C# 先頭の文字のみ大文字に変換する処理で「ToUpper+Substring」と「Regex.Replace」と「ToUpper+ToCharArray」のパフォーマンスを計測して比較する
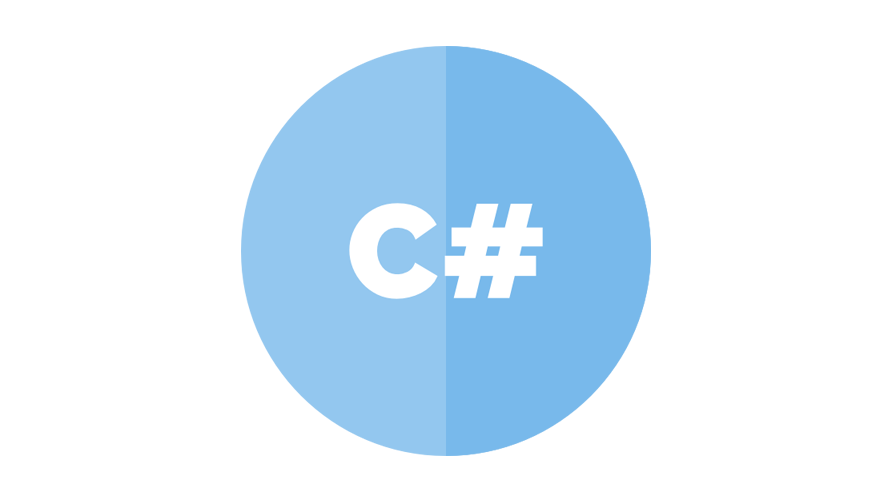
C#で、先頭の文字のみ大文字に変換する処理を「ToUpper+Substring」と「Regex.Replace」と「ToUpper+ToCharArray」のそれぞれで実行したパフォーマンスを計測して比較するコードと結果を記述してます。
環境
- OS windows11 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.2.3
パフォーマンス計測
「System.Diagnostics.Stopwatch」を使用して、先頭の文字のみ大文字に変換する処理で「ToUpper+Substring」と「Regex.Replace」と「ToUpper+ToCharArray」を100万回実行して、計測した結果を比較してみます。
using System;
using System.Text.RegularExpressions;
using System.Linq;
namespace ConsoleApp1
{
internal class Program
{
private const int n = 1_000_000;
static void Main(string[] args)
{
string txt = "mebee";
string result;
var time = new System.Diagnostics.Stopwatch();
// 計測開始
time.Start();
for (int i = 0; i < n; i++)
{
result = char.ToUpper(txt[0]) + txt.Substring(1);
}
time.Stop();
System.Diagnostics.Debug.WriteLine($"ToUpper+Substring : {time.ElapsedMilliseconds}ms");
// 計測開始
time.Reset(); // リセット
time.Start();
for (int i = 0; i < n; i++)
{
result = Regex.Replace(txt, "^[a-za-z]", x => x.Value.ToUpper());
}
time.Stop();
System.Diagnostics.Debug.WriteLine($"Regex.Replace : {time.ElapsedMilliseconds}ms");
// 計測開始
time.Reset(); // リセット
time.Start();
for (int i = 0; i < n; i++)
{
char[] ch = txt.ToCharArray();
ch[0] = char.ToUpper(ch[0]);
result = new string(ch);
}
time.Stop();
System.Diagnostics.Debug.WriteLine($"ToCharArray+ToUpper : {time.ElapsedMilliseconds}ms");
}
}
}
実行結果をみると「Equals」を使用した方が、速そうです。
【1回目】
ToUpper+Substring : 60ms
Regex.Replace : 561ms
ToCharArray+ToUpper : 43ms
【2回目】
ToUpper+Substring : 60ms
Regex.Replace : 554ms
ToCharArray+ToUpper : 44ms
【3回目】
ToUpper+Substring : 60ms
Regex.Replace : 567ms
ToCharArray+ToUpper : 44ms
-
前の記事
CSS 「プロパティ値が無効」ですが発生した場合の対処法 2022.08.10
-
次の記事
javascript 時刻を午前(AM)・午後(PM)形式で表示する 2022.08.10
コメントを書く