javascript lodashを使って関数をまとめて実行する
- 作成日 2021.06.21
- 更新日 2022.04.19
- javascript lodash
- javascript, lodash
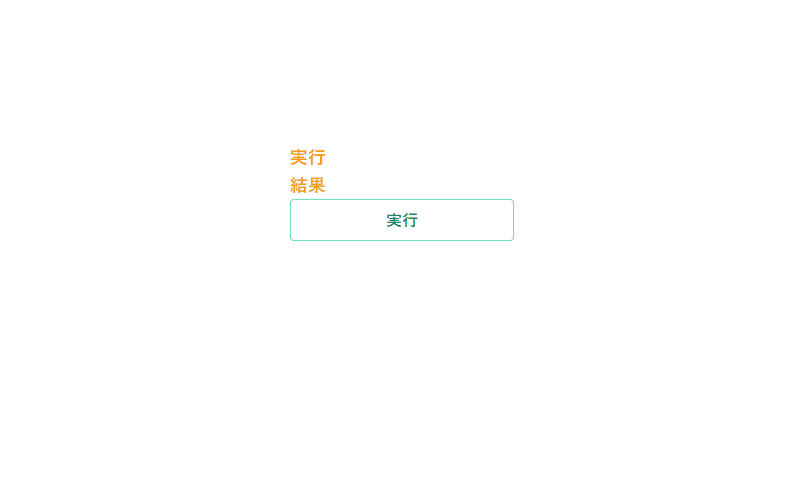
javascriptで、lodashを使って関数をまとめて実行するサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- lodash 4.17.21
- ブラウザ chrome 91.0.4472.77
lodash使用
こちらのサイトから最新版を確認して、CDN版を使用してます。
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.21/lodash.min.js"></script>
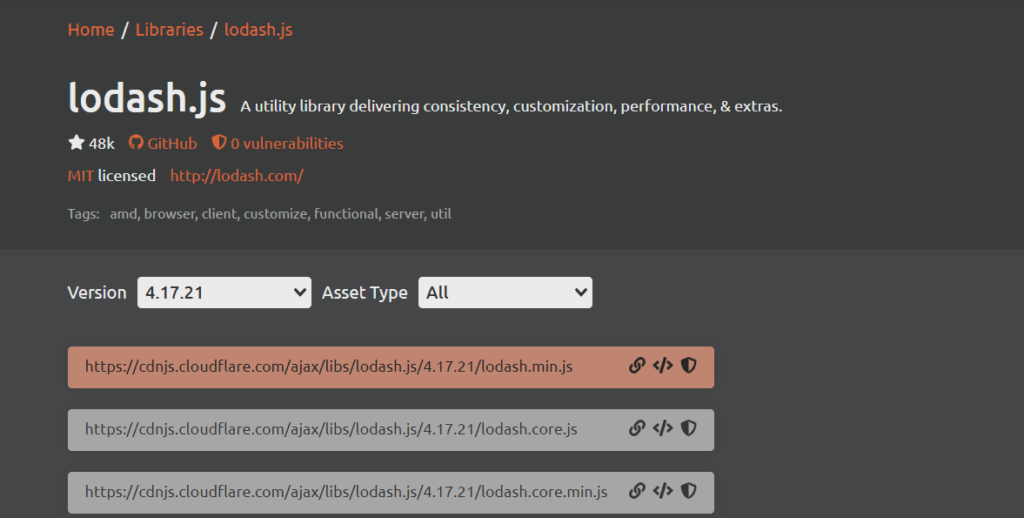
関数をまとめて実行
関数をまとめて実行するには、「_.overArgs」を使用します。
'use strict';
function hoge(x) {
return x * 2;
}
function foo(x) {
return x * x;
}
const func = _.overArgs(function (x, y) {
return [x, y];
}, [hoge, foo]);
console.log(
func(2, 2) // [4, 4]
)
console.log(
func(3, 3) // [6, 9]
)
実行結果を確認すると、関数がまとめられて実行されていることが確認できます。
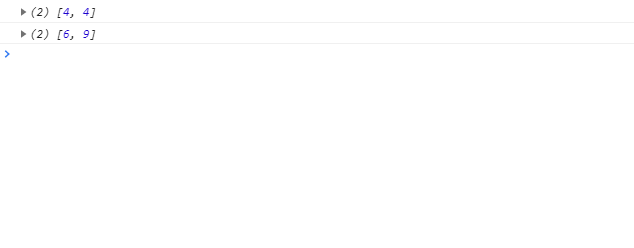
サンプルコード
以下は、
「実行」ボタンをクリックして、3個の関数をまとめて実行するサンプルコードとなります。
※cssには「tailwind」を使用して、アロー関数で関数は定義してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css" rel="stylesheet">
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.21/lodash.min.js"></script>
</head>
<script>
const hoge = (x) => {
bar1.innerHTML = x
}
const foo = (x) => {
bar2.innerHTML = x
}
const fuga = (x) => {
bar3.innerHTML = x
}
const f = _.overArgs((x, y, z) => [x, y, z], [hoge, foo, fuga]);
window.onload = () => {
btn.onclick = () => { f("hello", "world", "!!") }
}
</script>
<body>
<div class="container mx-auto my-56 w-64 px-4">
<div id="sample" class="flex flex-col justify-center">
<h1 id="bar1" class="font-semibold text-yellow-500 text-lg mr-auto">実行</h1>
<h1 id="bar2" class="font-semibold text-yellow-500 text-lg mr-auto">結果</h1>
<h1 id="bar3" class="font-semibold text-yellow-500 text-lg mr-auto"></h1>
<button id="btn"
class="mb-2 md:mb-0 bg-transparent hover:bg-green-300 text-green-700 font-semibold hover:text-white py-2 px-4 border border-green-300 hover:border-transparent rounded">
実行
</button>
</div>
</div>
</body>
</html>
実行結果を確認すると、まとめて実行されていることが確認できます。
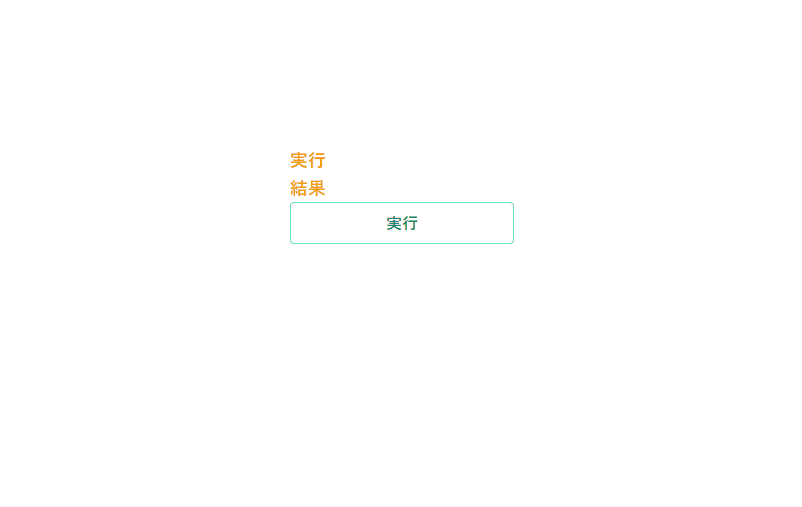
-
前の記事
C# テキストファイル内のデータの行数をカウントする 2021.06.20
-
次の記事
javascript オブジェクトの配列のソートを行う 2021.06.21
コメントを書く