javascript オーバーライドを行う
- 作成日 2021.01.12
- 更新日 2022.08.02
- javascript
- javascript
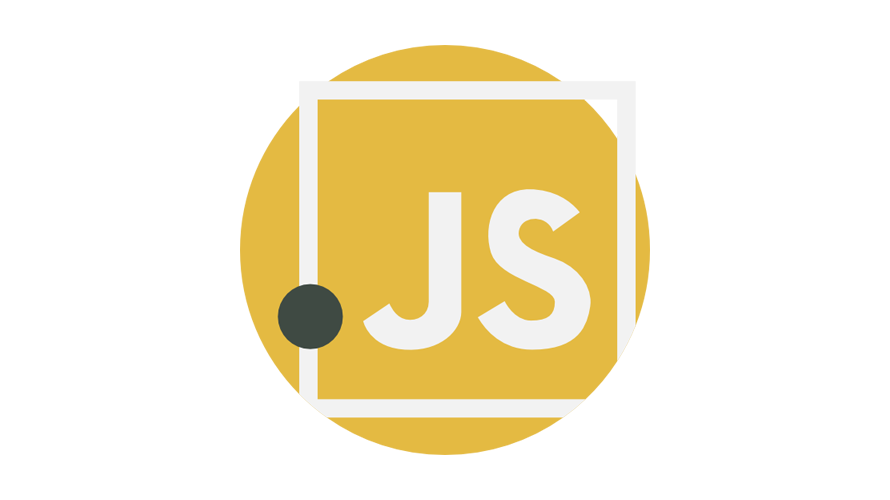
javascriptで、ES6(ECMAScript 2015)から利用することができるクラス構文のオーバーライドを行う方法を記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 103.0.5060.134
オーバーライド
以下のクラスを、継承してメソッドをオーバライドしてみます
class Hoge {
constructor(num) {
this._num = num;
}
// 静的メソッドの定義
calc() {
return this._num + this._num;
}
}
const hoge = new Hoge(3);
console.log(hoge.calc()); // 6
継承してメソッドをオーバライドします。
class Hoge {
constructor(num) {
this._num = num;
}
// 静的メソッドの定義
calc() {
return this._num + this._num;
}
}
class Foo extends Hoge {
calc() {
return this._num * this._num;
}
}
const hoge = new Hoge(3);
console.log(hoge.calc()); // 6
const foo = new Foo(3);
console.log(foo.calc()); // 9
ちゃんとメソッドがオーバライドされて、実行結果が変わることが確認できます。
constructorのオーバーライド
constructorもオーバーライドできます。オーバーライドするには「super」を使用します。
class Hoge {
constructor(num) {
this._num = num;
}
// 静的メソッドの定義
calc() {
return this._num + this._num;
}
}
class Foo extends Hoge {
constructor(num,str) {
super(num,str)
this._num = num;
this._str = str;
}
calc() {
return this._num * this._num;
}
disp() {
return this._str
}
}
const foo = new Foo(3,"abc");
console.log(foo.disp()); // abc
関数のオーバーライド
関数は、同じ名称だった場合は、最後に定義されているものが実行されるためオーバーライドはできません。
function fn(x) {
console.log("引数1つが実行されました");
}
function fn(x,y) {
console.log("引数2つが実行されました");
}
fn(1);
fn(1,2);
実行結果

-
前の記事
Ruby 配列をランダムに並び替える 2021.01.12
-
次の記事
rbenv install時に「ruby-build: TMPDIR=/xxx is set to a non-accessible location」が発生 2021.01.13
コメントを書く