java 配列同士が同じであるかを判定する
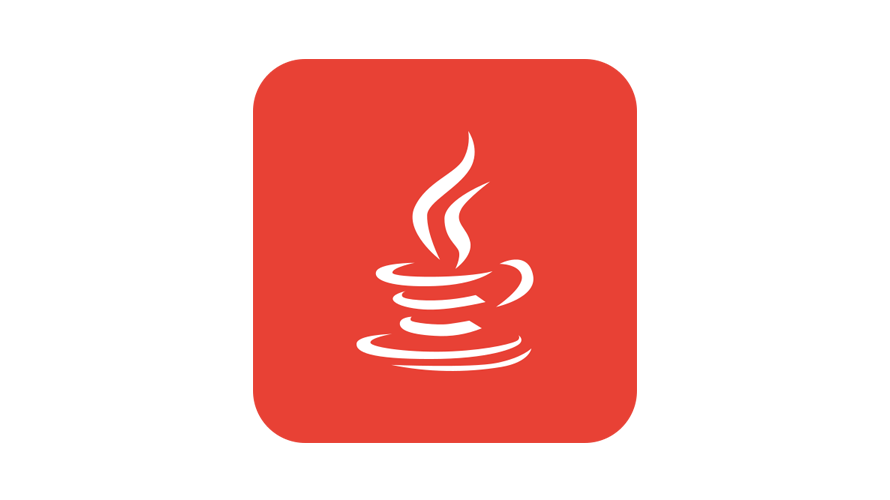
javaで、配列同士が同じであるかを判定する手順を記述してます。「Arrays.equals」に判定したい配列を指定することで可能です。「==」では判定できません。
環境
- OS windows11 home
- java 19.0.1
手順
配列同士が同じであるかを判定するには、「Arrays.equals」を使用します。
Arrays.equals( 配列 , 配列 )
実際に、使用してみます。
import java.util.Arrays;
public class App {
public static void main(String[] args) throws Exception {
int[] a = {1, 2, 3, 4, 5};
int[] b = {1, 2, 3, 4, 5};
int[] c = {1, 2, 3};
int[] d = a;
System.out.println(Arrays.equals(a, b)); // true
System.out.println(Arrays.equals(a, c)); // false
System.out.println(Arrays.equals(a, d)); // true
}
}
比較されていることが確認できます。
「==」で比較
ちなみに「==」で比較すると正しい結果は得られません。
import java.util.Arrays;
public class App {
public static void main(String[] args) throws Exception {
int[] a = {1, 2, 3, 4, 5};
int[] b = {1, 2, 3, 4, 5};
int[] c = {1, 2, 3};
int[] d = a;
System.out.println(a == b); // false
System.out.println(a == c); // false
System.out.println(a == d); // true
}
}
多次元配列を比較
多次元配列を比較する場合は「equals」ではできず、「deepEquals」を使用します。
import java.util.Arrays;
public class App {
public static void main(String[] args) throws Exception {
int[][] a = {{1, 2}, {3, 4}};
int[][] b = {{1, 2}, {3, 4}};
int[][] c = {{1, 2, 3}, {4}};
int[][] d = a;
System.out.println(Arrays.equals(a, b)); // false
System.out.println(Arrays.equals(a, c)); // false
System.out.println(Arrays.equals(a, d)); // true
System.out.println(Arrays.deepEquals(a, b)); // true
System.out.println(Arrays.deepEquals(a, c)); // false
System.out.println(Arrays.deepEquals(a, d)); // true
}
}
-
前の記事
Thunderbird タブを閉じるショートカットキー 2023.12.26
-
次の記事
Oracle Database ログインしているユーザーを確認する 2023.12.28
コメントを書く