C# ランダムな文字列生成処理で「for」と「Linq」のパフォーマンスを計測して比較する
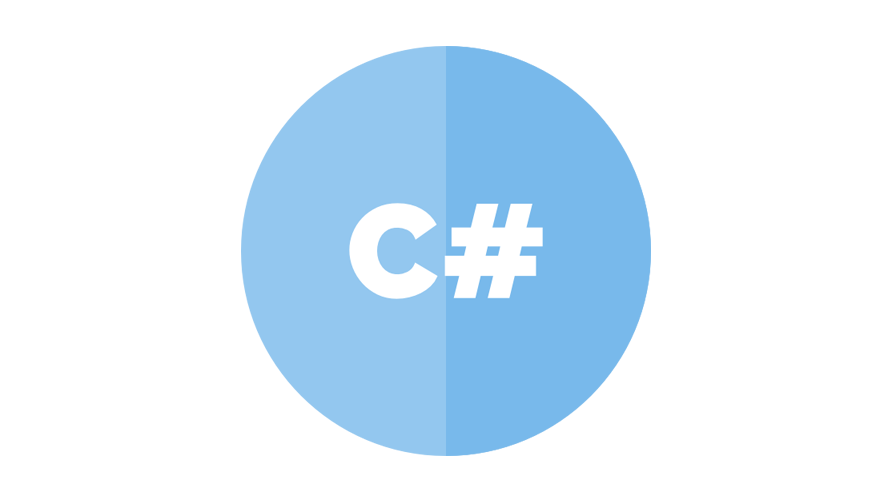
C#で、ランダムな文字列生成処理を「for」と「Linq」のそれぞれで実行したパフォーマンスを計測して比較するコードと結果を記述してます。結果は「Linq」を使用したほうが良さそうです。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.2.3
パフォーマンス計測
「System.Diagnostics.Stopwatch」を使用して、ランダムな文字列生成処理で「for」と「Linq」を100万回実行して、計測した結果を比較してみます。
using System;
using System.Linq;
namespace ConsoleApp1
{
internal class Program
{
private const string txt = "123456789";
private const int n = 1_000_000;
static void Main(string[] args)
{
var txt = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
var m = 100; // 100文字生成
var random = new Random(); // 乱数発生用
var time = new System.Diagnostics.Stopwatch();
string a, b;
// 計測開始
time.Start();
for (int i = 0; i < n; i++)
{
a = "";
for (int j = 0; j < m; j++)
{
a += txt[random.Next(txt.Length)]; // 文字数の範囲内で乱数を生成
}
}
time.Stop();
System.Diagnostics.Debug.WriteLine($"for : {time.ElapsedMilliseconds}ms");
// 計測開始
time.Reset(); // リセット
time.Start();
for (int i = 0; i < n; i++)
{
b = new string(Enumerable.Repeat(txt, m)
.Select(x => x[random.Next(x.Length)]).ToArray());
}
time.Stop();
System.Diagnostics.Debug.WriteLine($"Linq : {time.ElapsedMilliseconds}ms");
}
}
}
実行結果をみると「Linq」を使用した方が、速そうです。
【1回目】
for : 5904ms
Linq : 2793ms
【2回目】
for : 5883ms
Linq : 2780ms
【3回目】
for : 5867ms
Linq : 2783ms
-
前の記事
GAS googleドライブの使用量を確認する 2023.06.13
-
次の記事
MariaDB 時間を全て秒で取得する 2023.06.14
コメントを書く