React.js ライブラリ「React-StableList」をインストールしてリストビューを実装する
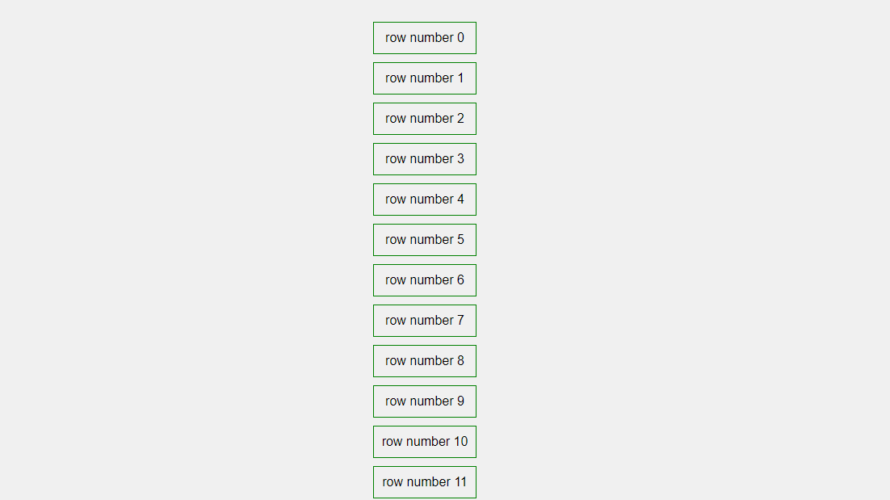
ライブラリ「React-StableList」をインストールすると、リストビューを実装するが可能です。ここでは、react.jsでReact-StableListを利用するための手順と簡単な使い方を記述してます。
環境
- OS CentOS Linux release 8.0.1905 (Core)
- node V12.13.1
- npm 6.14.2
- React 16.13.0
react.js環境構築
下記のコマンドで構築してます。ここでは、react-appという名前でプロジェクトを作成してます。
create-react-app react-app
React-StableListインストール
作成したプロジェクトに移動して、インストールします。
## 作成したプロジェクトに移動
cd react-app
## インストール
npm install react-stablelist
React-StableList使い方
srcディレクトリにsample.jsと名前で下記のコードを記述します。
import React from 'react';
import StableList from "react-stablelist";
import { v4 as uuid } from "uuid";
import clsx from "clsx";
import "./style.css";
const makeData = count => [...Array(count).keys()];
const propProvider = (key, index, isFresh, isFirstRender, propData) => {
return {
key,
content: `row number ${propData}`
};
};
const sampleData = makeData(45000);
const SpecialComponent = ({ content, className }) => {
return <div className={clsx(className, "sepcial-item")}>{content}</div>;
}
const Sample = () => {
return (
<div>
<StableList
data={sampleData}
dataKey={uuid()}
component={SpecialComponent}
itemCount={sampleData.length}
propProvider={propProvider}
/>
</div>
);
}
export default Sample;
次に、srcディレクトリ配下にあるApp.jsを下記のように編集します。
import React from 'react';
import Sample from './sample';
import './App.css';
function App() {
const style = {
margin: "0 auto",
marginTop: 150,
};
return (
<div className="App">
<div style={style}>
<Sample />
</div>
</div>
);
}
export default App;
最後に、src配下に style.css を作成して以下を追加します。
.sepcial-item {
padding: 10px;
margin: 10px 0;
border: thin solid green;
}
.sepcial-item.animate {
animation: fade-in 1s ease;
}
@keyframes fade-in {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
実行します。
npm start
ブラウザから http://プライベートIP:3000にアクセスすると、リストビュー が表示されていることが確認できます。
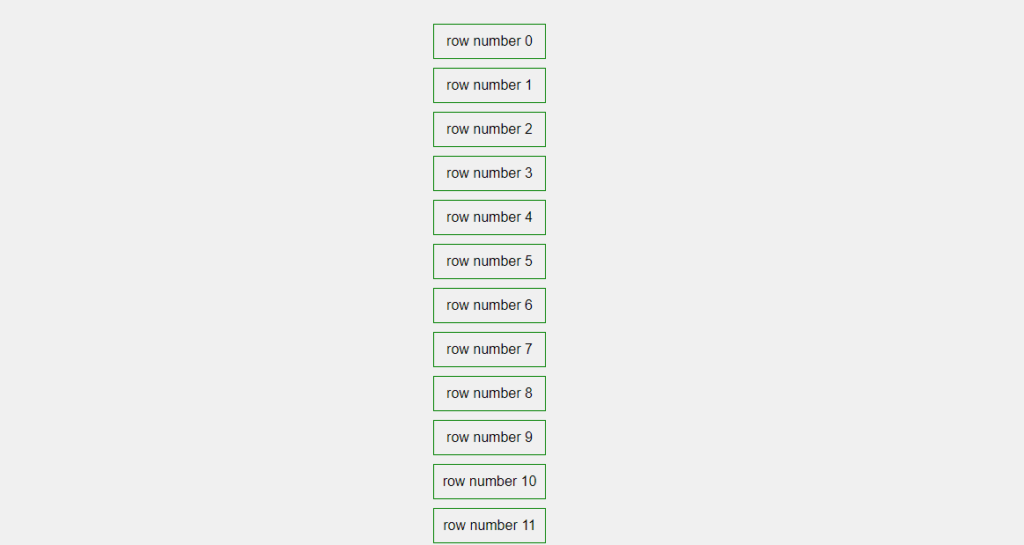
-
前の記事
ZorinOSを日本語化する 2020.07.21
-
次の記事
php ファイルの拡張子を取得する方法 2020.07.22
コメントを書く