VSCODEでASP.NET Coreを起動する
- 作成日 2021.06.05
- ASP.NET Core vscode
- ASP.NET Core, vscode
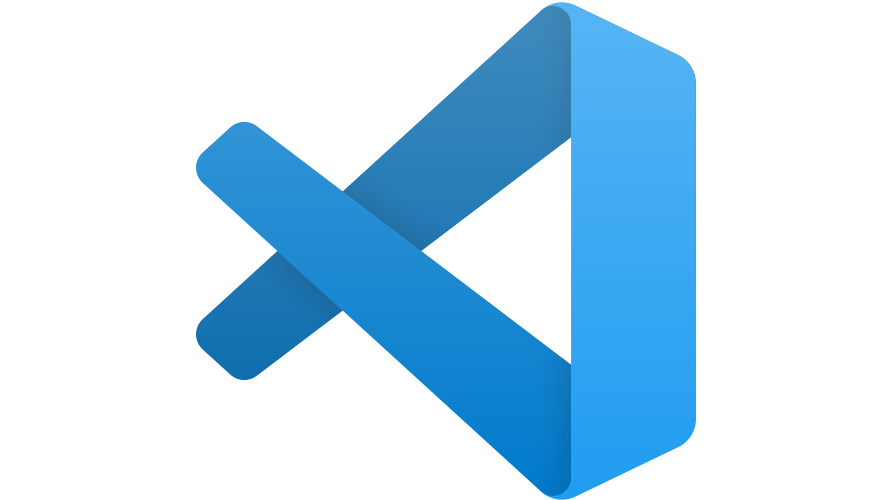
VSCODEでASP.NET Coreを起動するまでの手順を記述してます。
環境
- OS windows10 pro 64bit
- VSCODE 1.56.2
- .NET Core 3.1.409
.NET Coreインストール
こちらのサイトからインストーラーをダウンロードして、インストール可能です。
※なぜかchromeだとうまくいかなかったのでfirefoxを使用しました。
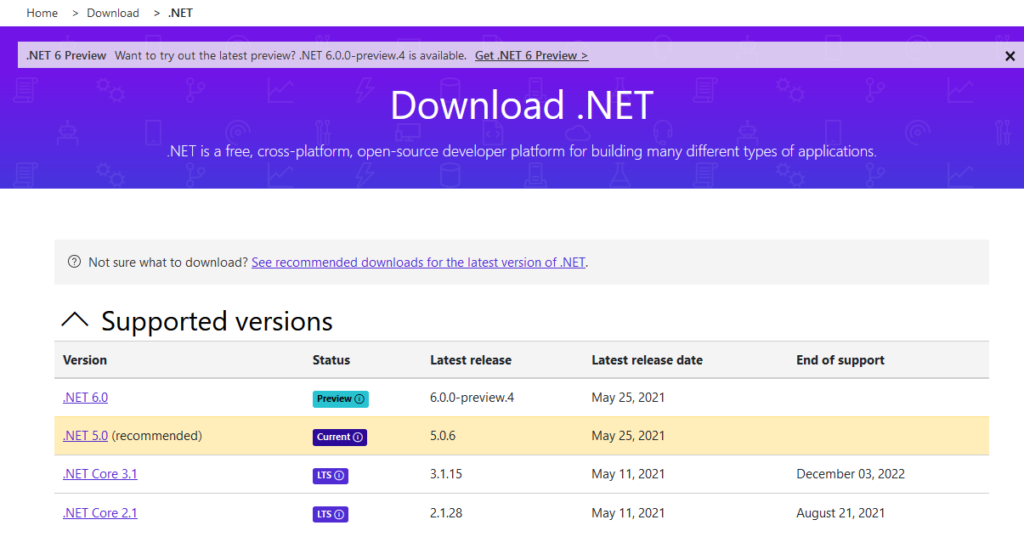
extension追加
とりあえず、以下のextensionだけ追加します。
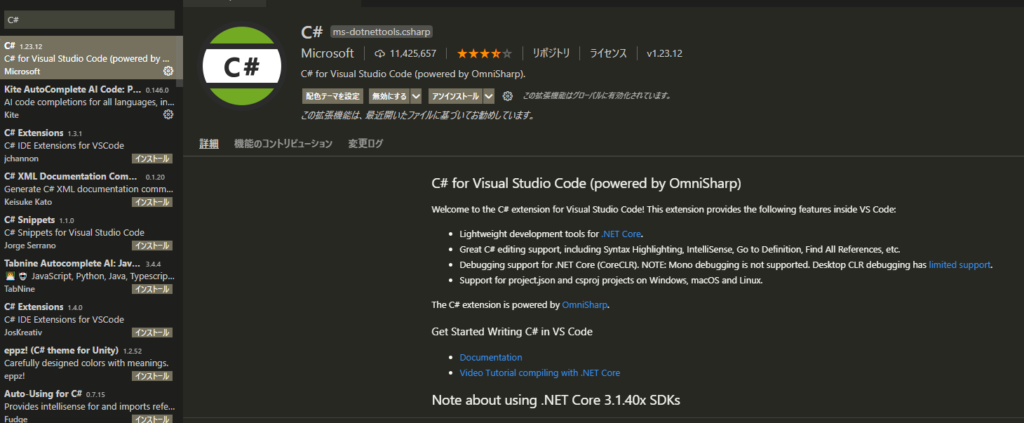
ASP.NET Coreプロジェクト作成
任意のフォルダを作成して、移動します。
※ここでは「coretest」というフォルダを作成してます。
## C直下に移動
cd /
## coretestというフォルダを作成
mkdir coretest
## coretestに移動
cd coretest
sampleApiというwebapiプロジェクトを作成します。
dotnet new webapi -o sampleApi
作成したら、移動します。
cd sampleApi
後はVSCODEからフォルダを開いて、
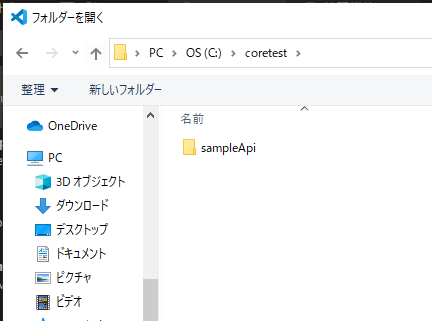
httpsでアクセスするため証明書をインストールしておきます。
dotnet dev-certs https --trust
「はい」を選択します。
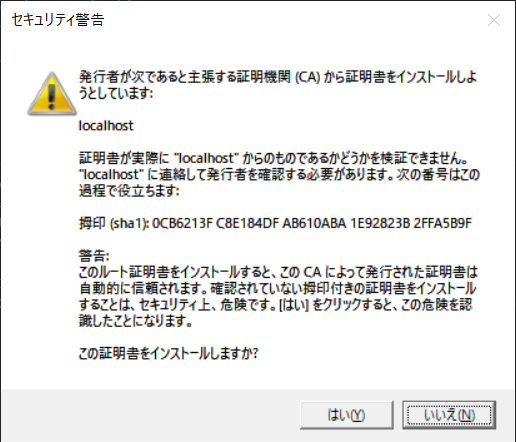
「ctrl」+「F5」か、下図の「緑の再生ボタン」から起動が可能です。
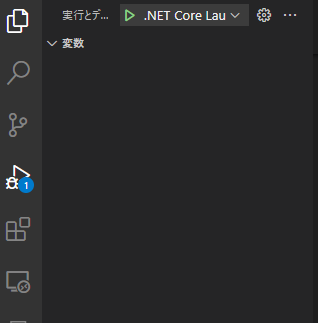
ブラウザから http://プライベートIP or サーバーアドレス:port/WeatherForecast にアクセスすると、JSONが表示されます。
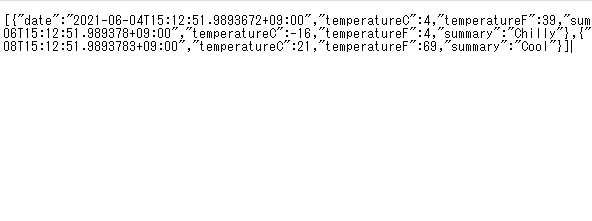
Api作成
webapiを作成してみます。「Model」フォルダを作成して「BookItem.cs」というファイルを作成します。

「BookItem.cs」を以下のように編集します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace Book.Models
{
public class BookItem
{
public int Id { get; set; }
public string Name { get; set; }
}
}
次に「Controllers」配下に「BookController.cs」を作成します。
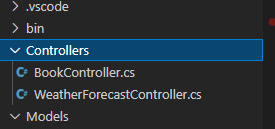
「BookController.cs」を以下のように編集します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Book.Models;
namespace Book.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class BookController : ControllerBase
{
private static List<BookItem> items = new List<BookItem>() {
new BookItem() { Id = 1, Name = @"a" },
new BookItem() { Id = 2, Name = @"b" },
new BookItem() { Id = 3, Name = @"c" },
};
[HttpGet]
public ActionResult<List<BookItem>> GetAll()
=> items;
[HttpGet("{id}", Name = "BookItem")]
public ActionResult<BookItem> GetById(int id)
{
var item = items.Find(i => i.Id == id);
if (item == null)
return NotFound();
return item;
}
}
}
起動して、ブラウザから https://localhost:5001/api/book にアクセスすると「JSON」が表示されていることが確認できます。
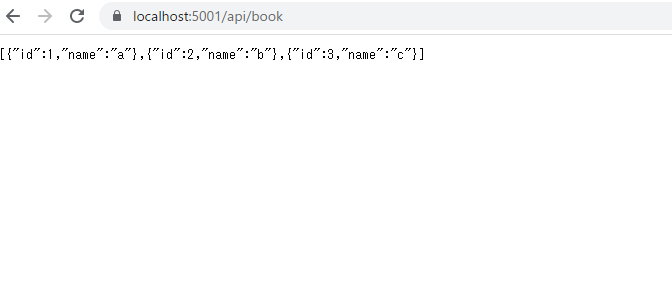
-
前の記事
javascript readonly属性の状態を取得して変更する 2021.06.05
-
次の記事
mysql データベースのサイズを一覧で確認する 2021.06.06
コメントを書く