C# 空白除去処理で「Regex.Replace」と「Linq(Concat・Char.IsWhiteSpace)」のパフォーマンスを計測して比較する
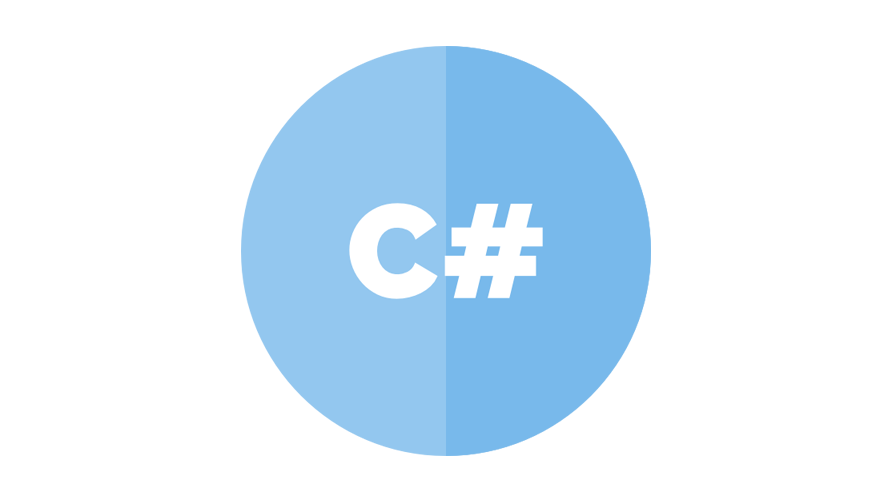
C#で、空白除去処理を「Regex.Replace」と「Linq(Concat・Char.IsWhiteSpace)」のそれぞれで100万回実行したパフォーマンスを計測して比較するコードと結果を記述してます。結果はわずかですが「Linq(Concat・Char.IsWhiteSpace)」を使用した方が良さそうです。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.2.3
パフォーマンス計測
「System.Diagnostics.Stopwatch」を使用して、同じ空白除去処理で「Regex.Replace」と「Linq(Concat・Char.IsWhiteSpace)」を100万回実行して、計測した結果を比較してみます。
using System;
using System.Text.RegularExpressions;
using System.Linq;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
var time = new System.Diagnostics.Stopwatch();
int n = 1_000_000;
string str = " he ll o w o r l d";
string result;
TimeSpan ts;
// 計測開始
time.Start();
for (int i = 0; i < n; i++)
{
result = Regex.Replace(str, @"\s", "");
}
time.Stop();
ts = time.Elapsed;
System.Diagnostics.Debug.WriteLine($"Regex.Replace : {time.ElapsedMilliseconds}ms");
// 計測開始
time.Start();
for (int i = 0; i < n; i++)
{
result = String.Concat(str.Where(x => !Char.IsWhiteSpace(x)));
}
time.Stop();
ts = time.Elapsed;
System.Diagnostics.Debug.WriteLine($"Linq(Concat:Char.IsWhiteSpace) : {time.ElapsedMilliseconds}ms");
}
}
}
実行結果をみると「Linq(Concat:Char.IsWhiteSpace)」の方がパフォーマンスは良さそうです。
【1回目】
Regex.Replace : 2769ms
Linq(Concat:Char.IsWhiteSpace) : 459ms
【2回目】
Regex.Replace : 2749ms
Linq(Concat:Char.IsWhiteSpace) : 551ms
【3回目】
Regex.Replace : 2768ms
Linq(Concat:Char.IsWhiteSpace) : 435ms
-
前の記事
java 文字列が空文字であるかを判定する 2023.01.05
-
次の記事
PostgreSQL sin値を取得する 2023.01.05
コメントを書く