go言語 文字列を数値に変換する
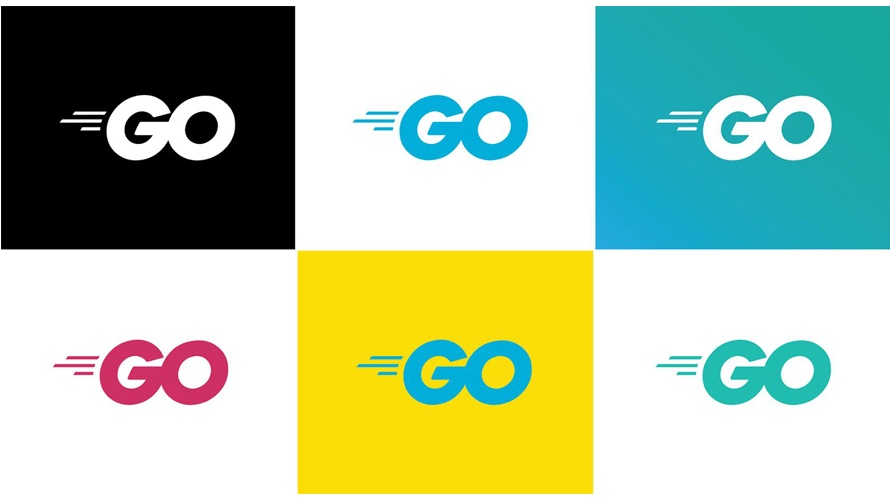
go言語で、strconvパッケージのAtoiを使って、文字列を数値に変換するサンプルコードを記述してます。go言語のバージョンは1.15.4を使用してます。
環境
- OS windows10 pro 64bit
- go言語 1.15.4
Atoi使い方
Atoiを使用すると、整数にみえる文字列を数値に変換することが可能です。
strconv.Atoi(文字列)
以下は、整数の文字列を数値に変換するサンプルコードとなります。
package main
import (
"fmt"
"strconv"
)
func main() {
str1 := "10"
num1, _ := strconv.Atoi(str1)
fmt.Println(num1 + 1)
// 11
str2 := "-10"
num2, _ := strconv.Atoi(str2)
fmt.Println(num2 + 1)
// -9
}
小数点を含む場合は「ParseFloat」を使用します。
package main
import (
"fmt"
"strconv"
)
func main() {
str1 := "10.1"
num1, _ := strconv.ParseFloat(str1, 64)
fmt.Println(num1 + 1)
// 11.1
str2 := "-10.1"
num2, _ := strconv.ParseFloat(str2, 64)
fmt.Println(num2 + 1)
// -9.1
}
-
前の記事
javascript insertAdjacentHTMLを使用してhtml要素を挿入する 2021.04.17
-
次の記事
rails elseifでエラー「NoMethodError」がでる 2021.04.17
コメントを書く