ASP.NET core mongoDBを使用してAPIを作成する
- 作成日 2021.12.30
- ASP.NET Core mongoDB
- ASP.NET Core, mongodb
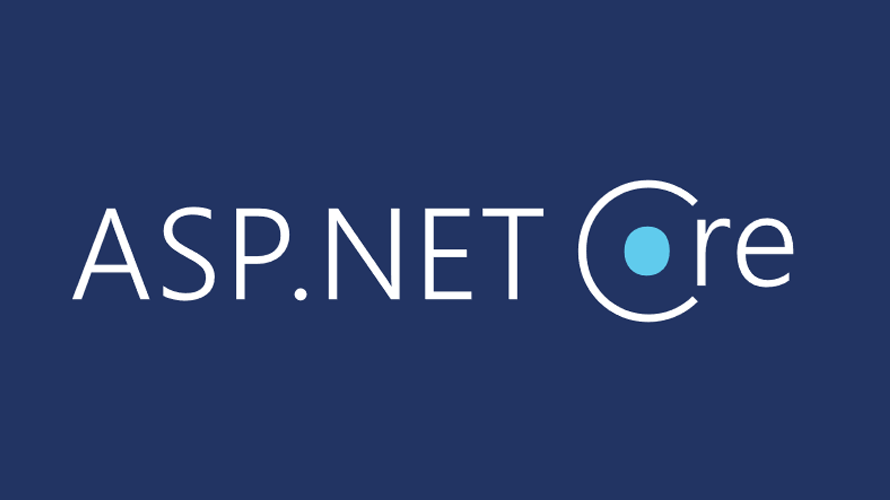
ASP.NET coreでmongoDBを使用してAPIを作成する順を記述してます。asp.coreのバージョンは「6」です。
目次
環境
- OS windows 10 pro
- asp.core 6
- mongoDB 5.0.5
- visual stadio 2022
APIプロジェクト作成
まずはプロジェクトを作成します。「ASP.NET Core Web API」を選択します。
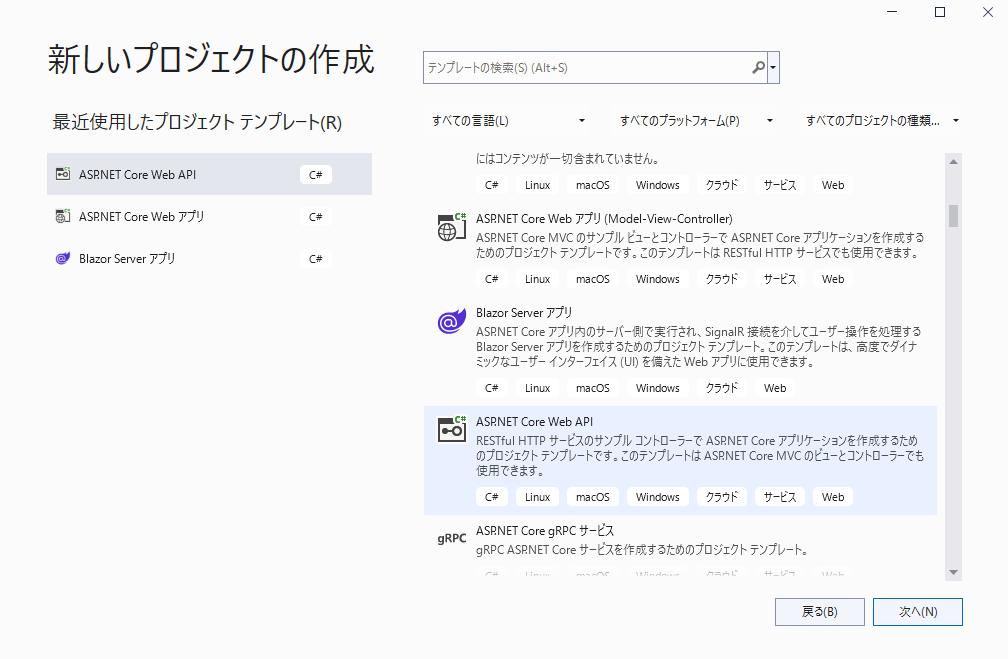
任意のプロジェクト名で作成します。
※すいません。「mysqlSample」とちょっとわかりにくい名前になっているのは使いまわしているからです。
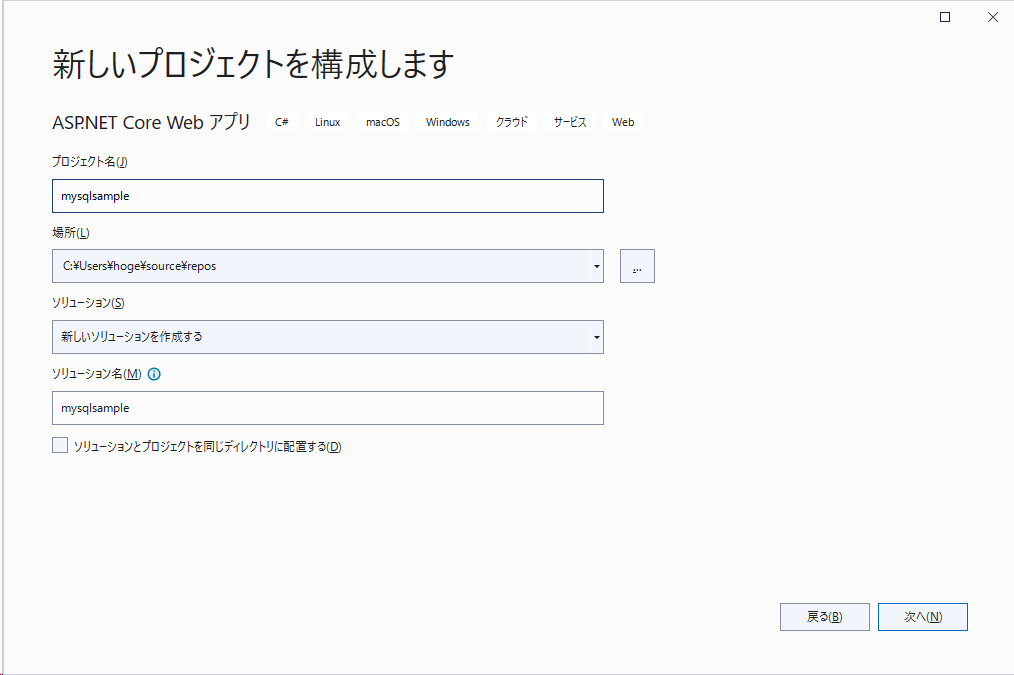
「.NET 6.0」を使用します。
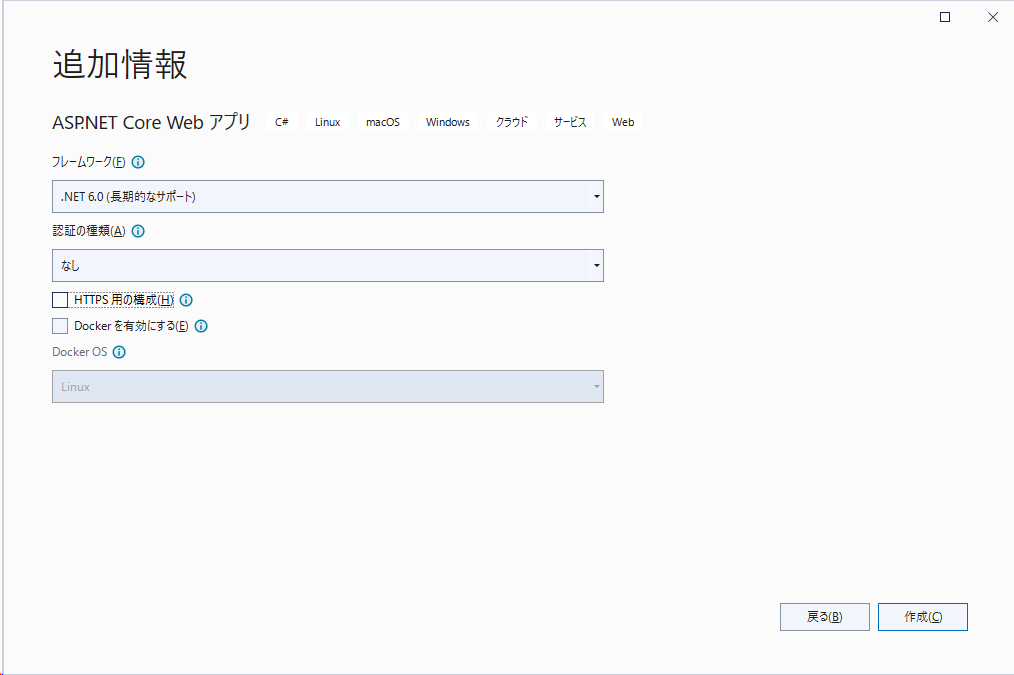
MongoDB.Driverを使用
「MongoDB」を使用するために「MongoDB.Driver」を使用します。
「ツール」 > 「NuGetパッケージマネージャー」>「ソリューションのNuGetパッケージの管理」を選択します。
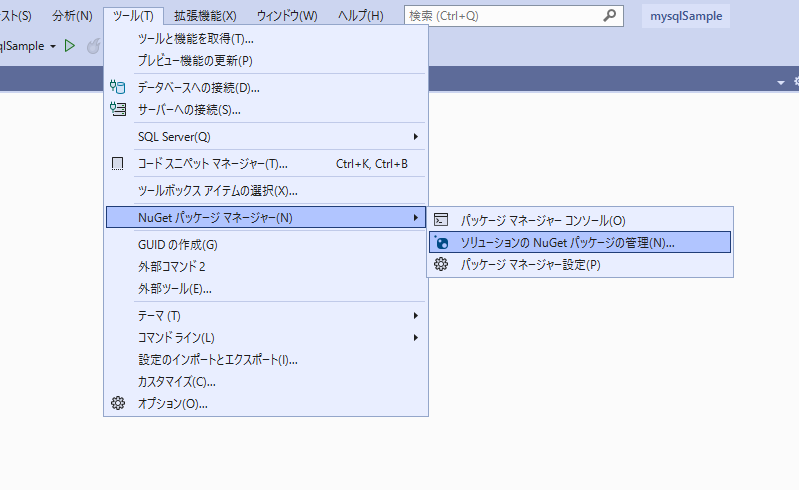
「MongoDB.Driver」で検索します。
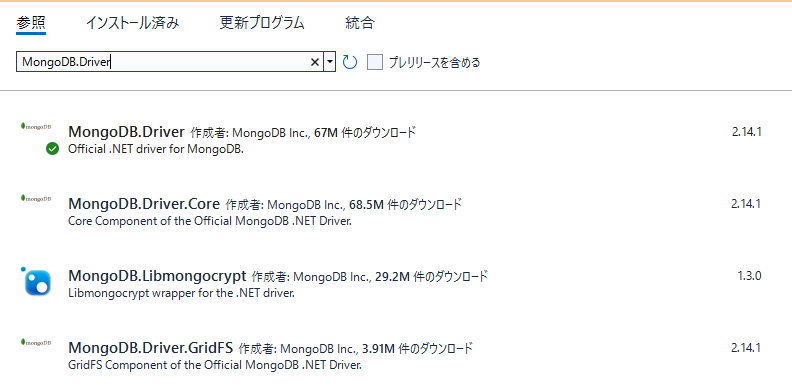
インストールを実行します。
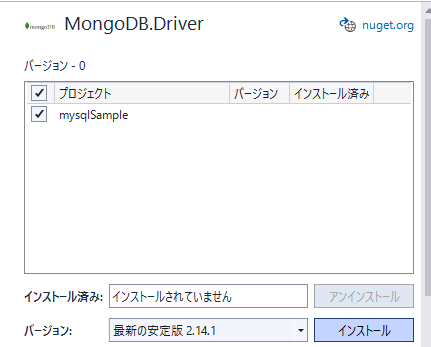
「同意する」をクリックしてインストールを完了します。
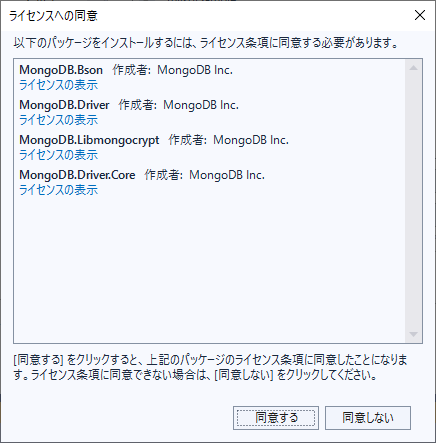
MongoDB
MongoDBは、以下のテーブルを使用します。
> use hoge
switched to db hoge
> db.foo.insert({name:'itiro', age:10, gender:'m'});
WriteResult({ "nInserted" : 1 })
> db.foo.insert({name:'jiro', age:20, gender:'m'});
WriteResult({ "nInserted" : 1 })
> db.foo.insert({name:'saburo', age:30, gender:'m'});
WriteResult({ "nInserted" : 1 })
> db.foo.insert({name:'jiro', age:40, gender:'f'});
WriteResult({ "nInserted" : 1 })
> db.foo.insert({name:'jiro', age:50, gender:'x'});
WriteResult({ "nInserted" : 1 })
> db.foo.find()
{ "_id" : ObjectId("61cabf3205d68674258825be"), "name" : "itiro", "age" : 10, "gender" : "m" }
{ "_id" : ObjectId("61cabf3205d68674258825bf"), "name" : "jiro", "age" : 20, "gender" : "m" }
{ "_id" : ObjectId("61cabf3205d68674258825c0"), "name" : "saburo", "age" : 30, "gender" : "m" }
{ "_id" : ObjectId("61cabf3205d68674258825c1"), "name" : "jiro", "age" : 40, "gender" : "f" }
{ "_id" : ObjectId("61cabf3405d68674258825c2"), "name" : "jiro", "age" : 50, "gender" : "x" }
「foo」コレクション
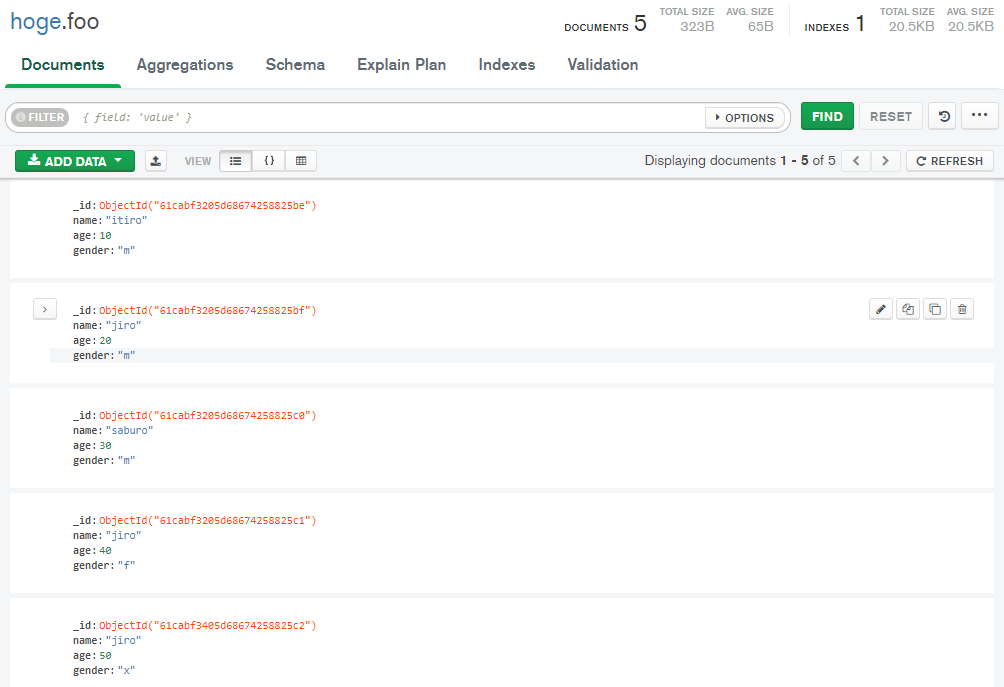
フォルダ構成
最終的なフォルダ構成は以下のようになります。
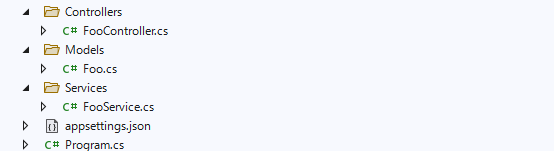
Models作成
「Models」フォルダを作成して配下に「Foo.cs」を作成します。
using MongoDB.Bson;
using MongoDB.Bson.Serialization.Attributes;
namespace mysqlSample.Models
{
public class Foo
{
[BsonId]
[BsonRepresentation(BsonType.ObjectId)]
public string Id { get; set; }
public string name { get; set; }
public decimal age { get; set; }
public string gender { get; set; }
}
}
mongoDB接続情報作成
「appsettings.json」に接続情報を記述します。
localhostに接続してます。
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"FoostoreDatabaseSettings": {
"FooCollectionName": "foo",
"ConnectionString": "mongodb://localhost:27017",
"DatabaseName": "hoge"
},
"AllowedHosts": "*"
}
接続情報を使用するため「Models」配下に「FoostoreDatabaseSettings.cs」を作成します。
namespace mysqlSample.Models
{
public class FoostoreDatabaseSettings: IFoostoreDatabaseSettings
{
public string FooCollectionName { get; set; }
public string ConnectionString { get; set; }
public string DatabaseName { get; set; }
}
public interface IFoostoreDatabaseSettings
{
string FooCollectionName { get; set; }
string ConnectionString { get; set; }
string DatabaseName { get; set; }
}
}
接続情報などの登録
「Program.cs」に接続情報などの登録を記述しておきます。
using Microsoft.EntityFrameworkCore;
using mysqlSample.Models;
using mysqlSample.Services;
using Microsoft.Extensions.Options;
var builder = WebApplication.CreateBuilder(args);
ConfigurationManager configuration = builder.Configuration;
builder.Services.Configure<FoostoreDatabaseSettings>(
configuration.GetSection(nameof(FoostoreDatabaseSettings)));
builder.Services.AddSingleton<IFoostoreDatabaseSettings>(sp =>
sp.GetRequiredService<IOptions<FoostoreDatabaseSettings>>().Value);
builder.Services.AddSingleton<FooService>();
builder.Services.AddControllers();
var app = builder.Build();
// Configure the HTTP request pipeline
app.UseAuthorization();
app.MapControllers();
app.Run();
サービス作成
「Services」フォルダを作成して、「FooService.cs」を作成してサービスを作成します。
using mysqlSample.Models;
using MongoDB.Driver;
using System.Collections.Generic;
using System.Linq;
namespace mysqlSample.Services
{
public class FooService
{
private readonly IMongoCollection<Foo> _foos;
public FooService(IFoostoreDatabaseSettings settings)
{
var client = new MongoClient(settings.ConnectionString);
var database = client.GetDatabase(settings.DatabaseName);
_foos = database.GetCollection<Foo>(settings.FooCollectionName);
}
public List<Foo> Get() =>
_foos.Find(foo => true).ToList();
public Foo Get(string id) =>
_foos.Find<Foo>(foo => foo.Id == id).FirstOrDefault();
public Foo Create(Foo foo)
{
_foos.InsertOne(foo);
return foo;
}
public void Update(string id, Foo fooIn) =>
_foos.ReplaceOne(foo => foo.Id == id, fooIn);
public void Remove(Foo fooIn) =>
_foos.DeleteOne(foo => foo.Id == fooIn.Id);
public void Remove(string id) =>
_foos.DeleteOne(foo => foo.Id == id);
}
}
Controller作成
「Controllers」フォルダを作成して「FooController.cs」を追加します。
using mysqlSample.Models;
using mysqlSample.Services;
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
namespace mysqlSample.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class FooController : ControllerBase
{
private readonly FooService _fooService;
public FooController(FooService fooService)
{
_fooService = fooService;
}
[HttpGet]
public ActionResult<List<Foo>> Get() =>
_fooService.Get();
[HttpGet("{id:length(24)}", Name = "GetFoo")]
public ActionResult<Foo> Get(string id)
{
var foo = _fooService.Get(id);
if (foo == null)
{
return NotFound();
}
return foo;
}
[HttpPost]
public ActionResult<Foo> Create(Foo foo)
{
_fooService.Create(foo);
return CreatedAtRoute("GetFoo", new { id = foo.Id.ToString() }, foo);
}
[HttpPut("{id:length(24)}")]
public IActionResult Update(string id, Foo fooIn)
{
var foo = _fooService.Get(id);
if (foo == null)
{
return NotFound();
}
_fooService.Update(id, fooIn);
return NoContent();
}
[HttpDelete("{id:length(24)}")]
public IActionResult Delete(string id)
{
var foo = _fooService.Get(id);
if (foo == null)
{
return NotFound();
}
_fooService.Remove(foo.Id);
return NoContent();
}
}
}
実行
実行するとAPIが利用できることが確認できます。
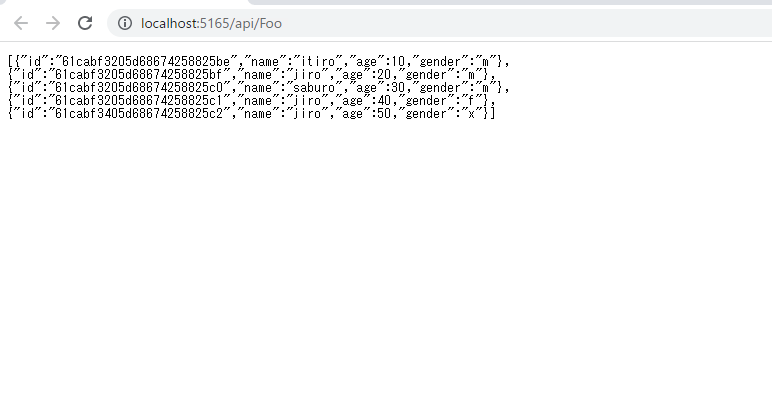
-
前の記事
javascript lodashを使ってパスを指定してオブジェクトから値を取得する 2021.12.30
-
次の記事
Vue.js delete・backspaceキーの入力を取得する 2021.12.30
コメントを書く