javascript 数値を文字列に変換する
- 作成日 2022.08.02
- javascript
- javascript
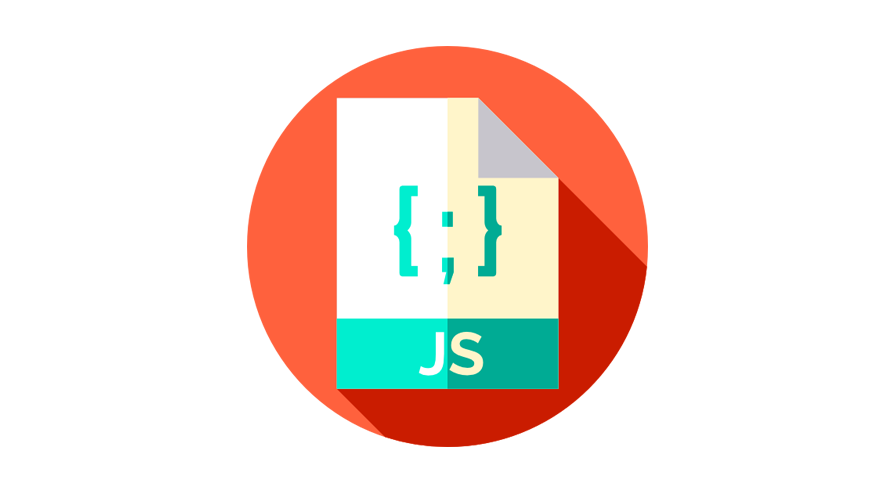
javascriptで、数値を文字列に変換するサンプルコードを掲載してます。ブラウザはchromeを使用しています。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 103.0.5060.134
文字列に変換
数値を文字列に変換するは、「String」を使用します。
String(数値)
実際に変換してます。
let num = 11
console.log( String( num ) ) // 11
console.log( typeof( String(num) ) ) // string
num = 11.11
console.log( String( num ) ) // 11.11
console.log( typeof( String(num) ) ) // string
num = 0.11
console.log( String( num ) ) // 0.11
console.log( typeof( String(num) ) ) // string
num = -0.11
console.log( String( num ) ) // -0.11
console.log( typeof( String(num) ) ) // string
また、「リテラルテンプレート」や「toString」を使用して変更することも可能です。
let num = 11
console.log( `${num}` ) // 11
console.log( typeof( `${num}` ) ) // string
console.log( num.toString() ) // 11
console.log( typeof( num.toString() ) ) // string
// '' + num でも同じ結果になります。
console.log( num + '' ) // 11
console.log( typeof( num + '' ) ) // string
num = 11.11
console.log( `${num}` ) // 11.11
console.log( typeof( `${num}` ) ) // string
console.log( num.toString() ) // 11.11
console.log( typeof( num.toString() ) ) // string
console.log( num + '' ) // 11.11
console.log( typeof( num + '' ) ) // string
num = 0.11
console.log( `${num}` ) // 11.11
console.log( typeof( `${num}` ) ) // string
console.log( num.toString() ) // 11.11
console.log( typeof( num.toString() ) ) // string
console.log( num + '' ) // 11.11
console.log( typeof( num + '' ) ) // string
num = -0.11
console.log( `${num}` ) // -0.11
console.log( typeof( `${num}` ) ) // string
console.log( num.toString() ) // -0.11
console.log( typeof( num.toString() ) ) // string
console.log( num + '' ) // -0.11
console.log( typeof( num + '' ) ) // string
パフォーマンスは「+」か「リテラルテンプレート」を使用する方法が一番いいです。
toFixed()
少数の桁数を指定する必要がありますが「toFixed()」でも変換可能です。
※「toFixed()」は、表示したい少数の桁数を引数に指定します。
let num = 11
console.log( num.toFixed() ) // 11
console.log( typeof( num.toFixed() ) ) // string
num = 11.11
console.log( num.toFixed(2) ) // 11.11
console.log( typeof( num.toFixed(2) ) ) // string
num = 0.11
console.log( num.toFixed(2) ) // 0.11
console.log( typeof( num.toFixed(2) ) ) // string
num = -0.11
console.log( num.toFixed(2) ) // -0.11
console.log( typeof( num.toFixed(2) ) ) // string
-
前の記事
MariaDB 数値にパーセントを付与する 2022.08.02
-
次の記事
PostgreSQL 日や月の足し算を行う 2022.08.02
コメントを書く