python PySimpleGUIでTabの選択時の背景色とテキストの色を設定する
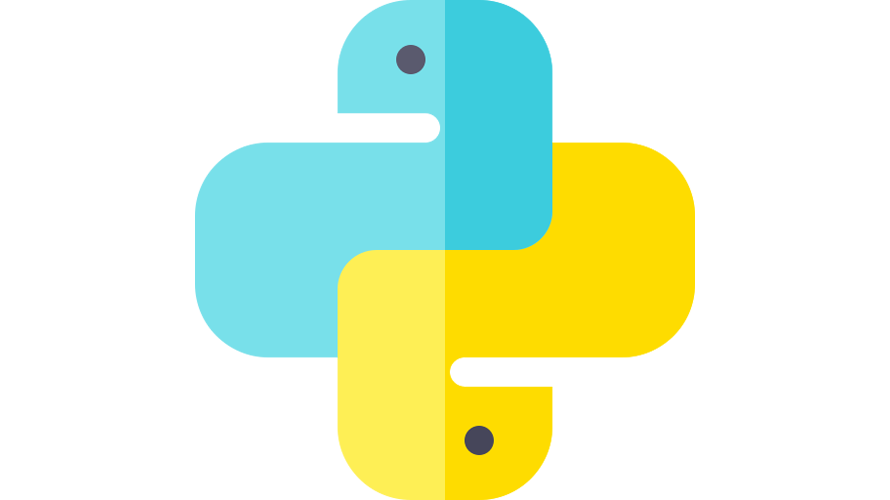
pythonで、ライブラリPySimpleGUIを使用して、Tabの選択時の背景色とテキストの色を設定するサンプルコードを記述してます。pythonのバージョンは3.8.5を使用してます。
環境
- OS windows10 pro 64bit
- python 3.8.5
PySimpleGUIインストール
PySimpleGUIをインストールされていない方は、pipでインストールしておきます。
PySimpleGUI
<出力結果>
Collecting PySimpleGUI
Downloading PySimpleGUI-4.41.2-py3-none-any.whl (348 kB)
|████████████████████████████████| 348 kB 1.3 MB/s
Installing collected packages: PySimpleGUI
Successfully installed PySimpleGUI-4.41.2
選択時の背景色とテキストの色を設定
選択時の背景色とテキストの色を設定するには、「selected_background_color」に背景色にしたいカラーコードを指定して、「selected_title_color」にテキストの色にしたいカラーコードを指定します。
import PySimpleGUI as sg
t1 = sg.Tab('backpack' ,[[sg.Text('tab1')],[sg.Button('button1')]])
t2 = sg.Tab('alarm-clock' ,[[sg.Input(key='input')]])
t3 = sg.Tab('pencil-case.png' ,[[sg.Image(filename='pencil-case.png')]])
layout=[
[sg.TabGroup ([[t1 ,t2, t3 ]], selected_background_color='#FBDE08', selected_title_color='#ff0000')]
]
以下は、tab選択時の背景色とテキストの色を指定して表示するサンプルコードとなります。
import PySimpleGUI as sg
# ウィンドウのテーマ
sg.theme('SandyBeach')
# Tab( タイトル, レイアウト)
t1 = sg.Tab('text-tab' ,[[sg.Text('tab1')],[sg.Button('button1')]])
t2 = sg.Tab('input-tab' ,[[sg.Input(key='input')]])
t3 = sg.Tab('image-tab' ,[[sg.Image(filename='pencil-case.png')]])
# ウィンドウのレイアウト
layout=[
[sg.TabGroup ([[t1 ,t2, t3 ]], selected_background_color='#FBDE08', selected_title_color='#ff0000')]
]
# ウィンドウオブジェクトの作成
window = sg.Window('title', layout, size=(500, 500))
# イベントのループ
while True:
# イベントの読み込み
event, values = window.read()
# ウィンドウの×ボタンクリックで終了
if event == sg.WIN_CLOSED:
break
# ウィンドウ終了処理
window.close()
実行結果
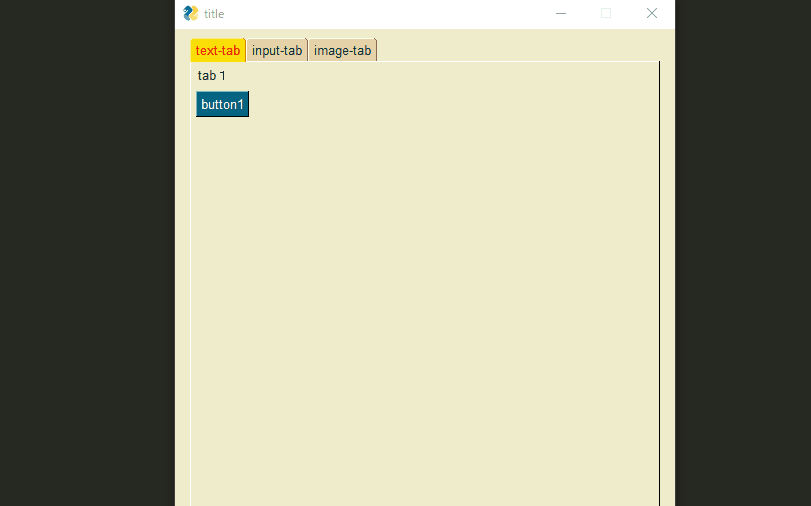
-
前の記事
javascript ${}(テンプレートリテラル)をカスタマイズする 2021.05.22
-
次の記事
go言語 スライス(配列)の和を計算する 2021.05.22
コメントを書く