rails6 APIを実装する
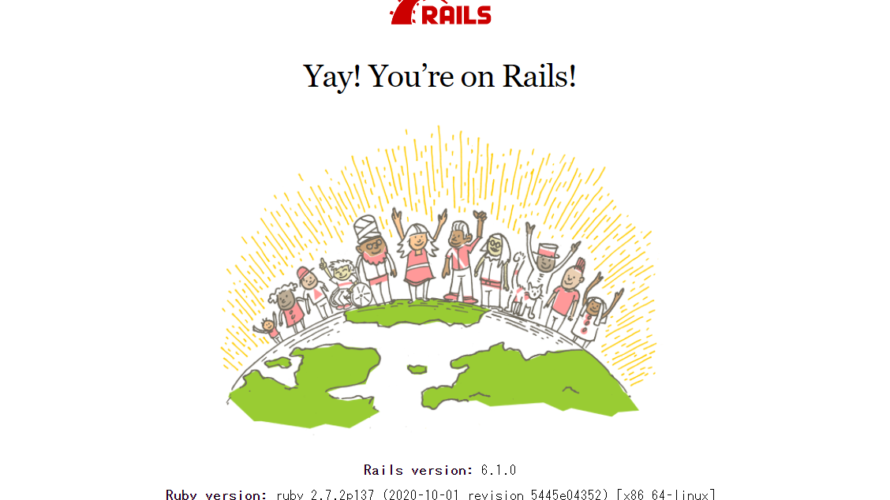
rails6で、APIを実装するまでの手順を記述してます。APIを確認するために「Talend API Tester」を使用してます。railsのバージョンは6.1.0です。
環境
- OS ubuntu20.10
- ruby 2.7.2
- rails 6.1.0
- Postgresql 13.1 (dockerで構築)
テーブルとモデル作成
テーブル「customers」を作成します。
rails g model Customer name:string email:string
マイグレーションを実行します。
rails db:migrate
テーブル「customers」

ルーティング設定
「config」ディレクトリ配下にある「routes.rb」に以下を追加します。
Rails.application.routes.draw do
root "top#index"
# 追加
namespace :api, format: "json" do
resources :customers, only: [:index, :create, :update]
end
end
コントローラー作成
「app/controllers」に「api」ディレクトリを作成して「customers_controller.rb」を作成します。
class Api::CustomersController < ApplicationController
skip_before_action :verify_authenticity_token
def index
@customers = Customer.all
end
def create
@customer = Customer.new(customer_params)
if @customer.save
render :show, status: :created
else
render json: @customer.errors, status: :unprocessable_entity
end
end
def update
@customer = Customer.find(params[:id])
if @customer.update(customer_params)
render :show, status: :ok
else
render json: @customer.errors, status: :unprocessable_entity
end
end
private
def customer_params
params.fetch(:customer, {}).permit(
:name, :email
)
end
end
APIデータ作成
Fakerを使って、ダミーデータを作成してます。
jbuilderテンプレート作成
「app/views/api/customers」ディレクト配下に、「index.json.jbuilder」
json.set! :customers do
json.array! @customers do |customer|
json.extract! customer, :id, :name, :email, :created_at, :updated_at
end
end
「show.json.jbuilder」を作成します。
json.set! :customer do
json.extract! @customer, :id, :name, :email, :created_at, :updated_at
end
API確認
Talend API Testerを使用して確認してます。
get
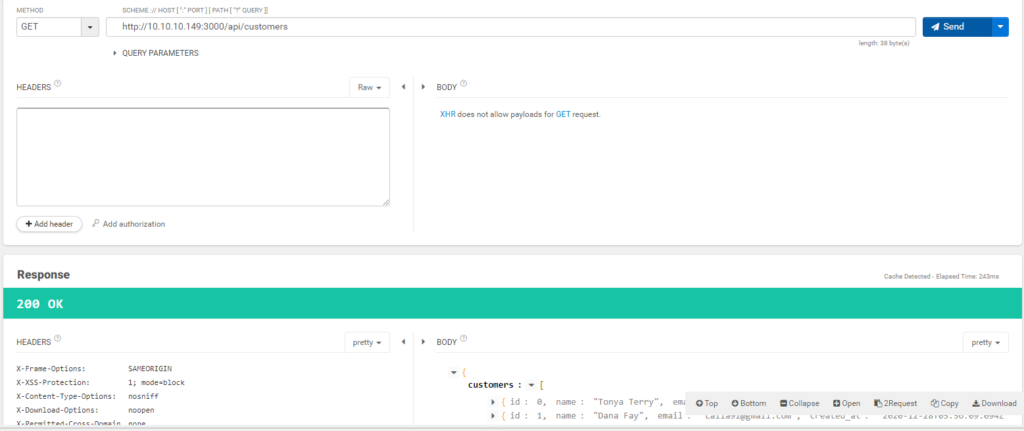
post
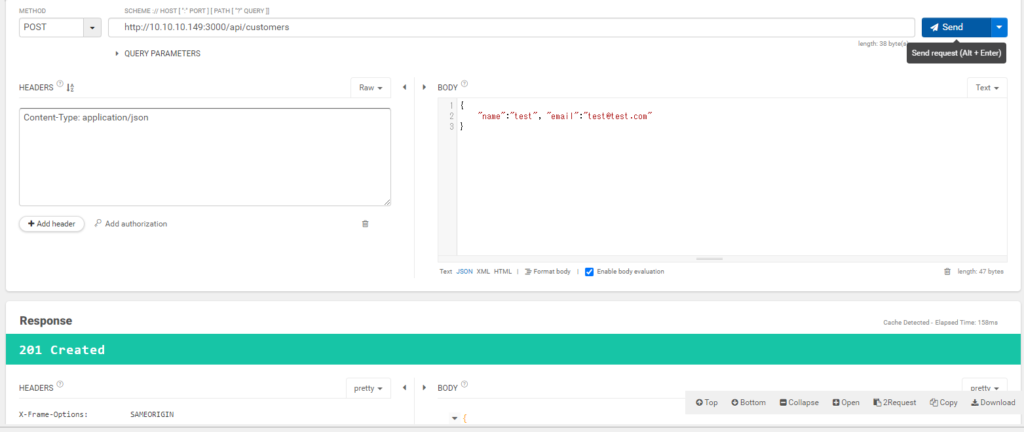
curlコマンドでも確認できます。
get
curl localhost:3000/api/customers

post
curl -X POST localhost:3000/api/customers -d 'customer[name]=hoge&customer[email]=test@test.com'

自分が一番利用するpostmanを使用した場合は、以下となります。
get
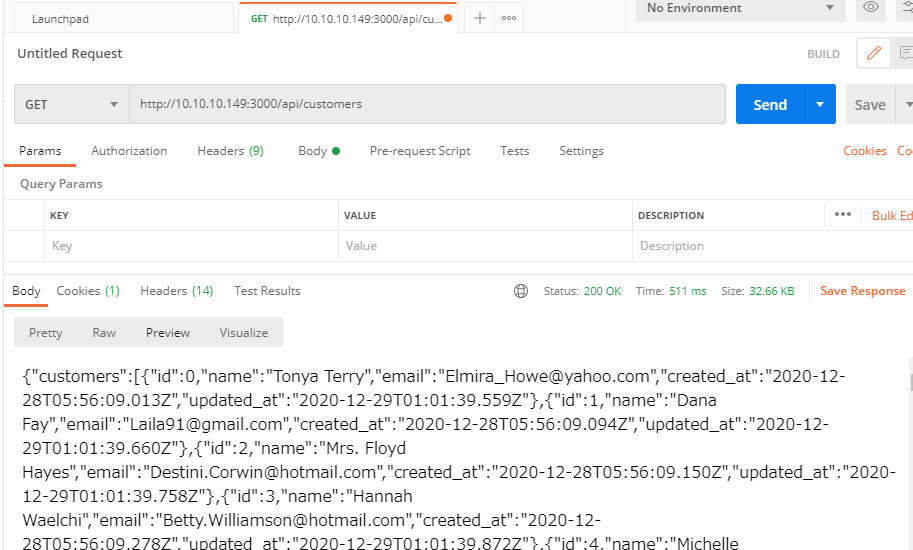
post
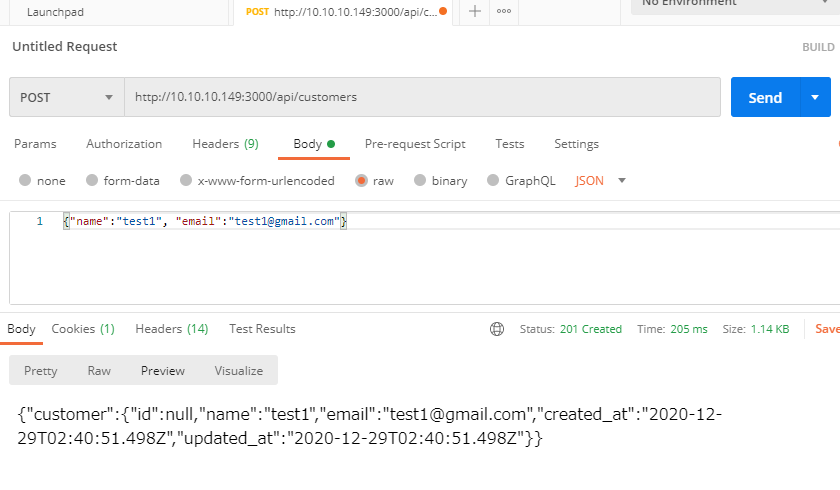
-
前の記事
Alpine.jsを使ってhoverで表示されるツールチップを作成する 2021.01.16
-
次の記事
javascript ノードを移動させる 2021.01.16
コメントを書く