javascript レンジ入力(input type=”range”)の値を取得する
- 作成日 2020.09.11
- 更新日 2022.06.21
- javascript
- javascript
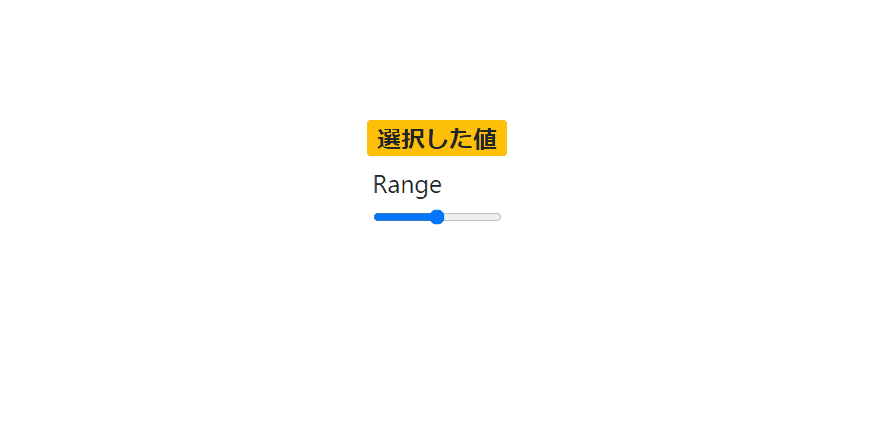
javascriptで、レンジ入力(input type=”range”)の値を取得するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 102.0.5005.115
取得方法
以下のコードで取得可能です。
<form name="frm">
<input type="range" id="range" min="0" max="100" value="20" name="hoge" class="bar">
</form>
<script>
// idから取得
console.log( document.getElementById("range").value ); // valueが20なので 20 が表示されます
// nameから取得
console.log( document.getElementsByName("hoge")[0].value ) // 20
// class名から取得
console.log( document.getElementsByClassName("bar")[0].value ) // 20
// formのidから取得
console.log( document.frm.range.value ) // 20
</script>
実行結果
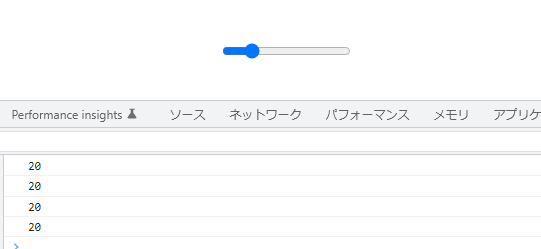
また、document.getElementByIdを省略して「id名」のみで記述することも可能です。
console.log( range.value );
サンプルコード
以下は、
レンジを変更するたびに、値を表示する
サンプルコードとなります。
※cssには「bootstrap」を使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity="sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin="anonymous">
</head>
<style>
.main {
margin: 0 auto;
margin-top: 200px;
display: flex;
flex-direction: column;
align-items: center;
font-size: 25px;
width: 800px;
}
</style>
<script>
window.onload = function () {
let hoge = document.getElementById("range");
// 選択した際のイベント取得
hoge.addEventListener('change', (e) => {
document.getElementsByClassName('badge-warning')[0].textContent = hoge.value;
});
}
</script>
<body>
<div class="main">
<h2><span class="badge badge-warning">選択した値</span></h2>
<form id="foo">
<div class="form-group">
<label for="formControlRange">Range</label>
<input type="range" class="form-control-range" id="range" min="0" max="100" value="50" >
</div>
</form>
</div>
</body>
</html>
取得されていることが確認できます。
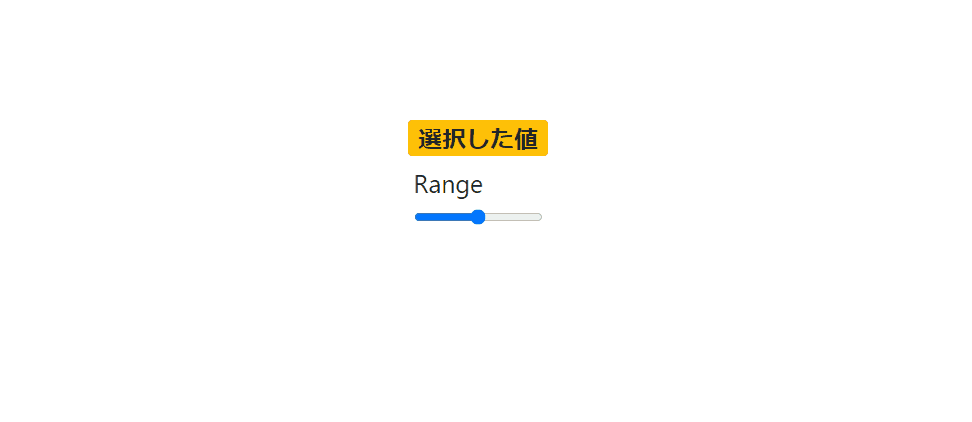
-
前の記事
Nuxt.js ライブラリ「vue-load-image」をインストールしてローダーを表示する 2020.09.10
-
次の記事
javascript 配列の値を全て削除する 2020.09.11
コメントを書く