node.js テキストを暗号化する
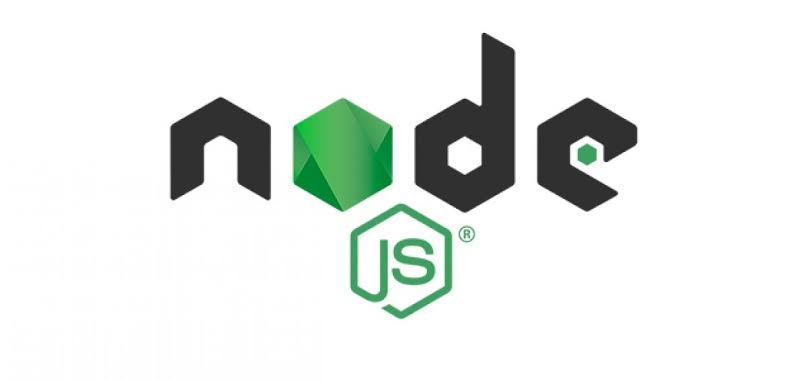
node.jsで、テキストを暗号化するサンプルコードを記述してます。
環境
- OS windows 10 pro
- node.js 14.6.0
暗号化
テキストを暗号化するには、「crypto」と「cipher」を使用します。
var crypto = require("crypto");
サンプルコード
「hello world」をパスワード「pass」で暗号化してみます。
サンプルコードは、以下となります。
※ここでは「test.js」という名前でファイルを作成してます。
const crypto = require('crypto')
const cipher = crypto.createCipher('aes-256-cbc', "pass")
const crypted = cipher.update("hello world", 'utf-8', 'hex')
const text = crypted + cipher.final('hex')
console.log(text)
実行結果を見ると、暗号化されていることが確認できます。
>node test.js
b18951add08a01a79e2854db8a072095
(node:15280) [DEP0106] DeprecationWarning: crypto.createCipher is deprecated.
(Use `node --trace-deprecation ...` to show where the warning was created)
復号化
復号化することも可能です。
さきほど暗号化したものを復号化してみます。
var crypto = require("crypto");
const decipher = crypto.createDecipher('aes-256-cbc', "pass")
const decrypted = decipher.update("b18951add08a01a79e2854db8a072095", 'hex', 'utf-8')
const text = decrypted + decipher.final('utf-8')
console.log(text)
実行結果を確認すると復号化されていることが確認できます。
>node test.js
hello world
(node:16976) [DEP0106] DeprecationWarning: crypto.createDecipher is deprecated.
(Use `node --trace-deprecation ...` to show where the warning was created)
暗号化できる種類やハッシュの種類は以下で確認可能です。
var crypto = require("crypto");
var cipers = crypto.getCiphers();
console.log(cipers);
var hashes = crypto.getHashes();
console.log(hashes);
-
前の記事
PostgreSQL nullだった場合は置換する 2022.02.28
-
次の記事
javascript lodashを使って配列内のオブジェクトのプロパティに条件を指定して抽出する 2022.03.01
コメントを書く