javascript イベント発生元の親要素までのイベントを取得する
- 作成日 2021.11.18
- 更新日 2022.10.08
- javascript
- javascript
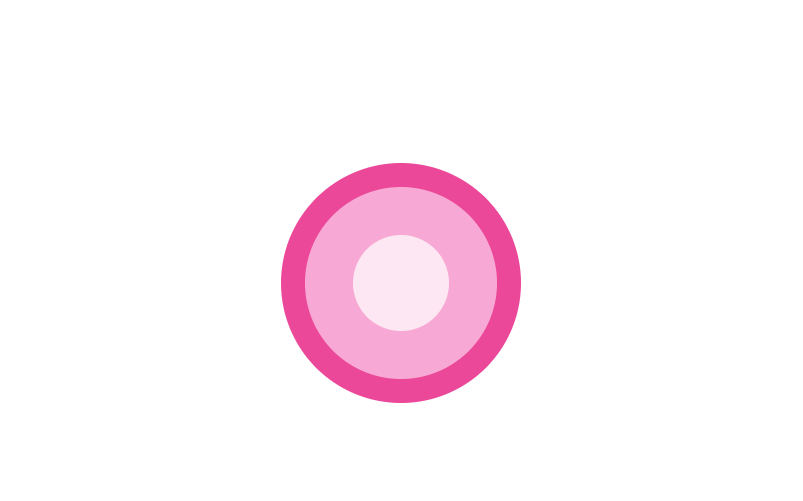
javascriptで、イベント発生元の親要素までのイベントを取得するサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows11 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 106.0.5249.103
イベント発生元の親要素のまでイベントを取得
イベント発生元の親要素までのイベントを取得するには、「Event.currentTarget」を使用します。
<div id="oya">
<input id="ko" type="button" value="ボタン" />
</div>
<script>
'use strict'
function hoge(e) {
console.log(`子要素が実行されました。 id : ${e.currentTarget.id}`)
}
function foo(e) {
console.log(`親要素が実行されました。 id : ${e.currentTarget.id}`)
}
document.getElementById("ko").addEventListener('click', hoge, false)
document.getElementById("oya").addEventListener('click', foo, false)
</script>
実行結果を確認すると、ボタンをクリックすると、クリックされたボタンの親要素までのイベントが取得されていることが確認できます。
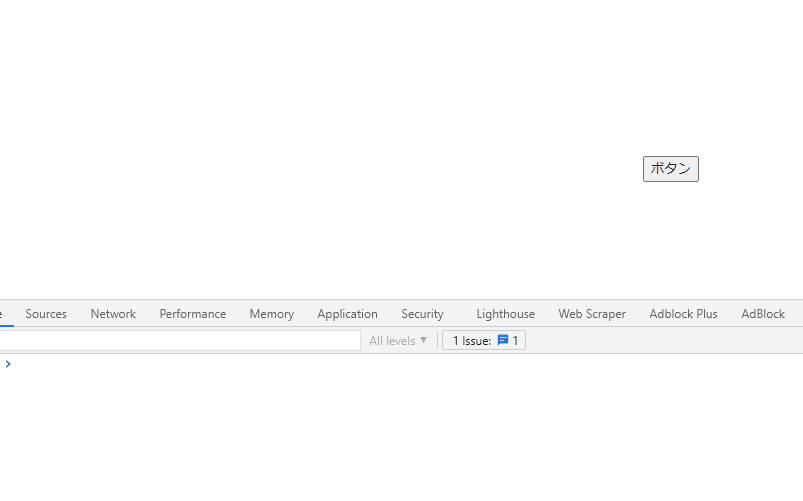
また、以下のコードを、
function hoge(e) {
console.log(`子要素が実行されました。 id : ${e.currentTarget.id}`)
}
function foo(e) {
console.log(`親要素が実行されました。 id : ${e.currentTarget.id}`)
}
document.getElementById("ko").addEventListener('click', hoge, false)
document.getElementById("oya").addEventListener('click', foo, false)
アロー関数とdocument.getElementByIdを省略して、簡潔に記述することもできます。
const hoge = (e) =>{
console.log(`子要素が実行されました。 id : ${e.currentTarget.id}`)
}
const foo = (e) =>{
console.log(`親要素が実行されました。 id : ${e.currentTarget.id}`)
}
ko.addEventListener('click', hoge, false)
oya.addEventListener('click', foo, false)
サンプルコード
以下は、
一番最後の要素のクリックイベントで、それぞれ親にあたる要素までのイベントを発生させて、それぞれの要素の「id」を表示するサンプルコードとなります。
※cssには「tailwind」を使用して、アロー関数で関数は定義してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css" rel="stylesheet">
</head>
<style>
</style>
<script>
window.onload = () => {
one.onclick = (e) => {
oya.textContent = `currentTarget : ${e.currentTarget.id}`
}
two.onclick = (e) => {
ko.textContent = `currentTarget : ${e.currentTarget.id}`
}
three.onclick = (e) => {
mago.textContent = `currentTarget : ${e.currentTarget.id}`
}
}
</script>
<body>
<div class="container mx-auto my-56 w-1/3 px-4">
<div id="sample" class="flex flex-col justify-center">
<h1 id="oya" class="font-semibold text-gray-500 text-lg mr-auto"></h1>
<h1 id="ko" class="font-semibold text-gray-500 text-lg mr-auto"></h1>
<h1 id="mago" class="font-semibold text-gray-500 text-lg mr-auto"></h1>
<div id="one" class="bg-pink-500 rounded-full h-60 w-60 flex items-center justify-center ">
<div id="two" class="bg-pink-300 rounded-full h-48 w-48 flex items-center justify-center">
<div id="three" class="bg-pink-100 rounded-full h-24 w-24 flex items-center justify-center">
</div>
</div>
</div>
</div>
</div>
</body>
</html>
実行結果を確認すると、親要素のまでの「id」表示されていることが確認できます。
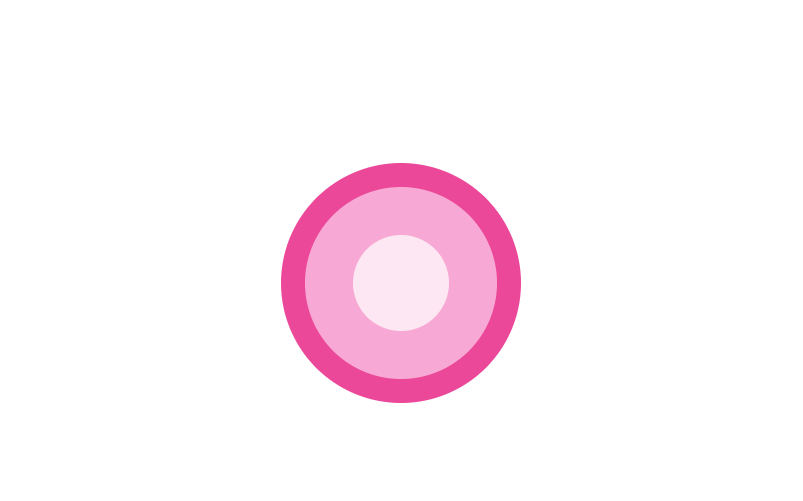
-
前の記事
rails6 EXCEL最終行まで読み込む 2021.11.17
-
次の記事
php round(四捨五入)・ceil(切り上げ)・floor(切り捨て)を実行する 2021.11.18
コメントを書く