javascript size属性の値を取得して変更する
- 作成日 2021.06.19
- 更新日 2022.09.07
- javascript
- javascript
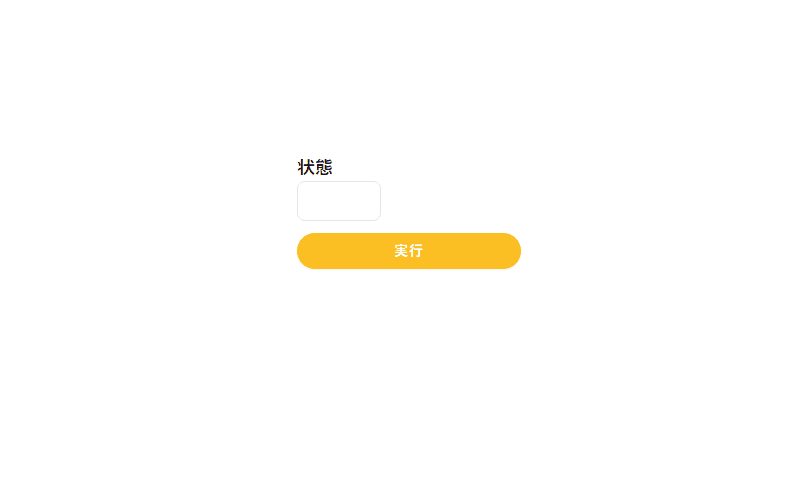
javascriptで、文字数でフォームの幅を指定できるsize属性の値を取得して変更するサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 104.0.5112.101
size属性 取得/変更
size属性を取得/変更するには、sizeプロパティを利用します。
<input id="hoge" type="text" name="hoge" size="5" />
<input id="btn" type="button" value="ボタン" />
<script>
'use strict';
document.getElementById('btn').onclick = function () {
const elm = document.getElementById('hoge')
// コンソールに出力
console.log(elm.size);
// 10に設定
elm.size = 10;
}
</script>
実行結果を確認すると、「size」属性の値が、コンソールに表示されて幅が変更されていることが確認できます。
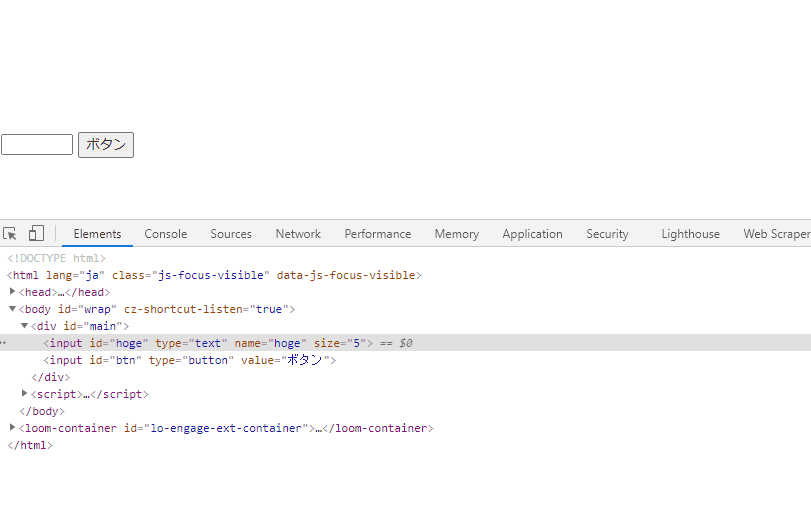
複数の要素を一括で取得/変更
例えば「class」名を指定して、一括で取得/変更する場合は以下のように「forEach」を使用します。
※「HTMLCollection」は配列に変更すると「forEach」が使用できます。
<input class="hoge" type="number" size="5"/>
<input class="hoge" type="number" size="5"/>
<input class="hoge" type="number" size="5"/>
<input id="btn" type="button" value="ボタン" />
<script>
'use strict';
document.getElementById('btn').onclick = function () {
const elm = document.getElementsByClassName('hoge');
[...elm].forEach(function (v) { v.size = 10 });
}
</script>
実行結果
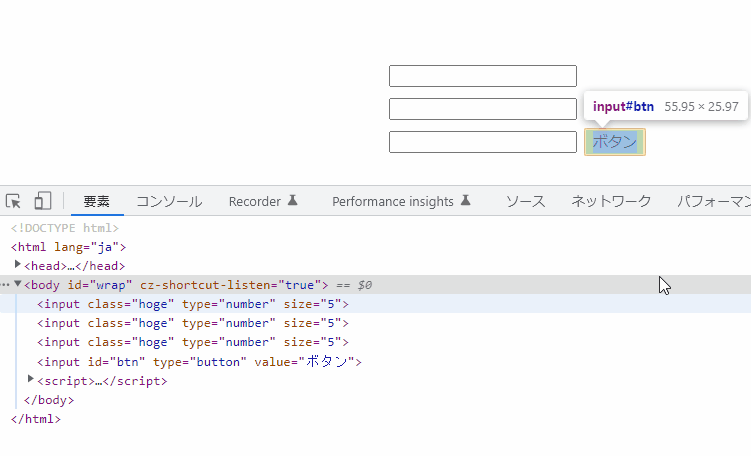
コードの簡略化
また、以下のコードを、
document.getElementById('btn').onclick = function () {
const elm = document.getElementById('hoge')
// コンソールに出力
console.log(elm.size);
// 10に設定
elm.size = 10;
}
アロー関数とdocument.getElementByIdを省略して、簡潔に記述することもできます。
btn.onclick = () =>{
// コンソールに出力
console.log(hoge.size);
// 10に設定
hoge.size = 10;
}
サンプルコード
以下は、
「実行」ボタンをクリックして、「size」属性の値を変更して表示するサンプルコードとなります。
※cssには「tailwind」を使用して、アロー関数で関数は定義してます。三項演算子も使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css" rel="stylesheet">
</head>
<script>
const hoge = () => {
foo.size == 5 ? foo.size = 15 : foo.size = 5;
disp.innerHTML = foo.size;
}
window.onload = () => {
btn.onclick = () => { hoge() };
}
</script>
<body>
<div class="container mx-auto my-56 w-64 px-4">
<div id="sample" class="flex flex-col justify-center">
<h2 id="disp" class="font-semibold text-lg mr-auto">状態</h2>
<div class="mb-3 md:space-y-2 w-full text-xs">
<input id="foo" size="5" class="appearance-none bg-grey-lighter text-grey-darker border border-grey-lighter rounded-lg h-10 px-4" type="text" />
</div>
<button id="btn" class="mb-2 md:mb-0 bg-yellow-400 px-5 py-2 text-sm shadow-sm font-medium tracking-wider text-white rounded-full hover:shadow-lg hover:bg-yellow-500">
実行
</button>
</div>
</div>
</body>
</html>
実行結果を確認すると、値が変更されて表示されていることが確認できます。
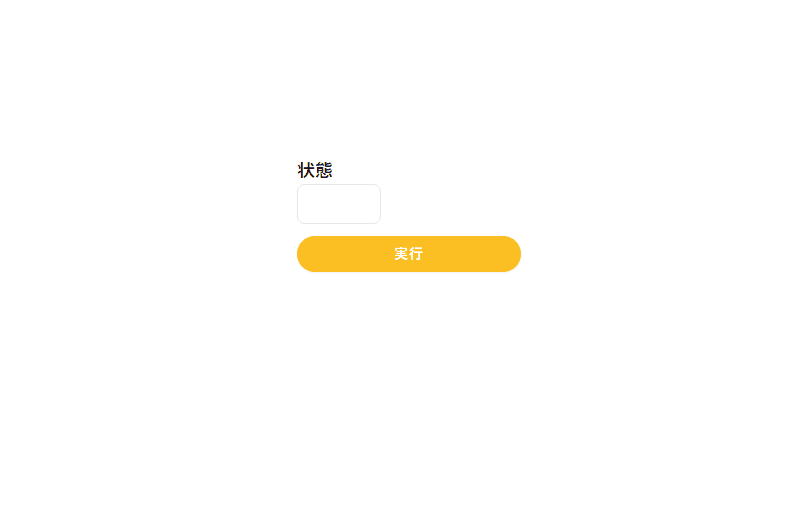
-
前の記事
C# CheckBoxのテキストの色を変更する 2021.06.19
-
次の記事
python numpyの配列をスライスする 2021.06.19
コメントを書く