C# List(リスト)の値をソートする
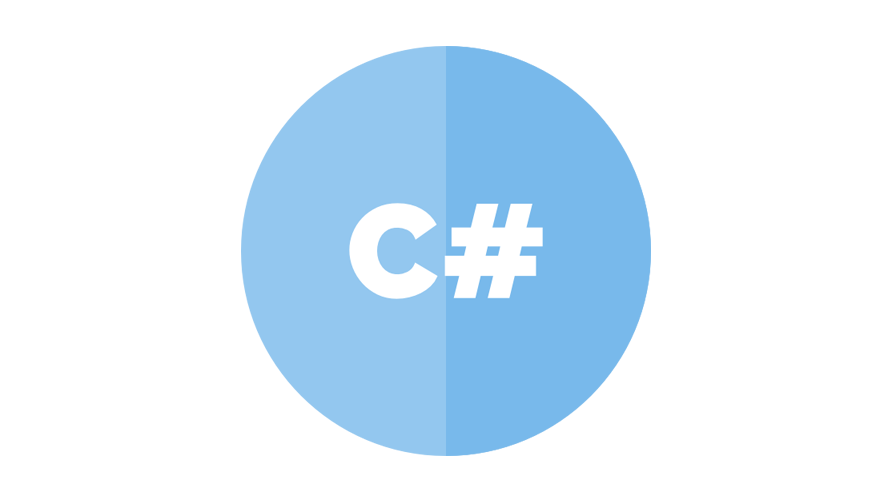
C#で、Sortを使用して、List(リスト)の値をソートするサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2019 Version 16.7.1
Sort使い方
Sortを使用すると、List(リスト)の値をソートすることが可能です。
ここでは、生成したlistとに「5, 1, 3, 4, 6, 2, 7」を追加して、これらを昇順で並び替えてます。
using System;
using System.Collections.Generic;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
var list = new List<int> { 5, 1, 3, 4, 6, 2, 7 };
list.Sort();
// 結果
foreach (int i in list)
{
Console.Write("{0} ", i); // 1 2 3 4 5 6 7
}
Console.ReadKey();
}
}
}
ちなみに、結果の表示は「foreach」ではなく
「Linq」または「ラムダ」を使用して表示することも可能です。
using System;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
var list = new List<int> { 5, 1, 3, 4, 6, 2, 7 };
list.Sort();
// 結果 Linq
Console.WriteLine(String.Join(" ", from i in list select i));
// 1 2 3 4 5 6 7
// 結果 ラムダ演算子
Console.WriteLine(String.Join(" ", list.Select(i => i)));
// 1 2 3 4 5 6 7
Console.ReadKey();
}
}
}
降順にする場合は、ラムダ式を使用します。
using System;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
var list = new List<int> { 5, 1, 3, 4, 6, 2, 7 };
list.Sort((x, y) => y - x);
// 結果
Console.WriteLine(String.Join(" ", list.Select(i => i)));
// 7 6 5 4 3 2 1
Console.ReadKey();
}
}
}
-
前の記事
python 時刻を分から時:分で表示する 2020.12.20
-
次の記事
Ruby 配列内の値を総和を求める 2020.12.21
コメントを書く