javascript 開いた別ウィンドウを閉じる
- 作成日 2020.09.18
- 更新日 2022.06.23
- javascript
- javascript
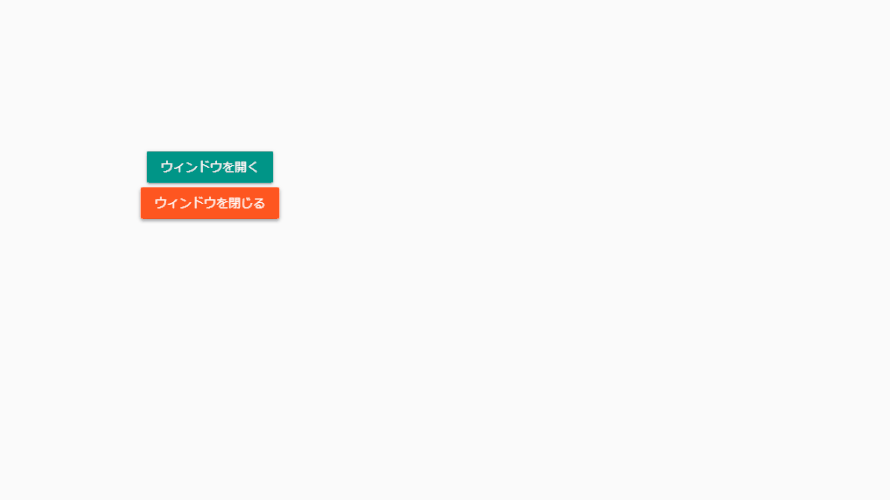
javascriptで、window.open()を使用して開いた別ウィンドウを閉じるサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 102.0.5005.115
閉じ方
別ウィンドウで開いたものを、「close」すれば閉じることが可能です。
// 開く
obj = window.open(
'https://mebee.info/',
'_blank',
'width=800, height=800,'
);
// 閉じる
obj.closed
サンプルコード
以下は、
「ウィンドウを開く」ボタンをクリックして、開いた別ウィンドウを、
「ウィンドウを閉じる」で閉じる
サンプルコードとなります。
※cssには「bootstrap material」を使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700|Material+Icons">
<link rel="stylesheet"
href="https://unpkg.com/bootstrap-material-design@4.1.1/dist/css/bootstrap-material-design.min.css"
integrity="sha384-wXznGJNEXNG1NFsbm0ugrLFMQPWswR3lds2VeinahP8N0zJw9VWSopbjv2x7WCvX" crossorigin="anonymous">
</head>
<style>
.main {
margin: 0 auto;
margin-top: 200px;
display: flex;
flex-direction: column;
align-items: center;
font-size: 25px;
}
</style>
<script>
// 変数を用意
let obj;
function hoge() {
// 別ウィンドウを開く
obj = window.open(
'https://mebee.info/',
'_blank',
'width=800, height=800,'
);
}
function foo() {
// 開かれていれば閉じる
if ((obj) && (!obj.closed)) {
obj.close();
}
else {
console.log('別ウィンドウは開かれておりません。');
}
obj = null;
}
</script>
<body>
<div class="main">
<button onclick="hoge()" type="button" class="btn btn-raised btn-primary">
ウィンドウを開く
</button>
<button onclick="foo()" type="button" class="btn btn-raised btn-warning">
ウィンドウを閉じる
</button>
</div>
</body>
</html>
別ウィンドウが閉じられてることが確認できます。
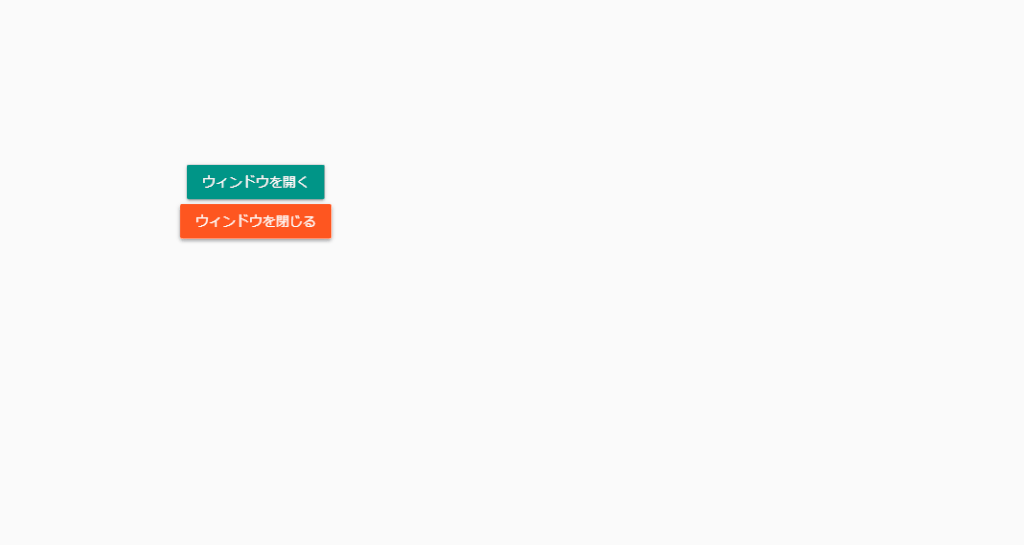
また、javascript部は三項演算子を使用して、windowオブジェクトを省略して記述すると少し簡潔に記述することが可能です。
function hoge() {
// 別ウィンドウを開く
obj = open(
'https://mebee.info/',
'_blank',
'width=800, height=800,'
);
}
function foo() {
// 開かれていれば閉じる
((obj) && (!obj.closed)) ? obj.close() : console.log('別ウィンドウは開かれておりません。')
obj = null;
}
-
前の記事
VirtualBoxにReactOSをインストールして利用する 2020.09.18
-
次の記事
javascript html要素を表示・非表示を切り替える 2020.09.18
コメントを書く