java 指定した範囲内に正規表現にマッチする文字列が含まれているかを判定する
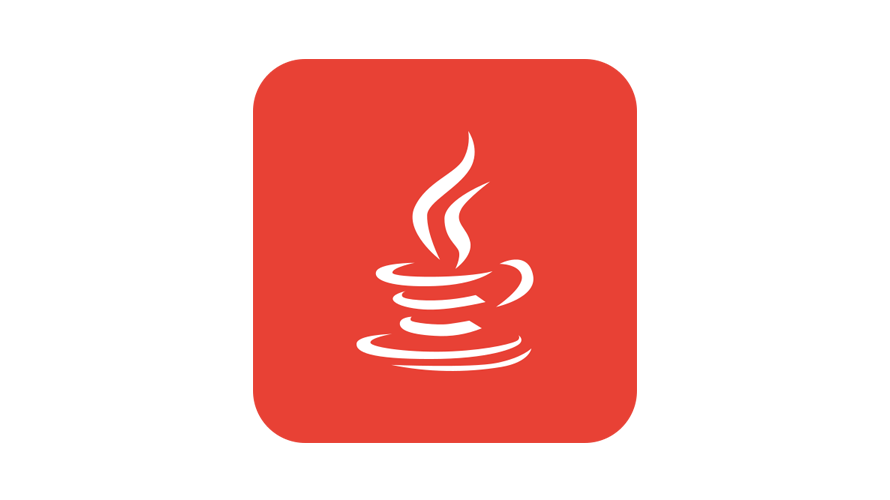
javaで、指定した範囲内に正規表現にマッチする文字列が含まれているかを判定する手順を記述してます。「Pattern.compile」と「region」で可能です。
環境
- OS windows11 home
- java 19.0.1
手順
指定した範囲内に正規表現にマッチする文字列が含まれているかを判定するには、「Pattern.compile」と「region」を使用します。
Pattern p = Pattern.compile("文字列");
Matcher m1 = p.matcher("文字列");
m1.region(開始位置 0から,終了位置 最終位置のより1プラス);
m1.find(); // 判定
実際に、使用してみます。
import java.util.regex.*;
public class App {
public static void main(String[] args) throws Exception {
String reg = "[0-9]{3}"; // 3文字の半角英数字
Pattern p = Pattern.compile(reg);
Matcher m1 = p.matcher("abcde12345");
m1.region(6, 10);
System.out.println(m1.find()); // true
Matcher m2 = p.matcher("abcde12345");
m2.region(0, 4);
System.out.println(m1.find()); // false
Matcher m3 = p.matcher("abcde12345");
m3.region(6, 9);
System.out.println(m1.find()); // false
}
}
判定されていることが確認できます。
範囲を超える
範囲を超えた場合は「IndexOutOfBoundsException」が発生します。
import java.util.regex.*;
public class App {
public static void main(String[] args) throws Exception {
String reg = "[0-9]{3}"; // 3文字の半角英数字
Pattern p = Pattern.compile(reg);
Matcher m1 = p.matcher("abcde12345");
m1.region(6, 11);
// Exception in thread "main" java.lang.IndexOutOfBoundsException: end
// at java.base/java.util.regex.Matcher.region(Matcher.java:1514)
// at App.main(App.java:10)
}
}
-
前の記事
Oracle Database インデックス名を変更する 2023.03.04
-
次の記事
GAS googleドライブの指定したidのファイルを取得する 2023.03.05
コメントを書く