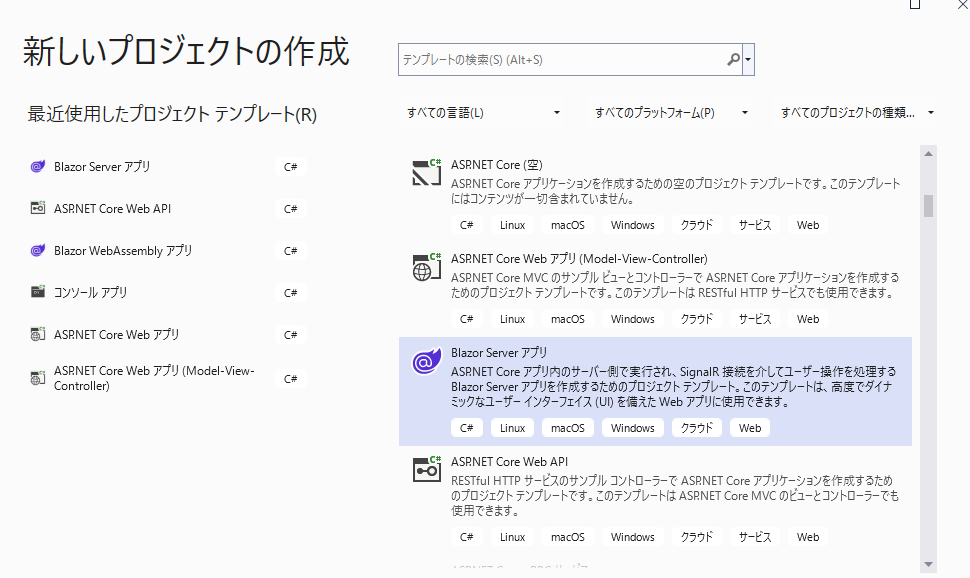
任意の名前でプロジェクトを作成します。
※ここでは「testBla」という名前で作成してます。
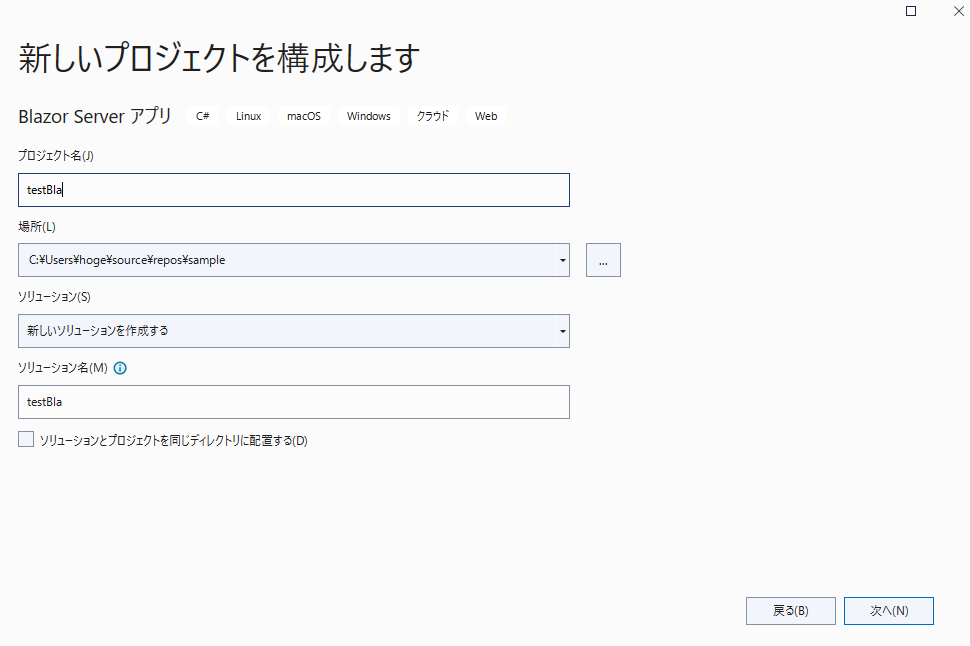
「.NET6.0」を選択して「作成」ボタンを押下します。
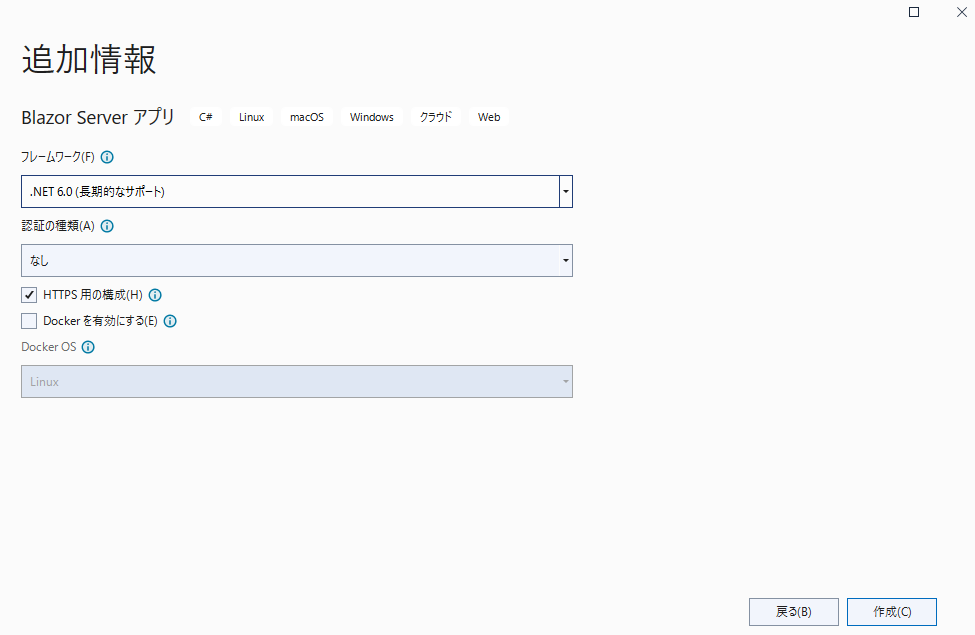
使用するDB
今回、接続するDBの種類は「sql server」で、以下のDB「hoge」内にあるテーブル「member」からモデルを作成します。
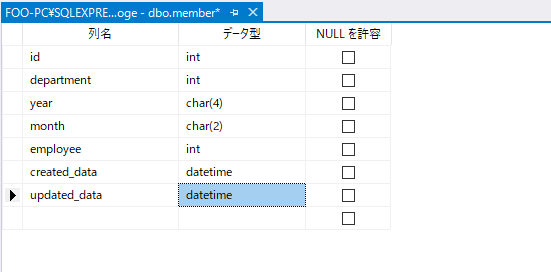
パッケージ追加
事前にパッケージを追加しておきます。「パッケージ マネージャー コンソール」を開きます。
「ツール」 > 「NuGet パッケージ マネージャー」 > 「パッケージ マネージャー コンソール」を選択します。
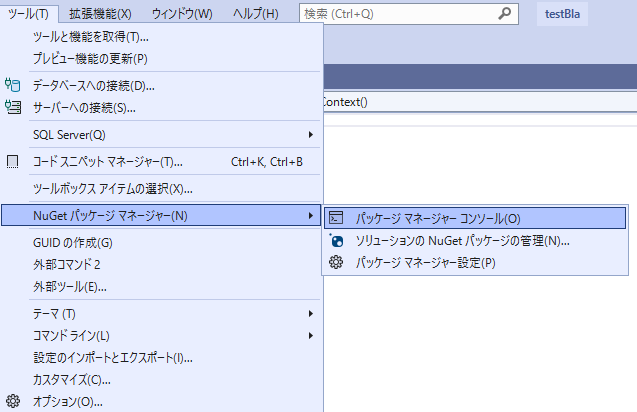
以下の2つのパッケージを追加しておきます。
PM> Install-Package -ProjectName testBla -Id Microsoft.EntityFrameworkCore.SqlServer
PM> Install-Package -ProjectName testBla -Id Microsoft.EntityFrameworkCore.Tools
モデル作成
パッケージ マネージャー コンソールから、モデルを作成します。
DBへの接続情報は以下となります。
Sql server : 192.168.xxx.xxx
Database : hoge
user : sa
password : password
「Scaffold-DbContext」を使用して作成します。
PM> Scaffold-DbContext -Provider Microsoft.EntityFrameworkCore.SqlServer -Connection "Data Source=192.168.xxx.xxx;Database=hoge;user id=sa;password=password" -f -OutputDir "Models" -Context "sampleDbContext" -UseDatabaseNames -DataAnnotations
「Build succeeded.」と表示されれば作成完了です。

項目 | 内容 | ここでの設定 |
---|---|---|
Provider | Microsoft.EntityFrameworkCore.SqlServer | Microsoft.EntityFrameworkCore.SqlServer “Data |
Connection | DBに接続するための接続文字列 | Source=192.168.xxx.xxx;Database=hoge;user id=sa;password=password |
f | 強制的に上書き | |
OutputDir | モデルを出力するパス | Models |
Context | コンテキストクラス名 | sampleDbContext |
UseDatabaseNames | データベースのテーブル名をクラス名にする | |
DataAnnotations | DataAnnotation属性の自動付与 |
モデル確認
「Models」配下に、作成されていることが確認できます。
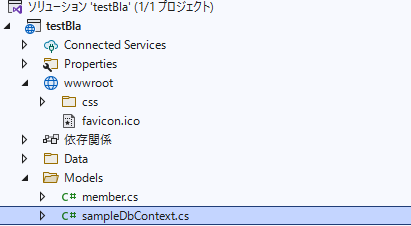
「member.cs」
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using Microsoft.EntityFrameworkCore;
namespace testBla.Models
{
[Keyless]
[Table("member")]
public partial class member
{
public int id { get; set; }
public int department { get; set; }
[StringLength(4)]
[Unicode(false)]
public string year { get; set; } = null!;
[StringLength(2)]
[Unicode(false)]
public string month { get; set; } = null!;
public int employee { get; set; }
[Column(TypeName = "datetime")]
public DateTime created_data { get; set; }
[Column(TypeName = "datetime")]
public DateTime updated_data { get; set; }
}
}
「sampleDbContext.cs」にはDBへの接続情報が記載されている「OnConfiguring」メソッドがあり警告がでます。
これは、削除して「appsettings.json」などに接続情報を記載してください。
using System;
using System.Collections.Generic;
using Microsoft.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore.Metadata;
namespace testBla.Models
{
public partial class sampleDbContext : DbContext
{
public sampleDbContext()
{
}
public sampleDbContext(DbContextOptions<sampleDbContext> options)
: base(options)
{
}
public virtual DbSet<member> members { get; set; } = null!;
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
if (!optionsBuilder.IsConfigured)
{
#warning To protect potentially sensitive information in your connection string, you should move it out of source code. You can avoid scaffolding the connection string by using the Name= syntax to read it from configuration - see https://go.microsoft.com/fwlink/?linkid=2131148. For more guidance on storing connection strings, see http://go.microsoft.com/fwlink/?LinkId=723263.
optionsBuilder.UseSqlServer("Data Source=192.168.xxx.xxx;Database=hoge;user id=sa;password=password");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<member>(entity =>
{
entity.Property(e => e.created_data).HasDefaultValueSql("(getdate())");
entity.Property(e => e.id).ValueGeneratedOnAdd();
entity.Property(e => e.month).IsFixedLength();
entity.Property(e => e.updated_data).HasDefaultValueSql("(getdate())");
entity.Property(e => e.year).IsFixedLength();
});
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
-
前の記事
Hasura REST APIのエンドポイントを作成する 2022.01.23
-
次の記事
javascript lodashを使ってメソッドチェーンで配列の値を反転させる 2022.01.24
コメントを書く