javascript 数値を指定した通貨単位に変換する
- 作成日 2022.06.25
- 更新日 2023.01.16
- javascript
- javascript
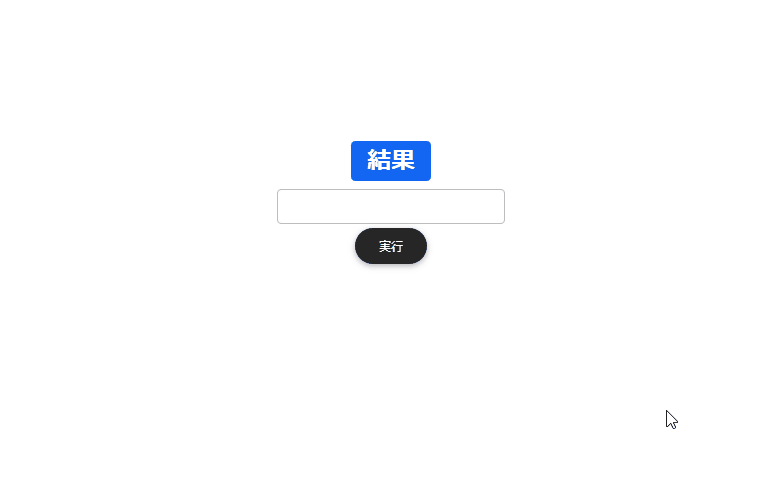
javascriptで、数値を指定した通貨単位に変換するサンプルコードを記述してます。「Intl.NumberFormat」に「USD」などの通貨単位を指定することで変換できます。「toLocaleString」を使用する方法もあります。
環境
- OS windows11 home
- ブラウザ chrome 109.0.5414.75
数値を指定した通貨単位に変換
数値を指定した通貨単位に変換するには「Intl.NumberFormat」を使用して、通貨単位を指定します。
例えば「$」に変換する場合は、localeを「en-US」に指定して「USD」を指定します。
const f = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD'
});
console.log( f.format(1000) ) // $1,000.00
「ユーロ」の場合は、以下を指定します。
const f = new Intl.NumberFormat('de-DE', {
style: 'currency',
currency: 'EUR'
});
console.log( f.format(1000) ) // 1.000,00 €
「円」にも変換できます。
const f = new Intl.NumberFormat('ja-JP', {
style: 'currency',
currency: 'JPY'
});
console.log( f.format(1000) ) // ¥1,000
「zh-Hans-CN-u-nu-hanidec」を使用すると、漢数字に変換することもできます。
const f = new Intl.NumberFormat('zh-Hans-CN-u-nu-hanidec');
console.log( f.format(1234567890) ) // 一,二三四,五六七,八九〇
toLocaleStringを使用
「toLocaleString」を使用しても同じ結果となります。
const result = (1000).toLocaleString('en-US', {
style: 'currency',
currency: 'USD'
});
console.log( result ); // $1,000.00
パフォーマンスは「toLocaleString」の方が少しだけ良さそうです。
サンプルコード
以下は、実行ボタンをクリックすると、数値を通貨「$」に変換して表示するだけのサンプルコードとなります。
※cssには「Material Design for Bootstrap」を使用してます。関数はアロー関数を使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<!-- MDB -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/mdb-ui-kit/4.1.0/mdb.min.css" rel="stylesheet" />
</head>
<body>
<div class="container text-center w-25" style="margin-top:150px">
<h2><span id="result" class="badge bg-primary">結果</span></h2>
<form name="frm">
<input type="number" id="n" class="form-control w-50 mx-auto"/>
</form>
<button id="btn" class="btn btn-dark btn-rounded mt-1">実行</button>
</div>
<script>
btn.onclick = () => {
result.innerHTML = new Intl.NumberFormat('en-US', { style: 'currency', currency: 'USD' } ).format(n.value)
}
</script>
</body>
</html>
変換されていることが確認できます。
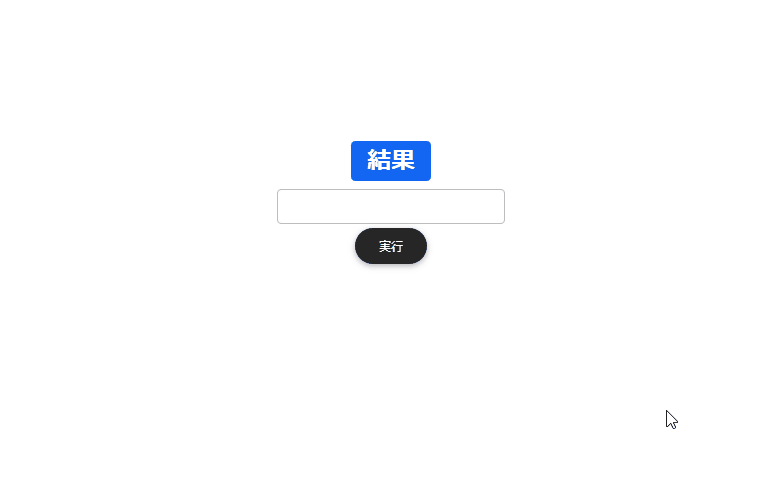
-
前の記事
Linux CSVから指定したカラムのデータを抽出する 2022.06.25
-
次の記事
Rocky Linux 「buff/cache」を削除する 2022.06.25
コメントを書く