C# 文字列を数値に変換する
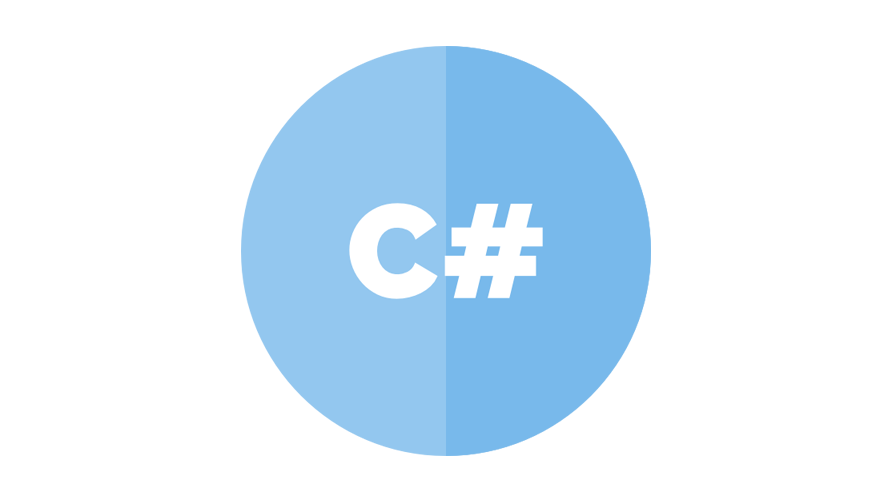
C#で、Parseを使用して、文字列を数値に変換するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2019 Version 16.7.1
数値に変換
各数値の型を指定して、文字列を数字に変換することが可能です。
using System;
using System.Collections.Generic;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
//文字列をint型に変換する
int i = int.Parse("10");
Console.WriteLine(i+1);
// 11
//文字列をlong型に変換する
long l = long.Parse("10");
Console.WriteLine(l + 1);
// 11
//文字列をfloat型に変換する
float f = float.Parse("10.1");
Console.WriteLine(f + 1);
// 11.1
//文字列をdouble型に変換する
double d = double.Parse("10.1");
Console.WriteLine(d + 1);
// 11.1
Console.ReadKey();
}
}
}
Convertクラスを使用することも可能です。
using System;
using System.Collections.Generic;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
int i = Convert.ToInt32("10");
long l = Convert.ToInt64("10");
Console.WriteLine(i + 1);
// 11
Console.WriteLine(l + 1);
// 11
Console.ReadKey();
}
}
}
-
前の記事
php array_filterで配列から空の値を除く 2021.01.10
-
次の記事
javascript nextSiblingで次にあるノードを取得する 2021.01.11
コメントを書く