javascript エラー「Uncaught TypeError: xxxxx.unshift is not a function」の解決方法
- 作成日 2022.10.30
- javascript
- javascript
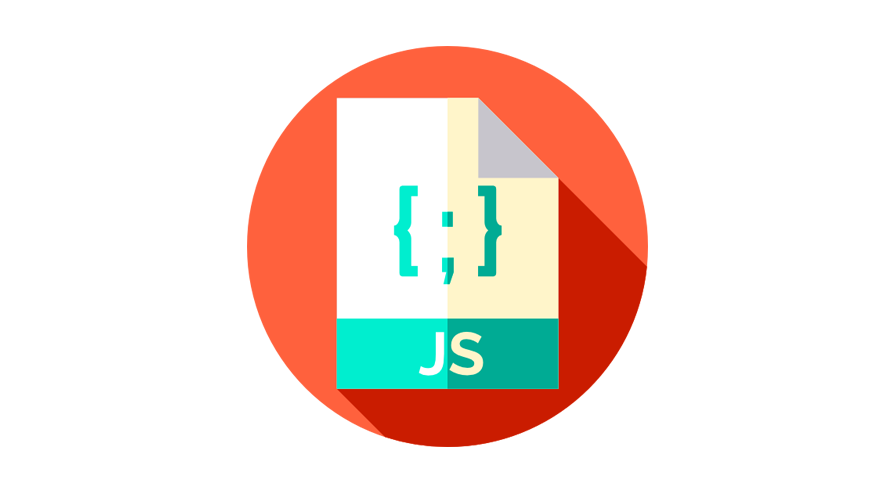
javascriptで、エラー「Uncaught TypeError: xxxxx.unshift is not a function」が発生した場合の原因と解決方法を記述してます。配列以外の値に、配列の初めに要素を追加する「unshift」を使用した際に発生します。「chrome」や「firefox」や「safari」の各ブラウザのエラーメッセージの画像もキャプチャしてます。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 106.0.5249.119
エラー内容
以下の、「オブジェクト」に対して「unshift」で先頭に値を追加しようとした際に発生。
let obj = { name:'mebee', age:25 };
obj.unshift({id: 1});
エラーメッセージ
Uncaught TypeError: obj.unshift is not a function
画像

firefox105の場合でも同じエラーが発生します。
Uncaught TypeError: obj.unshift is not a function
画像

safari15.5では、以下のエラーとなります。
TypeError: obj.unshift is not a function. (In 'obj.unshift({id: 1})', 'obj.unshift' is undefined)
画像

原因
「unshift」は、配列以外には使用できないため。以下は配列に「unshift」を使用した例となります。
const arr = ['aaa', 'bbb'];
const num = arr.unshift('ccc');
console.log(arr);
// ['ccc', 'aaa', 'bbb']
console.log(num);
// 3 ← 追加後の要素の数
解決方法
「Object.entries」などで「配列」に変換してから、再度オブジェクトに戻す
let obj = { name:'mebee', age:25 };
// Object.entriesで配列化
let arr = Object.entries(obj);
console.log(arr); // [['name', 'mebee'],['age', 25]]
// 先頭に追加
arr.unshift(['id',1]);
console.log(arr);// [['id',1],['name', 'mebee'],['age', 25]]
// 配列からオブジェクトに戻す
obj = arr.reduce((obj, [key, value]) => ({...obj, [key]: value}), {});
console.log(obj); // {id: 1, name: 'mebee', age: 25}
または、「配列」であるかを判定してから使用します。
let obj = { name:'mebee', age:25 };
const result = Array.isArray(obj) ? obj.unshift({id: 1}) : '配列ではありません';
console.log( result );
// 配列ではありません
-
前の記事
GAS アクティブなスプレッドシートを削除する 2022.10.29
-
次の記事
mongoDB エラー「ReferenceError: xxx is not defined (Are you trying to run a script written for the legacy shell? Try running snippet install mongocompat)」が発生した場合の対処法 2022.10.30
コメントを書く