javascript フォームの値を削除する
- 作成日 2020.08.24
- 更新日 2022.06.06
- javascript
- javascript
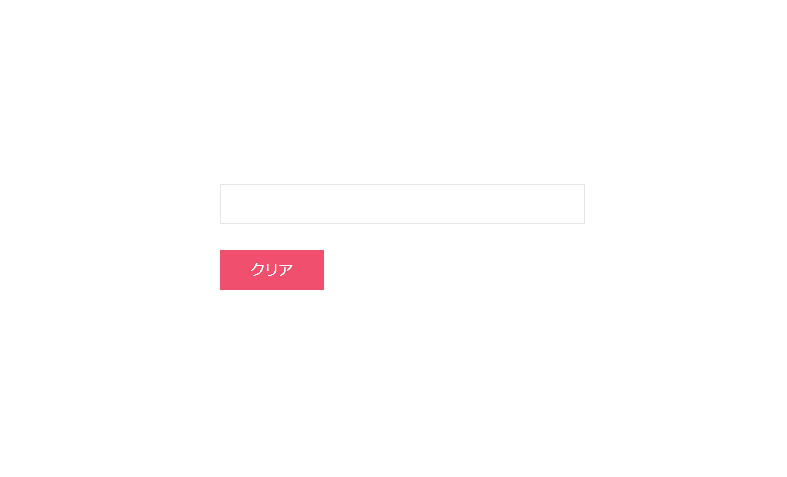
javascriptを使って入力したフォームの値を削除するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 102.0.5005.63
値削除
フォームに入力された値を削除するには、「value」を空に指定します。
document.getElementById("フォームのid名").value = '';
実際に、実行してクリアしてみます。
<input id="txt" type="text" value="foo">
<input id="btn" type="button" value="ボタン" />
<script>
'use strict';
function hoge() {
document.getElementById("txt").value='';
}
document.getElementById("btn").addEventListener('click', hoge, false);
</script>
実行結果を見ると削除されていることが確認できます。
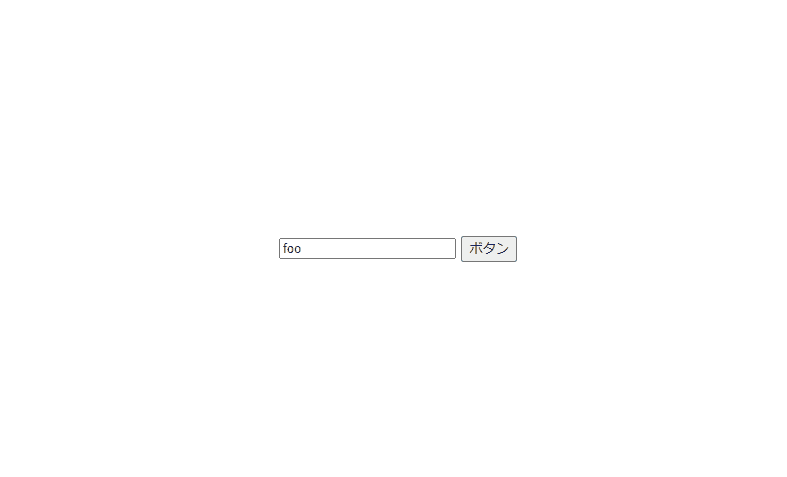
サンプルコード
以下は、
クリア ボタンをクリックすれば、入力したテキストの値を削除するサンプルコードとなります。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<!-- UIkit CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/uikit@3.5.5/dist/css/uikit.min.css" />
</head>
<style>
.container {
margin: 0 auto;
margin-top: 200px;
display: flex;
flex-direction: column;
align-items: center;
font-size: 30px;
}
</style>
<script>
function clearText() {
let txtVal = document.getElementById("txt");
txtVal.value = '';
}
</script>
<body>
<div class="container">
<form>
<fieldset class="uk-fieldset">
<div class="uk-margin">
<input class="uk-input" type="text" id="txt">
</div>
<button type="button" class="uk-button uk-button-danger" onclick="clearText()">クリア</button>
</fieldset>
</form>
</div>
</body>
</html>
実行結果を確認すると入力したフォームの内容が削除されていることが確認できます。
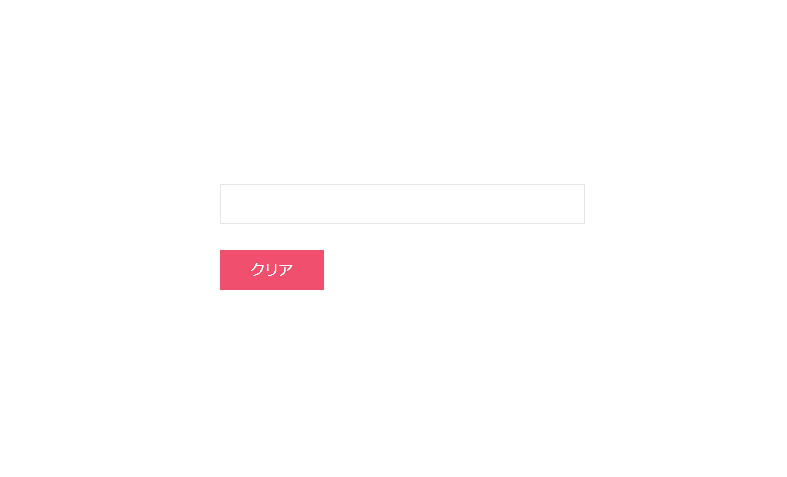
また、javascript部分を関数をアロー関数でdocument.getElementByIdを省略して簡潔に記述することも可能です。
const clearText = () =>{ txt.value = '' }
-
前の記事
javascript 前と後ろにある空白を全て削除する 2020.08.24
-
次の記事
php PHP_CodeSnifferを使用して構文チェックを行う 2020.08.24
コメントを書く