javascript 指定したhtml要素にフォーカスを当てる
- 作成日 2020.09.17
- 更新日 2022.06.23
- javascript
- javascript
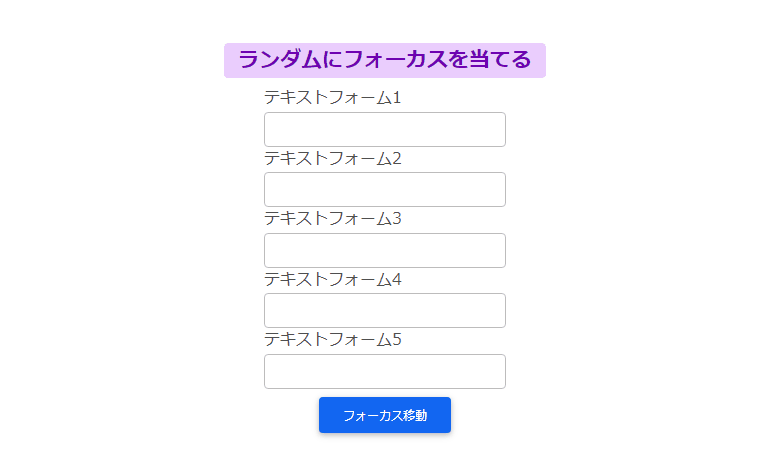
javascriptで、focusメソッドを使用して、指定したhtml要素にフォーカスを当てるサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 102.0.5005.115
focusメソッド使い方
focusメソッドを使うと、指定したhtml要素にフォーカスを当てることが可能です。
// idを指定した場合
document.getElementById('id名').focus();
// formから指定した場合
document.フォームのname属性値.name属性値.focus();
// nameを指定した場合
document.getElementsByName(name属性値)[番号].focus();
// classを指定した場合
document.getElementsByClassName(クラス名)[番号].focus();
document.getElementByIdを省略して、以下のように「id名」のみで記述することも可能です。
id名.focus();
focus使い方
<idを指定した場合>
<form name="hoge">
<input type="text" id='bar' name="foo">
<input type="text" name="foo2">
</form>
<script>
bar.focus();
</script>
実行結果

<formを指定した場合>
<form name="hoge">
<input type="text" name="foo">
<input type="text" name="foo2">
</form>
<script>
document.hoge.foo2.focus();
</script>
実行結果

<nameを指定した場合>
<form name="hoge">
<input type="text" name="foo">
<input type="text" name="foo2">
</form>
<script>
document.getElementsByName("foo2")[0].focus();
</script>
実行結果

<classを指定した場合>
<form name="hoge">
<input type="text" name="foo" class="bar">
<input type="text" name="foo2" class="bar">
</form>
<script>
document.getElementsByClassName("bar")[1].focus();
</script>
実行結果

サンプルコード
以下は、「フォーカス移動」ボタンをクリックすると、ランダムにテキストフォームを移動するサンプルコードとなります。
※cssには「bootstrap material」を使用してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<!-- MDB -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/mdb-ui-kit/4.2.0/mdb.min.css" rel="stylesheet" />
</head>
<style>
.main {
margin: 0 auto;
margin-top: 200px;
display: flex;
flex-direction: column;
align-items: center;
}
</style>
<script>
function hoge() {
// ランダムなidにフォーカスを移動させる
document.getElementById("txt" + Math.floor(Math.random() * 5 + 1)).focus();
};
</script>
<body>
<div class="main">
<h3><span class="badge badge-secondary">ランダムにフォーカスを当てる</span></h3>
<form class="form-group">
<div class="md-form">
<label for="txt1">テキストフォーム1</label>
<input type="text" class="form-control" id="txt1">
<label for="txt2">テキストフォーム2</label>
<input type="text" class="form-control" id="txt2">
<label for="txt2">テキストフォーム3</label>
<input type="text" class="form-control" id="txt3">
<label for="txt4">テキストフォーム4</label>
<input type="text" class="form-control" id="txt4">
<label for="txt5">テキストフォーム5</label>
<input type="text" class="form-control" id="txt5">
</div>
</form>
<button type="button" class="btn btn-raised btn-primary mt-2" onclick="hoge()">フォーカス移動</button>
</div>
</body>
</html>
移動していることが確認できます。
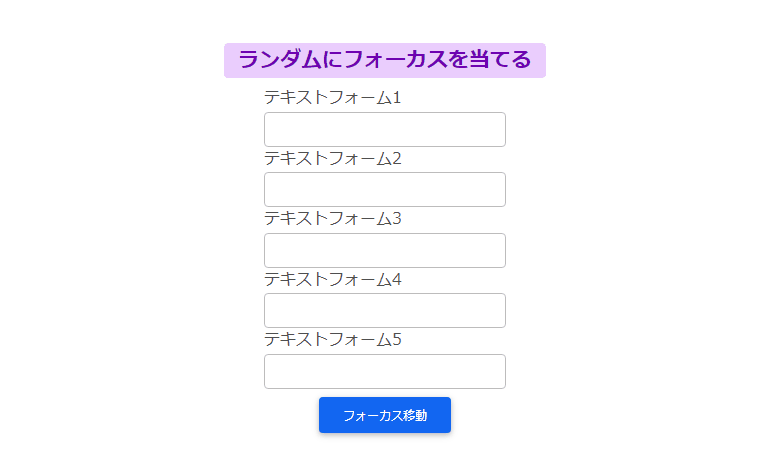
-
前の記事
OpenLiteSpeed バーチャルホストの設定手順 2020.09.17
-
次の記事
javascript placeholderに指定した値を取得する 2020.09.17
コメントを書く