ASP.NET Core Npgsqlのデータベースの接続情報を「appsettings.json」に記述する
- 作成日 2021.06.19
- ASP.NET Core
- ASP.NET
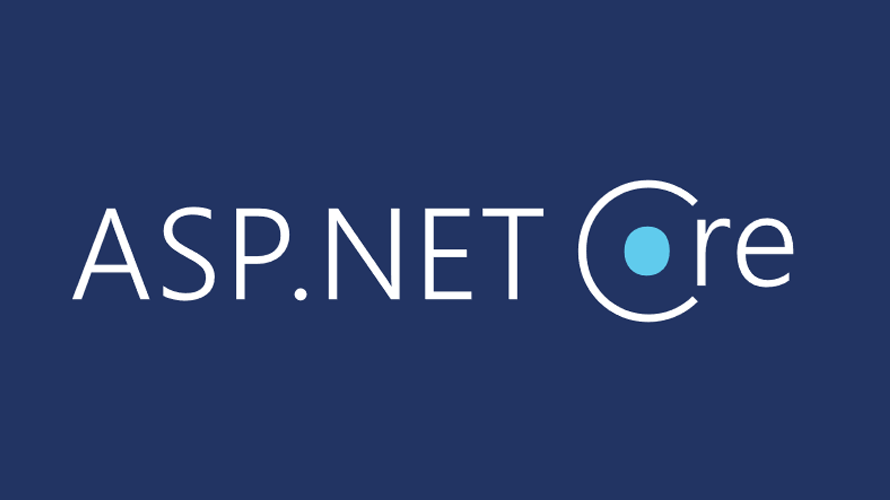
ASP.NET Coreで、Npgsqlのデータベースの接続情報を「appsettings.json」に記述するまでの手順を記述してます。
環境
- OS windows10 pro 64bit
- VSCODE 1.56.2
- postgres sql 13.3
- .NET Core 3.1.409
事前準備
ここではライブラリに「Npgsql」と「Dapper」を使用してます。
nugetで、どちらも追加してます。
nugetを追加したら、「F1」キーを押下して「NuGet Package Manager: Add Package」を選択して
「Npgsql」と「Dapper」と入力すれば、追加することができます。
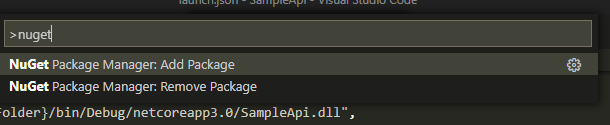
「.csproj」は以下ようになってます。
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>netcoreapp3.1</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Npgsql" Version="5.0.5"/>
<PackageReference Include="Dapper" Version="2.0.90"/>
</ItemGroup>
</Project>
また、ここで利用する「book」テーブルには、以下のデータが入ってます。
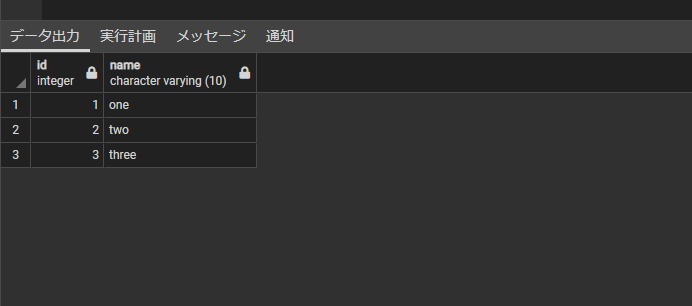
appsettings.json編集
DBへの接続情報である「ConnectionStrings」を「appsettings.json」に追加します。
{
"ConnectionStrings": {
"DefaultConnection": "Server=192.168.xxx.xxx;Port=5432;User Id=username;Password=password;Database=sample"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"
}
Services作成
Servicesフォルダを作成して「IBookService.cs」を作成します。
namespace Book.Services
{
public interface IBookService{
int CountBooks();
}
}
同様に「Services」配下に「BookService.cs」を作成します。
using System.Data;
using System.Linq;
using System.Collections.Generic;
using Dapper;
namespace Book.Services
{
public class BookService : IBookService{
private IDbConnection connection;
public BookService(IDbConnection connection)
{
this.connection = connection;
}
public int CountBooks(){
return connection.Query<int>("SELECT COUNT(*) FROM book").First();
}
}
}
Startup.cs編集
Startup.csに以下を追加します。
// 追加
using Npgsql;
using Book.Services;
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
// 追加
services.AddScoped<IDbConnection>(
provider=> new NpgsqlConnection(
Configuration.GetConnectionString("DefaultConnection")
)
);
// 追加
services.AddScoped<IBookService, BookService>();
}
Controller編集
後は、適当なControllerに以下を追加して利用します。
// 追加
using System.Data;
using Book.Services;
namespace Book.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class BookController : ControllerBase
{
// 追加
private IBookService service;
// 追加
public BookController(IBookService service)
{
this.service = service;
}
// 追加
public void CountBooks()
{
var count = service.CountBooks();
Console.WriteLine(count);
}
}
}
-
前の記事
C# テキストファイル内のデータの改行を削除して表示する 2021.06.19
-
次の記事
javascript lodashを使って配列からINDEX番号ごとにオブジェクトを生成する 2021.06.20
コメントを書く