javascript エラー「Uncaught TypeError: xxx.animate is not a function」の解決方法
- 作成日 2022.12.09
- javascript
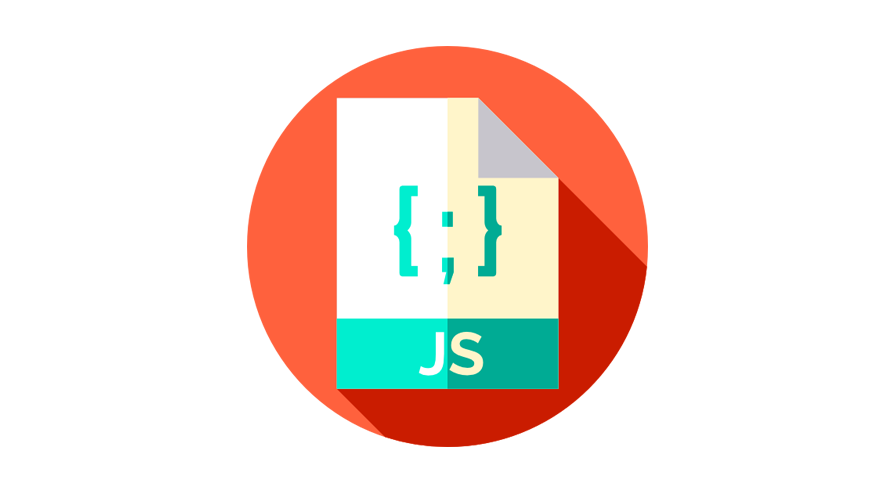
javascriptで、エラー「Uncaught TypeError: xxx.animate is not a function」が発生した場合の原因と解決方法を記述してます。「NodeList」に、そのまま「animate」を使用した場合に発生します。「chrome」や「firefox」や「safari」の各ブラウザのエラーメッセージの画像もキャプチャしてます。
環境
- OS windows11 pro 64bit
- ブラウザ chrome 107.0.5304.122
エラー内容
以下の、「animate」をクラスに使用とした際に発生。
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<script>
const elm = document.querySelectorAll('.box');
elm.animate(
// 変化させるスタイル
[
{ transform: 'scale(1, 5)' },
{ transform: 'scale(5, 1)' }
],
// プロパティ
{
duration: 500,
iterations: Infinity,
}
);
</script>
エラーメッセージ
Uncaught TypeError: elm.animate is not a function
画像

firefox107の場合では、以下のエラーが発生します。
Uncaught TypeError: elm.animate is not a function
画像

safari15.5では、以下のエラーとなります。
TypeError: undefined is not a function (near '...elm.animate...')
画像

原因
「NodeList」に対して、「animate」を使用しているため。
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<script>
const elm = document.querySelectorAll('.box');
console.log(elm);
// NodeList(3) [div.box, div.box, div.box]
</script>
解決方法
いずれかの要素を指定して実行する場合は「インデックス番号」を指定して「DOM要素」にします。
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<script>
const elm = document.querySelectorAll('.box');
elm[0].animate(
// 変化させるスタイル
[
{ transform: 'scale(1, 5)' },
{ transform: 'scale(5, 1)' }
],
// プロパティ
{
duration: 500,
iterations: Infinity,
}
);
</script>
取得した全ての要素に使用する場合は「forEach」などを使用します。
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<script>
const elm = document.querySelectorAll('.box');
elm.forEach(v => {
v.animate(
// 変化させるスタイル
[
{ transform: 'scale(1, 5)' },
{ transform: 'scale(5, 1)' }
],
// プロパティ
{
duration: 500,
iterations: Infinity,
}
)
});
</script>
または、判定してから使用します。
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<script>
const elm = document.querySelectorAll('.box');
if (typeof elm === 'object' && 'querySelectorAll' in elm && elm !== null) {
elm.animate(
// 変化させるスタイル
[
{ transform: 'scale(1, 5)' },
{ transform: 'scale(5, 1)' }
],
// プロパティ
{
duration: 500,
iterations: Infinity,
}
);
};
</script>
-
前の記事
MariaDB insert実行後のオートインクリメント値を取得する 2022.12.08
-
次の記事
Redis 最小値と最大値を指定してスコアのメンバーを取得する 2022.12.09
コメントを書く