C# 文字列から指定した文字の数をカウントする
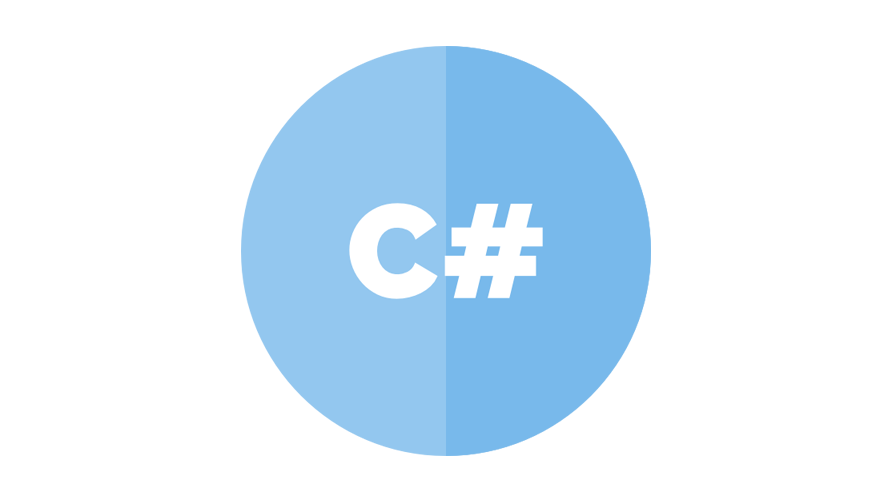
C#で、文字列から指定した文字の数をカウントするサンプルコードを記述してます。「foreach」などでループさせてカウントします。
環境
- OS windows11 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.2.6
文字列から指定した文字の数をカウント
文字列から指定した文字の数をカウントには、以下のように「foreach」を使用してループ処理することでカウントできます。
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string str = "mebee";
int count = 0;
foreach (char c in str)
{
if (c == 'e')
{
count++;
}
}
Console.WriteLine(count);
}
}
}
実行結果

「Linq」
「Linq」で「count」を使用しても、カウントすることが可能です。
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string str = "mebee";
int count = str.Count(v => v == 'e');
Console.WriteLine(count);
}
}
}
「where」を使用してもカウントできます。
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string str = "mebee";
int count = str.Where(x => (x == 'e')).Count();
Console.WriteLine(count);
}
}
}
「Replace」
「Replace」で置換した数から、カウントすることもできます。
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string str = "mebee";
int count = str.Length - str.Replace("e".ToString(), "").Length;
Console.WriteLine(count);
}
}
}
「Split」
分割した結果から「-1」することで、カウントすることもできます。
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string str = "mebee";
int count = str.Split('e').Length - 1;
Console.WriteLine(count);
}
}
}
「Regex」
「Regex」を使用しても可能です。
using System;
using System.Text.RegularExpressions;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string str = "mebee";
int count = Regex.Matches(str, 'e'.ToString()).Count;
Console.WriteLine(count);
}
}
}
※パフォーマンスは「foreach」が良さそうです。
-
前の記事
ruby 文字列を1文字で区切りインデックス番号とともに二次元配列化する 2023.02.17
-
次の記事
GAS googleドライブ内のファイルの説明を取得する 2023.02.17
コメントを書く