C# bindingSourceでDataSetの値を操作する
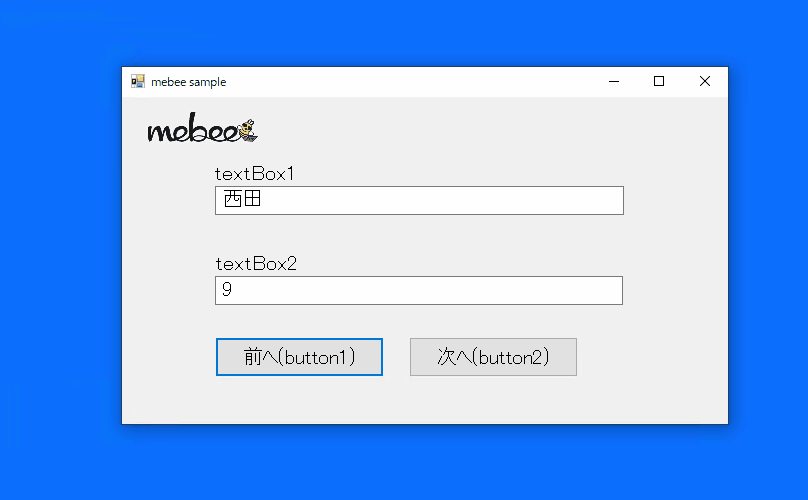
C#で、bindingSourceを使用して、DataSetの値を操作するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2019 Version 16.7.1
bindingSource使い方
bindingSourceを使用すると、DataSetの値を操作することが可能です。
// datasetを作成
DataSet ds = new DataSet();
DataTable dt = ds.Tables.Add("parson");
// カラムを追加
dt.Columns.Add("name");
dt.Columns.Add("age");
// 行を追加
dt.Rows.Add(new string[] { "西田", "9" });
dt.Rows.Add(new string[] { "佐賀", "33" });
dt.Rows.Add(new string[] { "徳川", "50" });
// DataSourceを設定
bindingSource1.DataSource = ds;
bindingSource1.DataMember = "parson";
サンプルコード
以下は、
bindingSourceにDataSetを設定して、「前へ」ボタンをクリックすると1つ前の値へ、「次へ」ボタンをクリックすると次のデータを、textboxに出力する
サンプルコードとなります。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
namespace FormTestApp
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
textBox1.Multiline = true;
textBox1.Height = 70;
textBox1.Text = "hello" + Environment.NewLine
+ "hello" + Environment.NewLine
+ "hello" + Environment.NewLine;
}
private void button1_Click(object sender, EventArgs e)
{
// 改行を分割文字列に指定
string[] arr = textBox1.Text.Split(new[] { Environment.NewLine }, StringSplitOptions.None);
//
foreach (string item in arr)
{
listBox1.Items.Add(item);
}
}
}
}
listboxに改行ごとに分割されて表示されることが確認できます。
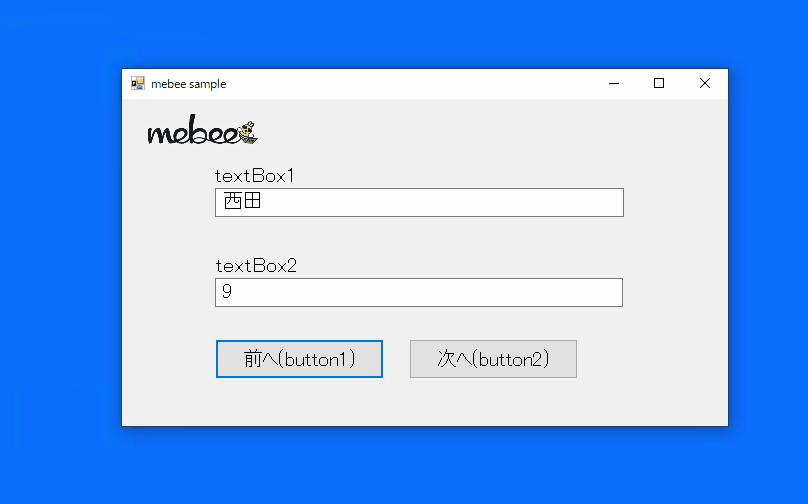
-
前の記事
php 配列をソートする 2020.12.13
-
次の記事
php array_reverseで配列の値を逆順にする 2020.12.13
コメントを書く