javascript 全角文字は2文字で半角文字は1文字としてカウントする
- 作成日 2020.12.21
- 更新日 2022.07.28
- javascript
- javascript
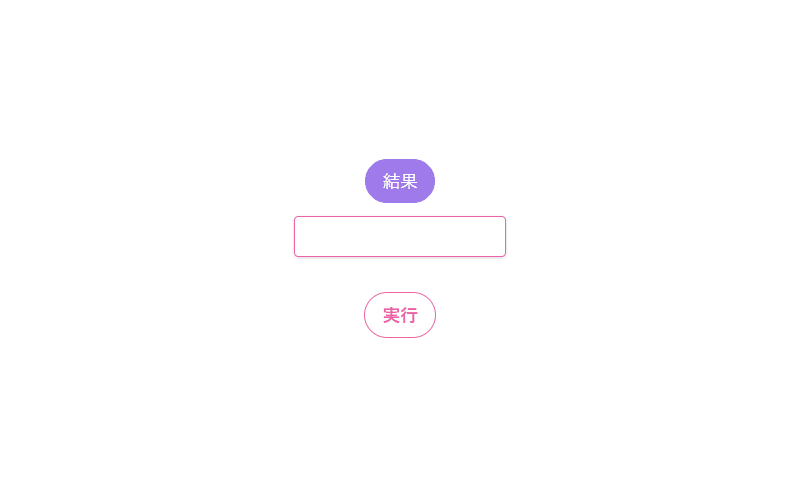
javascriptで、全角文字は2文字で半角文字は1文字としてカウントするサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Apache 2.4.43
- ブラウザ chrome 103.0.5060.134
文字数カウント
「全角文字」は2文字で、「半角文字」は1文字としてカウントするには、正規表現「[ -~]」を使用します。
文字列.match(/[ -~]/)) ? 半角時の処理 : 全角時の処理
正規表現を使用することで、「全角文字」は2文字で「半角文字」は1文字としてカウントすることが
以下のように可能です。関数はアロー関数で記述してます。
function count (str) {
let len = 0;
for (let i = 0; i < str.length; i++) {
(str[i].match(/[ -~]/)) ? len += 1 : len += 2;
}
return len;
}
console.log(count("abcde")); // 5
console.log(count("ABCDE")); // 5
console.log(count("あいうえお")); // 10
console.log(count("aあ")); // 3
console.log(count("AA")); // 3
サロゲートペア文字のような通常の2バイトで1文字で表すところを、4バイトで1文字となるものは、
「4」としてカウントされます。
function count (str) {
let len = 0;
for (let i = 0; i < str.length; i++) {
(str[i].match(/[ -~]/)) ? len += 1 : len += 2;
}
return len;
}
console.log(count("😇")); // 4
console.log(count("😇🙅")); // 8
サンプルコード
以下は、
「実行」ボタンをクリックすると、フォームに入力された文字列を全角文字は2文字で半角文字は1文字としてカウントして表示する
サンプルコードとなります。
※cssには「tailwind」を使用してます。関数はアロー関数で記述してます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>mebeeサンプル</title>
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
</head>
<script>
const count = (str) => {
let len = 0;
for (let i = 0; i < str.length; i++) {
(str[i].match(/[ -~]/)) ? len += 1 : len += 2;
}
return len;
}
const hoge = () => {
result.innerHTML = count(str.value);
}
window.onload = () => {
// クリックイベントを登録
btn.onclick = () => { hoge() }; // document.getElementById('btn');を省略
}
</script>
<body>
<div class="container mx-auto my-56 w-56 px-4">
<div class="flex justify-center">
<p id="result" class="bg-purple-500 text-white py-2 px-4 rounded-full mb-3 mt-4">結果</p>
</div>
<div class="flex justify-center">
<input id="str" type="text"
class="shadow appearance-none border border-pink-500 rounded w-full py-2 px-3 text-gray-700 mb-3 leading-tight focus:outline-none focus:shadow-outline">
</div>
<div class="flex justify-center">
<button id="btn" type="button"
class="mt-5 bg-transparent border border-pink-500 hover:border-pink-300 text-pink-500 hover:text-pink-300 font-bold py-2 px-4 rounded-full">
実行
</button>
</div>
</div>
</body>
</html>
文字数がカウントされていることが確認できます。
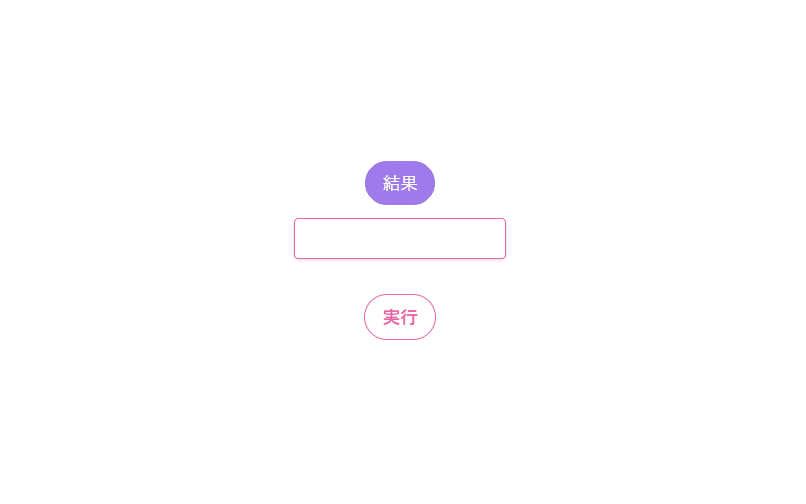
また、for文は以下のように1行で記述することも可能です。
[...Array(str.length)].forEach((v, i) => (str[i].match(/[ -~]/)) ? len += 1 : len += 2)
windowオブジェクトも省略して記述することが可能です。
onload = () => {
btn.onclick = () => { hoge(); };
}
-
前の記事
Deno ファイルを読み込んで内容を出力する 2020.12.21
-
次の記事
node.js performance.now使用時に「ReferenceError: performance is not defined」が発生した場合の対処 2020.12.22
コメントを書く