ASP.NET Core DBと接続してAPIを実装する
- 作成日 2021.02.08
- 更新日 2021.02.12
- ASP.NET Core Sql Server
- ASP.NET Core
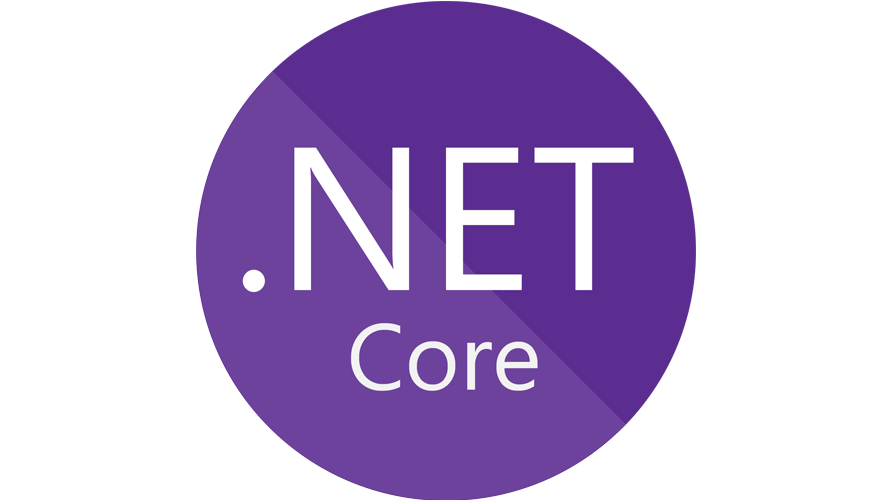
ASP.NET CoreでDBと接続してAPIを実装するまでの手順を記述してます。.NET Coreのバージョンは3.1を使用してます。
環境
- OS windows10 pro
- IDE Visual Studio 2019
- .NET Core 3.1
- SQL Server2019 Express
プロジェクト作成
Visual Studio 2019からプロジェクトを作成します。
ASP.NET Core Webアプリケーションを選択します。
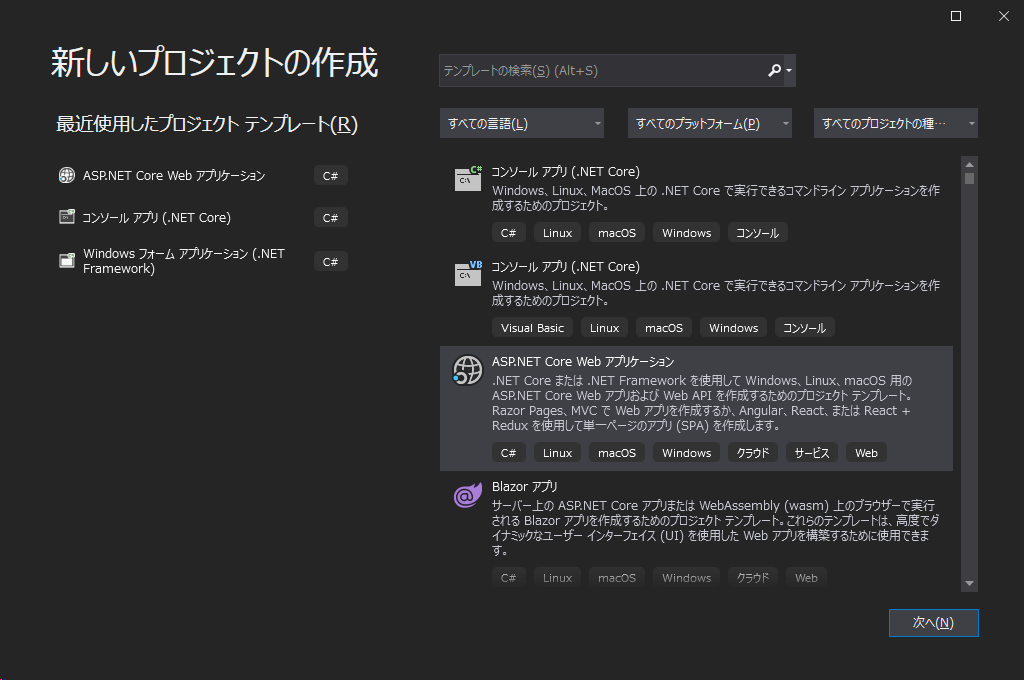
任意の名前でプロジェクトを作成します。
※ここでは「sample」として作成してます。
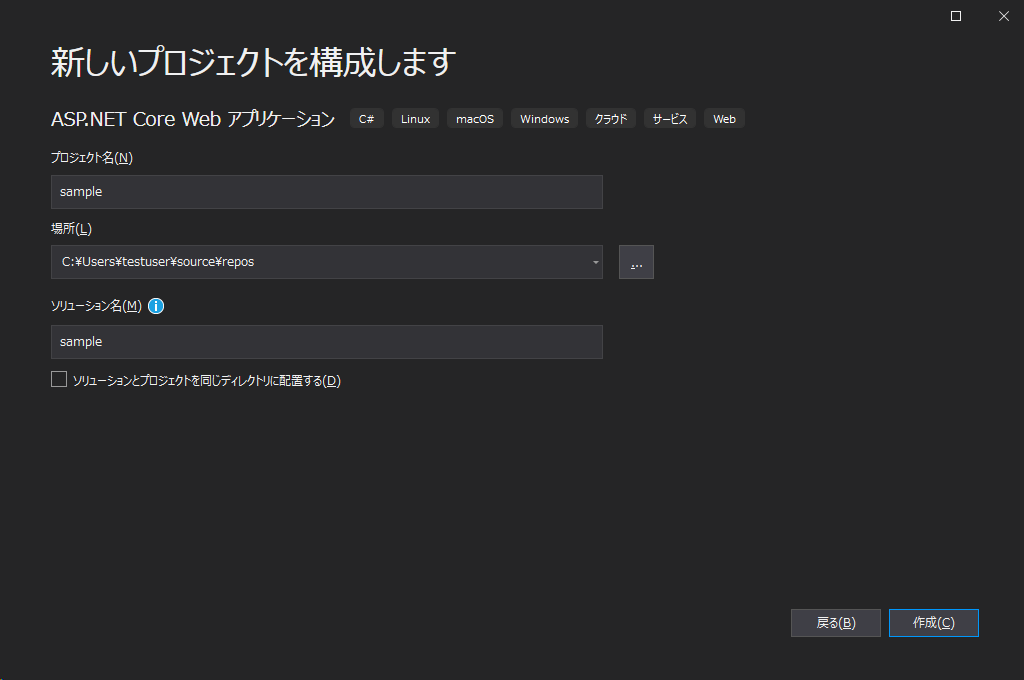
「API」を選択します。
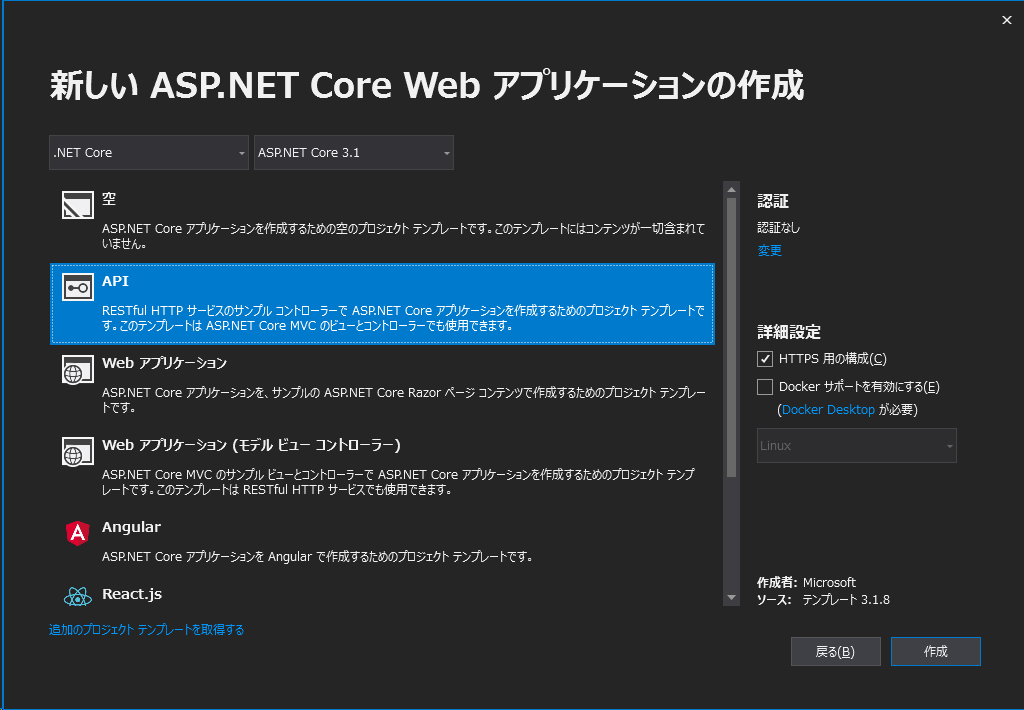
プロジェクトが作成されます。
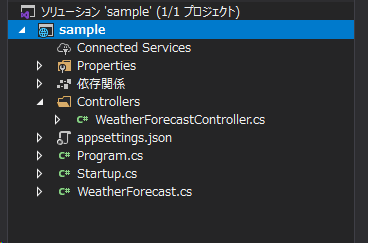
パッケージ追加
NuGetパッケージから以下の3つをインストールしておきます。
- Microsoft.EntityFrameworkCore
- Microsoft.EntityFrameworkCore.SqlServer
- Microsoft.EntityFrameworkCore.Tools
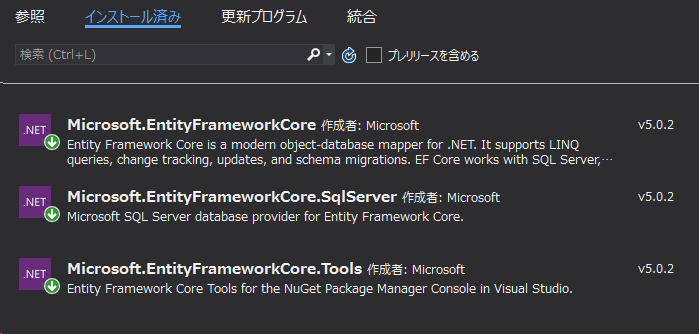
接続文字列追加
「appsettings.json」にSQL Serverとの接続文字列を追加しておきます。
サーバー localhost
DB名 sample
ユーザー名 sa
パスワード password
で追加してます。
"ConnectionStrings": {
"DefaultConnection": "Server=localhost;Database=sample;User ID=sa;Password=password;"
},
Entitiesクラス作成
まずはプロジェクト配下に「Entities」フォルダを作成して、そこに「Parson.cs」を作成します。
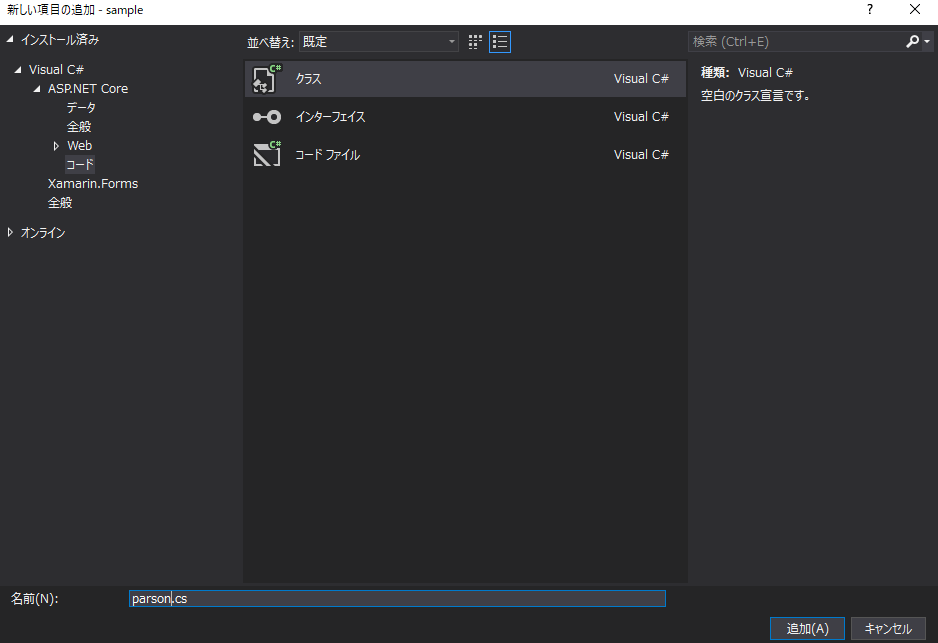

「Parson.cs」を以下のように記述します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace sample.Entities
{
public class Parson
{
public int Id { get; set; }
public string name { get; set; }
}
}
マッピング
マッピングを行うため、プロジェクト配下に「Data」フォルダを作成して、そこに「ParsonContext.cs」を作成します。
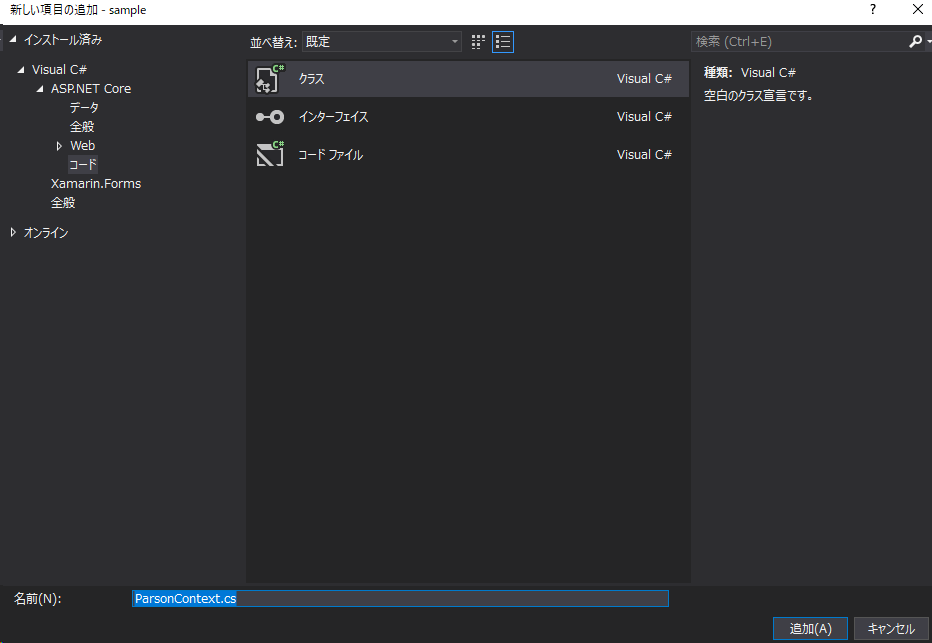

「ParsonContext.cs」を以下のように記述します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.EntityFrameworkCore;
using sample.Entities;
namespace sample.Data
{
public class ParsonContext : DbContext
{
public ParsonContext(DbContextOptions options) : base(options)
{
}
public DbSet<Parson> Parsons { get; set; }
}
}
Controller作成
「Controllers」フォルダ配下に、そこに「APIコントローラー」を選択して、「ParsonsController.cs」を作成します。
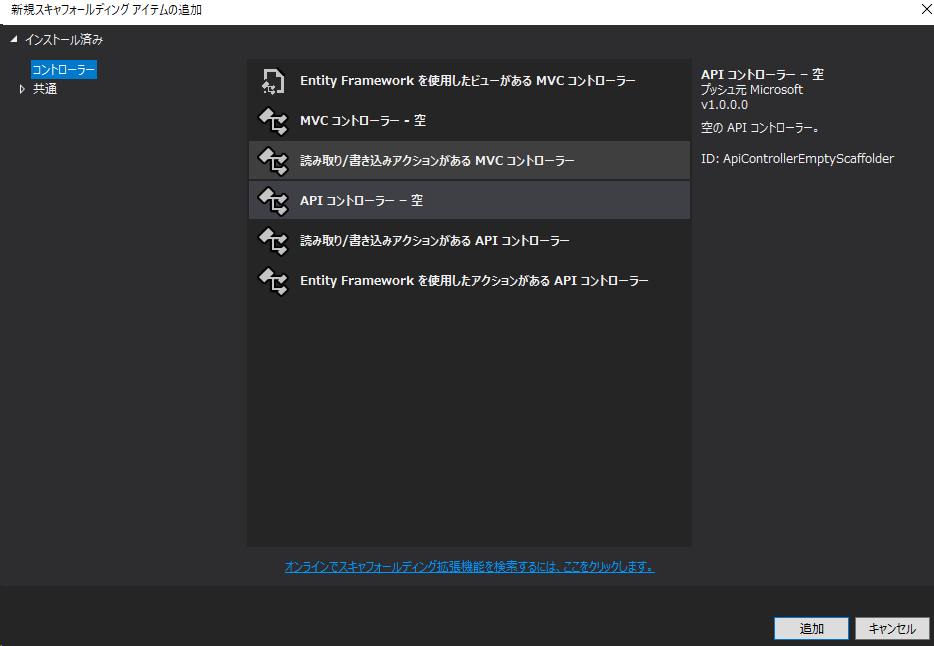

「ParsonsController.cs」を以下のように記述します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using sample.Data;
using sample.Entities;
namespace sample.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ParsonsController : ControllerBase
{
private readonly ParsonContext context;
public ParsonsController(ParsonContext context)
{
this.context = context;
}
[HttpGet]
public ActionResult<IEnumerable<Parson>> List()
{
var parsons = context.Parsons;
return parsons;
}
}
}
サービス登録
「Startup.cs」にサービスを登録します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.HttpsPolicy;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using sample.Data;
namespace sample
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ParsonContext>(options => options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_3_0);
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
}
}
Migration実行
パッケージマネージャーコンソールを開いて、Migrationを実行します。
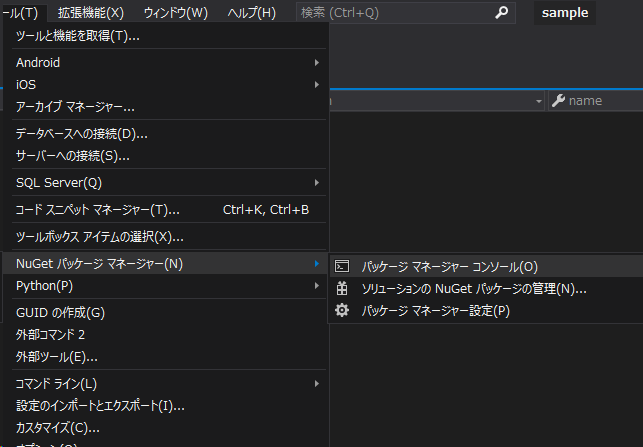
以下のコマンドを実行します。
Add-Migration Initial
「Migrations」フォルダ配下にマイグレーションファイルが作成されます。

テーブルを作成します。
Update-Database
テーブルが作成されていることが確認できます。
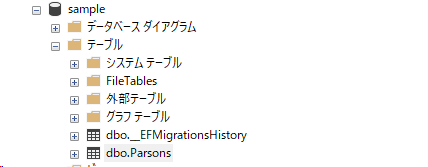
データ追加
データを追加するためinsert文を流します。
INSERT INTO Parsons (name) VALUES (N'sato');
INSERT INTO Parsons (name) VALUES (N'iwai');
API確認
デバックして「/api/parsons」にアクセスするとapiが取得できていることが確認できます。
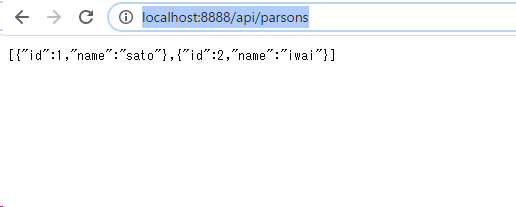
-
前の記事
React.js ライブラリ「react-finderselect」を使ってファインダーセレクトを実装する 2021.02.08
-
次の記事
javascript アロー関数でfilterを使う 2021.02.09
コメントを書く